Question
Computer Science II func. assignment #1 This is an example of a function where you do not pass any parameters to the function and nothing
Computer Science II func. assignment #1
This is an example of a function where you do not pass any parameters to the function and nothing is returned to the calling function. (the function returns void)Write a C function that will : 1. in the function, ask the user for a number of gallons
- convert a given number of gallons into quarts, liters, cups, and fluid ounce.
- print out the answer in the function
- the output should look like:
??? gallons is: ??? quarts ???
liters ???
cups ???
fluid ounces
function header should be void convertGallons()
in main
- call your function
- the output should look like:
??? gallons is: ??? quarts
??? liters
??? cups
??? fluid ounces
Use the output (cout) manipulators to format the output correctly [setw(?), fixed, right, left, etc]
Computer Science II func. assignment #2
This is an example of a function where, (in the function) you read in 3 numbers, do a calculation, and return a value
Write a C function that will:
- Determine if you have entered a right triangle
- Accept 3 ints (in main)
- Length of side one of the triangle
- Length of side two of the triangle
- Length of side three of the triangle
- returns a bool (true or false) whether the 3 lengths entered determines a right triangle
- the function does not print out the answer, just returns the answer
- answer is printed in main
- the three sides of the triangle are printed in the function
- the length of the sides are not input in any particular order
function header should be
bool determineRightTri()
in the function
- ask for the length of one side of a triangle
- read in this length
- ask for the length of one the second side of a triangle
- read in this length
- ask for the length of one the third side of a triangle
- read in this length
- determine whether this is a right triangle
- return true if you have a right triangle, else false
Output should look like the following
??? length of side 1
??? length of side 2
??? length of side 3
is a right triangle
Or
is not a right triangle
these lines are printed in the function
??? length of side 1
??? length of side 2
??? length of side 3
this is printed in main--using the returned Boolean value
is a right triangle
Or
is not a right triangle
Use the output (cout) manipulators to format the output correctly [setw(?), fixed, right, left, etc]
Computer Science Ii func. assignment #3
Write a C program that has 1 function:
void giveDays(int days, int weeks)
- accepts as parameters two ints. The first is a number of days and the second is a number of weeks
- calculates how many total days are represented by the 2 parameters
Example: 25 days and 3 full weeks represents a total number of days of 46
- print out (in the function) how many total days are calculated
function headers should be:
void giveDays(int days, int weeks)
in main
- ask for the number of days
- read in the number of days
- ask for the number of weeks
- read in the number ofweeks
- call your function giveDays(int, int)
output from the function should look like:
??? days
??? weeks
has ??? total days
Use the output (cout) manipulators to format the output correctly [setw(?), fixed, right, left, etc]
Computer Science II func. assignment #4
Write a C program that has 1 function:
- accepts as parameters two ints (row and column)
- evaluates the row and column and determines if the row and columns numbers would be on a checker board.
- In other words, are the row and column numbers greater than or equal to 0 and less than or equal to 7
- Are the row and column numbers contained on an 8X8 array
- Return true if the row and column numbers are in the array else return false
function headers should be:
bool evaluateRowCol(int row, int col)
in main
- ask for the row and column numbers
- read in the row and column numbers numbers
- call your function bool evaluateRowCol(int row, int col)
- output in main should be
row ?
column ?
is on the checker board
or
is not on the checker board
Use the output (cout) manipulators to format the output correctly [setw(?), fixed, right, left, etc] Computer Science II assignment #5
Write a C program named assignment04.cpp that will
- ask for and read in a float for a radius
- ask for and read in a float for the height
- each of the following functions will calculate some property of a shape (area, volume, circumference, etc)
- The functions will accept one or two parameters (radius and/or height) and return the proper calculation.
- There is no input/output (cin or cout) in the function just the calculation
- write the following functions
- float areaCircle(float radius)
where the area of a circle is calculated by
area = PI * radius * radius
- float circumCircle(float radius)
where the circumference of a circle is calculated by
circumference = PI * radius * 2
- float areaCylinder(float radius, float height)
where the area of a cylinder is calculated by
area = 2 * PI * radius * radius + height * PI * radius * 2
- float volumeCylinder(float radius, float height)
where the volume of a cylinder is calculated by
volume = PI * radius * radius * height
- float volumeCone(float radius, float height)
where the area of a cylinder is calculated by
volume = (1 / 3) * (PI * radius * radius * height)
All input and output (cin and cout) are in maim()
in main()
- get the radius and height
- call each of the functions and print out the answer
- output should look like
radius is ??????
height is ??????
area of the circle is ????????
circumference of the circle is ????????
area of the cylinder is ????????
volume of the cylinder is ????????
volume of the cone is ????????
Step by Step Solution
There are 3 Steps involved in it
Step: 1
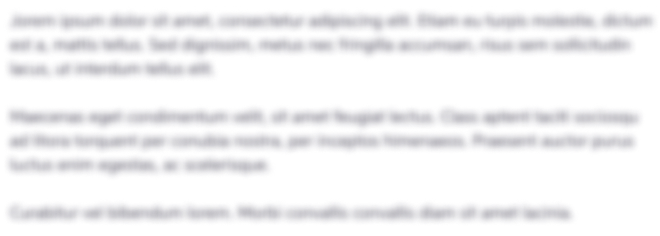
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started