Question
Complete the method so that the program can determine whether a string that contains (, ), [, ], {, and } are properly nested. Note
Complete the method so that the program can determine whether a string that contains (, ), [, ], {, and } are properly nested. Note that ( and ) match each other as a pair, [ and ] are a pair, and { and } are a pair. All other characters should be ignored.
For example:
{[()]()} is nested properly: true {a+[b*(10+10)]*(c-d)} is nested properly: true ([()]{} is nested properly: false (a+[b*(e-f)]-{a+b} is nested properly: false [{}]()]() is nested properly: false [{)]() is nested properly: false [{})() is nested properly: false
public class ParenthesesBracketsMatch { public static boolean AreParenthesesBracketsNested(String str) { // Create a stack java.util.Stack public static void main(String[] args) { //DO NOT CHANGE THE MAIN METHOD String str1 = "{[()]()}"; boolean matched1 = AreParenthesesBracketsNested(str1); System.out.println(str1 + " is nested properly: " + matched1); String str1b = "{a+[b*(10+10)]*(c-d)}"; boolean matched1b = AreParenthesesBracketsNested(str1b); System.out.println(str1b + " is nested properly: " + matched1b); String str2 = "([()]{}"; boolean matched2 = AreParenthesesBracketsNested(str2); System.out.println(str2 + " is nested properly: " + matched2); String str2b = "(a+[b*(e-f)]-{a+b}"; boolean matched2b = AreParenthesesBracketsNested(str2b); System.out.println(str2b + " is nested properly: " + matched2b); String str3 = "[{}]()]()"; boolean matched3 = AreParenthesesBracketsNested(str3); System.out.println(str3 + " is nested properly: " + matched3); String str4 = "[{)]()"; boolean matched4 = AreParenthesesBracketsNested(str3); System.out.println(str4 + " is nested properly: " + matched4); String str5 = "[{})()"; boolean matched5 = AreParenthesesBracketsNested(str5); System.out.println(str5 + " is nested properly: " + matched5); java.util.Scanner input = new java.util.Scanner(System.in); System.out.println("Enter a string of (, ), [, ], {, and }:"); String str = input.next(); boolean matched = AreParenthesesBracketsNested(str); System.out.println(str + " is nested properly: " + matched); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
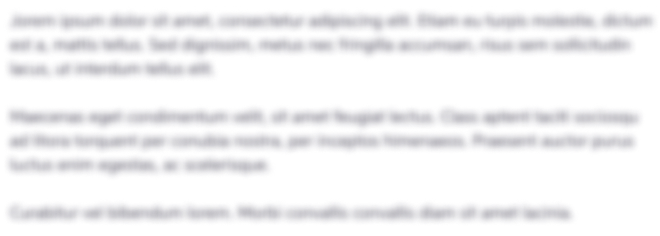
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started