Question
Consider the classic lock-based solution to the dining philosophers problem (according to Wikipedia): Five silent philosophers sit at a table around a bowl of spaghetti.
Consider the classic lock-based solution to the dining philosophers problem (according to Wikipedia):
Five silent philosophers sit at a table around a bowl of spaghetti. A fork is placed between each pair of adjacent philosophers.
Each philosopher must alternately think and eat. Eating is not limited by the amount of spaghetti left: assume an infinite supply. However, a philosopher can only eat while holding both the fork to the left and the fork to the right (an alternative problem formulation uses rice and chopsticks instead of spaghetti and forks).
Each philosopher can pick up an adjacent fork, when available, and put it down, when holding it. These are separate actions: forks must be picked up and put down one by one.
The problem is how to design a discipline of behavior (a concurrent algorithm) such that each philosopher won't starve, i.e. can forever continue to alternate between eating and thinking.
In this implementation, there are n philosophers with id number i, and each thread runs the following pseudocode based on an array of pthread_mutex_t structures called fork_lock: fork_lock[i].acquire(); fork_lock[(i+1)%n].acquire(); // eat fork_lock[i].release(); fork_lock[(i+1)%n].release(); this solution can deadlock, though it might not right away. In fact, there are four causes to this deadlock, which must be simultaneously met for deadlock to actually occur:
Mutual Exclusion: two or more threads (philosophers) want the same resource (fork), and if one has it, the other(s) do not
No Preemption: Once the fork is picked up, it cannot be taken away to resolve the fight
Circular Dependency: Thread A wants resource X and is holding resource Y, while Thread B wants resource Y and is holding X
Hold-And-Wait: Each thread holds one (or more) of the needed resources while waiting for the other(s)
Correcting any of these four conditions removes the deadlock situation. Fix the above solution to the dining philosophers problem by correcting the hold-and-wait condition. That is, if a philosopher picks up a single fork, that philosopher is guaranteed to pick up both forks, or else the philosopher will not attempt to pick up any forks (by blocking). You may introduce additional pthread_mutex_t locks, or change the pseudocode. Explain your changes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
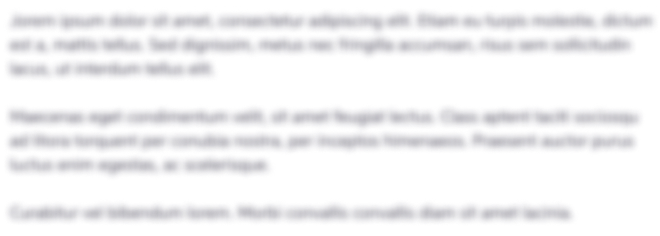
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started