Question
Consider the classes class cashRegister and dispenserTypegiven in the Programming Example Juice Machine in Chapter 10 . In the class cashRegister, add the functions to
Consider the classes class cashRegister and dispenserTypegiven in the Programming Example Juice Machine in Chapter 10.
In the class cashRegister, add the functions to overload the binary operators + and to add and subtract an amount in a cash register; the relational operators to compare the amount in two cash registers; and the stream insertion operator for easy output.
The class dispenserType, in the Programming Example Juice Machine in Chapter 10, is designed to implement a dispenser to hold and release products. In this class, add the functions to overload the increment and decrement operators to increment and decrement the number of items by one, respectively, and the stream insertion operator for easy output.
Write a program to test the classes designed in steps 1 and 2.
cashRegister.cpp
#include
#include "cashRegister.h"
using namespace std;
int cashRegister::getCurrentBalance() const { return cashOnHand; }
cashRegister::cashRegister(int cashIn) { if (cashIn >= 0) cashOnHand = cashIn; else cashOnHand = 500; }
// Define the functions to overload the '+' and '-' operators below
bool cashRegister::operator==(const cashRegister& cashR) const { return (cashOnHand == cashR.cashOnHand); } bool cashRegister::operator!=(const cashRegister& cashR) const { return (cashOnHand != cashR.cashOnHand); } bool cashRegister::operator<=(const cashRegister& cashR) const { return (cashOnHand <= cashR.cashOnHand); }
bool cashRegister::operator<(const cashRegister& cashR) const { return (cashOnHand < cashR.cashOnHand); }
bool cashRegister::operator>=(const cashRegister& cashR) const { return (cashOnHand >= cashR.cashOnHand); }
bool cashRegister::operator>(const cashRegister& cashR) const { return (cashOnHand > cashR.cashOnHand); }
ostream& operator<<(ostream& os, const cashRegister& cashR) { os << cashR.cashOnHand;
return os; }
cashRegister.h
#include
using namespace std; class cashRegister { friend ostream& operator<<(ostream&, const cashRegister&); public: int getCurrentBalance() const;
// Declare the functions for the overloaded operators '+' and '-' below
bool operator==(const cashRegister& cashR) const; bool operator!=(const cashRegister& cashR) const; bool operator<=(const cashRegister& cashR) const; bool operator<(const cashRegister& cashR) const; bool operator>=(const cashRegister& cashR) const; bool operator>(const cashRegister& cashR) const;
cashRegister(int cashIn = 500);
private: int cashOnHand; //variable to store the cash //in the register };
dispenserType.cpp
//********************************************************** // Author: D.S. Malik // // Implementation file dispenserType.cpp // This file contains the definitions of the functions to // implement the operations of the class dispenserType. //********************************************************** #include
using namespace std;
int dispenserType::getNoOfItems() const { return numberOfItems; }
int dispenserType::getCost() const { return cost; }
// Define the functions for the pre- and post- increment and decrement operators below. dispenserType::dispenserType(int setNoOfItems, int setCost) { if (setNoOfItems >= 0) numberOfItems = setNoOfItems; else numberOfItems = 50;
if (setCost >= 0) cost = setCost; else cost = 50; }
ostream& operator<<(ostream& os, const dispenserType& disp) { os << "Cost: " << disp.cost << ", quantity " << disp.numberOfItems;
return os; }
dispenserType.h
#include
using namespace std; class dispenserType { friend ostream& operator<<(ostream&, const dispenserType&); public: int getNoOfItems() const;
int getCost() const;
void makeSale();
// Declare the functions for the overloaded increment and decrement operators below dispenserType operator++(); dispenserType operator++(int); dispenserType operator--(); dispenserType operator--(int);
dispenserType(int setNoOfItems = 50, int setCost = 50);
private: int numberOfItems; //variable to store the number of //items in the dispenser int cost; //variable to store the cost of an item };
most write code in c++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
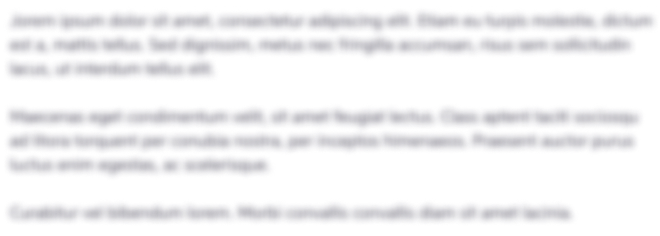
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started