Question
Computer Science Data Structures and Program Design Programming Project #1 Day Planner In this project we will develop classes to implement a Day Planner program.
Computer Science Data Structures and Program Design
Programming Project #1 Day Planner
In this project we will develop classes to implement a Day Planner program. Be sure to develop the code in a step by step manner, finish phase 1 before moving on to phase 2, then phase 3. Phase 1
Create a Netbeans Java Application project named 'Project1', After you select 'New Project' make sure it is set to 'Java-Java Application' and hit next. On the second screen you MUST change the project name to 'Project1', if you need to you can change the Project Location. But keep the rest to the default values and hit 'Finish'. When properly set up you should have a project named 'Project1', it contains a package named 'project1' (lowercase p) and a class named 'Project1' in a file named 'Project1.java'
If somehow you end up with code in the default package, or a strange package name, close the project, delete the folder and try again.
First thing we are going to do is test the UserInput class from Project0 (note: project 0 attached below, you can either copy and paste UserInput directly into Project1 file or create a project0 with code attacked below to keep following directions in paragraph ahead). Have BOTH projects open at the SAME time, right click and 'COPY' UserInput.java then right click on PACKAGE 'project1' and 'PASTE' (refactor), when done correctly Project1.java and UserInput.java will be right next to each other in the package 'project1'. You can close 'Project0'. This is not the best way to share code between projects, but I want it this way so UserInput is included when you turn in Project1. (optional just copy and paste code at bottom of question into another file)
In main of Project1.java ask the user to enter a number between 3 and 8 then use UserInput to get the value, this is your array size.
Create an integer array with the size input. When testing make SURE UserInput will not allow a value less than 3 or greater than 8.
In main of Project1.java ask the user to enter a number between 100 and 1000 then use UserInput to get the value, this is the maximum value to insert into the array.
Write a loop to fill the array with random numbers (with values between 0 and the maximum)
Insert each new number in the proper sorted position by shifting larger values before inserting a new value.
If values 300 and 500 are currently in the array and 400 is the NEW random number, shift 500 and insert 400 in the middle: 300 400 500
Writing a method insertValue() with parameters would be a nice touch, but is not required.
DO NOT insert all the numbers first then sort the array AFTER the inserts. Each new value must go in the proper spot.
Print the array from 0 to the end after the loop fills the entire array.
Phase 2
The class Appointment is essentially a record; an object built from this class will represent an Appointment in a Day Planner . It will contain 5 fields: month (3 character String), day (int), hour (int), minute (int), and message (String from 5 to 40 characters).
Write 5 get methods and 5 set methods, one for each data field. Make sure the set methods verify the data. (E.G. month is a valid 3 letter code). Simple error messages should be displayed when data is invalid, and the current value should NOT change.
Write 2 constructor methods for the class Appointment . One with NO parameters that assigns default values to each field and one with 5 parameters that assigns the values passed to each field. If you call the set methods in the constructor(s) you will NOT need to repeat the data checks.
Write a toString method for the class Appointment . It should create and return a nicely formatted string with ALL 5 fields. Pay attention to the time portion of the data, be sure to format it like the time should be formatted ( HH : MM ) , a simple if-else statement could add a leading zero, if needed.
Write a method inputAppointment () that will use the class UserInput from a previous project, ask the user to input the information and assign the data fields with the users input. Make sure you call the UserInput methods that CHECK the min/max of the input AND call the set methods to make sure the fields are valid.
Write a main() method, should be easy if you have created the methods above, it creates a Appointment object, calls the inputAppointment () method to input values and uses the method toString() print a nicely formatted Appointment object to the screen. As a test, use the constructor with 5 parameters to create a second object (you decide the values to pass) and print the second object to the screen. The primary purpose of this main() method is to test the methods you have created in the Appointment class.
Writing get and set methods, is sort of Object Oriented Programming for Dummies. The next step is to generate information from the data stored in the object. Write a method named getMonthNumber() which returns a number from 1 to 12. DO NOT STORE THIS NUMBER IN THE OBJECT. When the method is called it will look at the 3 letter variable month and return the proper value (ex. "Feb" returns 2). Return -1 if the 3 letter code is bad. Make it case insensitive so FEB, feb, Feb, fEb all return 2.
