Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Complete the python code. The d_linked_list is on this following page: https://www.chegg.com/homework-help/questions-and-answers/complete-python-code-doubly-linked-list-class-dlinkednode-def-init-self-initdata-initnext--q27312136 from d_linked_list import d_linked_list class m_sorted_list: def __init__(self, m_sorted): #Initialization of the class
Complete the python code. The d_linked_list is on this following page:
https://www.chegg.com/homework-help/questions-and-answers/complete-python-code-doubly-linked-list-class-dlinkednode-def-init-self-initdata-initnext--q27312136 from d_linked_list import d_linked_list class m_sorted_list: def __init__(self, m_sorted): #Initialization of the class with parameter mSorted def add(self, item): #Adds the item to the correct position if the list is sorted, # or adds the item to the end of the list if the list is not sorted def pop(self): #Removes and returns the first item in the list if the list is not sorted, # or returns and removes the largest number in the list if it is sorted def search(self, item): # Returns a tuple, with the first element being true or false if the item # was found in the list or not,and the second element is the index of the # of the item if it was found, or if it is not found, if the list is sorted # it is the index of the first item that is larger than item, and -1 if the # list is unsortedor there is no larger item in the list. def change_sorted(self): # Change the list from sorted to unsorted, or raises an exception with the # message I dont know how to sort a doubly linked list yet def get_size(self): # return the size of the lined list return self.__size def get_item(self, pos): # get the item at pos if pos >= 0: index = 0 current = self.__head while current != None: if index == pos: return current.getData() current = current.getNext() index = index + 1 else: index = -1 current = self.__tail while current != None: if index == pos: return current.getData() current = current.getPrevious() index = index - 1 return None def __str__(self): # return string representation of linked list if (self.get_size() == 0): return "Empty list" current = self.__head nodeList = "" while (current.getNext() != None): nodeList = nodeList + str(current.getData()) + " " current = current.getNext() nodeList = nodeList + str(current.getData()) return nodeList def test(): sor_list = m_sorted_list(True) is_pass = (sor_list.get_size() == 0) assert is_pass == True, "fail the test" sor_list.add(4) sor_list.add(3) sor_list.add(8) sor_list.add(7) sor_list.add(1) is_pass = (str(sor_list) == "1 3 4 7 8") assert is_pass == True, "fail the test" is_pass = (sor_list.get_size() == 5) assert is_pass == True, "fail the test" is_pass = (sor_list.pop() == 8) assert is_pass == True, "fail the test" is_pass = (sor_list.pop() == 7) assert is_pass == True, "fail the test" is_pass = (str(sor_list) == "1 3 4") assert is_pass == True, "fail the test" a = sor_list.search(2) is_pass = (a[0] == False and a[1] == 1) assert is_pass == True, "fail the test" a = sor_list.search(3) is_pass = (a[0] == True and a[1] == 1) assert is_pass == True, "fail the test" a = sor_list.search(7) is_pass = (a[0] == False and a[1] == -1) assert is_pass == True, "fail the test" is_pass = (sor_list.get_size() == 3) assert is_pass == True, "fail the test" is_pass = (sor_list.get_item(2) == 4) assert is_pass == True, "fail the test" sor_list.change_sorted() sor_list.add(1) is_pass = (str(sor_list) == "1 3 4 1") assert is_pass == True, "fail the test" is_pass = (sor_list.get_size() == 4) assert is_pass == True, "fail the test" is_pass = (sor_list.pop() == 1) assert is_pass == True, "fail the test" is_pass = (sor_list.pop() == 3) assert is_pass == True, "fail the test" sor_list.add(7) sor_list.add(6) is_pass = (str(sor_list) == "4 1 7 6") assert is_pass == True, "fail the test" a = sor_list.search(2) is_pass = (a[0] == False and a[1] == -1) assert is_pass == True, "fail the test" a = sor_list.search(7) is_pass = (a[0] == True and a[1] == 2) assert is_pass == True, "fail the test" a = sor_list.search(8) is_pass = (a[0] == False and a[1] == -1) assert is_pass == True, "fail the test" is_pass = (sor_list.get_size() == 4) assert is_pass == True, "fail the test" is_pass = (sor_list.get_item(2) == 7) assert is_pass == True, "fail the test" sor_list2 = m_sorted_list(False) is_pass = (sor_list2.get_size() == 0) assert is_pass == True, "fail the test" sor_list2.add(4) sor_list2.add(3) sor_list2.add(8) sor_list2.add(7) sor_list2.add(1) is_pass = (str(sor_list2) == "4 3 8 7 1") assert is_pass == True, "fail the test" is_pass = (sor_list2.get_size() == 5) assert is_pass == True, "fail the test" is_pass = (sor_list2.pop() == 4) assert is_pass == True, "fail the test" is_pass = (sor_list2.pop() == 3) assert is_pass == True, "fail the test" is_pass = (str(sor_list2) == "8 7 1") assert is_pass == True, "fail the test" a = sor_list2.search(2) is_pass = (a[0] == False and a[1] == -1) assert is_pass == True, "fail the test" a = sor_list2.search(7) is_pass = (a[0] == True and a[1] == 1) assert is_pass == True, "fail the test" is_pass = (sor_list2.get_size() == 3) assert is_pass == True, "fail the test" is_pass = (sor_list2.get_item(2) == 1) assert is_pass == True, "fail the test" try: sor_list2.change_sorted() except Exception as e: is_pass = True else: is_pass = False assert is_pass == True, "fail the test" sor_list2.add(3) sor_list2.add(2) is_pass = (str(sor_list2) == "8 7 1 3 2") assert is_pass == True, "fail the test" is_pass = (sor_list2.get_size() == 5) assert is_pass == True, "fail the test" is_pass = (sor_list2.pop() == 8) assert is_pass == True, "fail the test" is_pass = (sor_list2.pop() == 7) assert is_pass == True, "fail the test" is_pass = (str(sor_list2) == "1 3 2") assert is_pass == True, "fail the test" if is_pass == True: print ("=========== Congratulations! Your have finished exercise 2! ============") if __name__ == '__main__': test()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
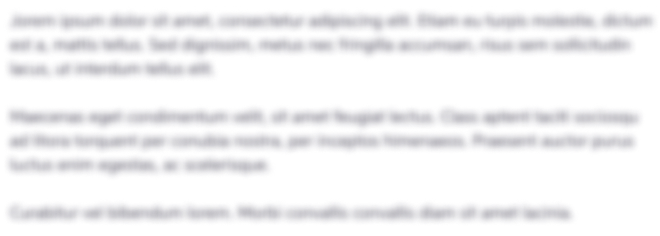
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started