After that the next step is to generate MORE information from the data stored in the object. Write a method named getDateNumber() which returns a a large number representing the ENTIRE date, such that a date in Jan is less than a date in Feb, which is less than Mar and so on. For example, the crude way is to use strings:
month day hour min "01" "03" "08" "30" --> "01030830" > convert and return int 1030830 "02" "12" "06" "00" --> "02120600" > convert and return int 2120600
You can then easiliy compare two dates and tell the 1st date is less than the 2nd date.
Using strings is easy to see how it works, but it is ugly. Do the math! Call getMonthNumber() and use multiplication to convert each date to a number. Beware of single digit numbers. TEST YOUR CODE. After you print a Appointment object display the number generated by getDateNumber(). Verify a date in Jan is less than a date in Feb and so on.
Phase 3
Create a class Planner , in the data area of the class declare an array of 20 Appointment objects. Make sure the array is private (data abstraction).
In this project we are going to build a simple Day Planner program that allow the user to create various Appointment objects and will insert each into an array. Be sure to insert each Appointment object into the array in the proper position, according to the date and time of the Appointment . This means the earliest Appointment object should be at the start of the array, and the last Appointment object at the end of the array.
Please pre load your array with the following Appointment objects: May 6, 17:30 Quiz 2 Apr 1, 17:30 Midterm Jun 3, 17:30 Final Mar 4, 17:30 Quiz 1
YOU MUST CREATE THEM IN THIS ORDER, DO NOT PRE-SORT THEM.
Notice how the objects are NOT ordered, use the number generated by getDateNumber() to insert them into the proper position in the array with the earliest date at the start of the array and the latest at the end of the array.
Loop through the array and print the 4 objects in the proper order, they SHOULD come out in date order.
Call the method inputAppointment () to input and create a new object. Insert the NEW object into the array in the proper position.
Loop through the array and print the 5 objects in the proper order, they SHOULD come out in date order.
You may add additional methods to the Planner and Appointment classes as long as you clearly document 'what' and 'why' you added the method at the top of the class. The Appointmentclass could use one or more constructor methods. DO NOT in any way modify the UserInput class. If it so much as refers to a day or month or anything else in the Planner or Appointmentclass there will be a major point deduction.
Project0 code fo UserInput
UserInput.java
import java.util.Scanner;
public class UserInput {
static Scanner scan = new Scanner(System.in);
public static int getInt() { while (true) { try { return Integer.parseInt(scan.nextLine()); } catch (Exception e) { System.out.println("Invalid Input, Try again..."); } } }
public static int getInt(int min, int max) { while (true) { int input = getInt(); if (input <= max && input >= min) { return input; } else { System.out.println("Input not in range, Try again..."); } } }
public static char getChar() { while (true) { String s = getString(); if (s.length() == 1) { return s.charAt(0); } else { System.out.println("Invalid Char, Try Again..."); } } }
public static char getChar(char min, char max) { while (true) { char c = getChar(); c = Character.toLowerCase(c); if ((c >= min && c <= max)) { return c; } else { System.out.println("Char not in range, Try Again..."); } } }
public static double getDouble() { while (true) { try { return Double.parseDouble(scan.nextLine()); } catch (Exception e) { System.out.println("Invalid input, Try again..."); } } }
public static double getDouble(double min, double max) { while (true) { double d = getDouble(); if (d >= min && d <= max) { return d; } else { System.out.println("Input not in range, Try Again..."); } } }
public static String getString() { return scan.nextLine(); }
public static String getString(int min, int max) { while (true) { String s = getString(); if (s.length() >= min && s.length() <= max) { return s; } else { System.out.println("Input not in range, Try Again..."); } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
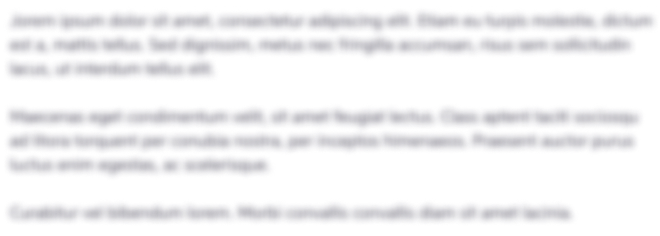
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started