Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Consider the following function: int func(int list[], int size) { int sum = 0; for (int index = 0; index < size; index++) sum =
- Consider the following function:
int func(int list[], int size)
{
int sum = 0;
for (int index = 0; index < size; index++)
sum = sum + list[index];
return sum;
}
- Find the number of operations executed by the function func() if the value of size is 10.
- Find the number of operations executed by the function func() if the value of size is n.
- What is the order (Big-O notation) of the function func()?
- Write a program for a clerk of Fresh Fruit Store so that the clerk will input the items that a customer buys and then print out the name, unit price, quantity, and cost of all item and the total cost as a receipt.
The program should do the following:
- Define a new data type, fruit, as a C++ class. The class fruit should include:
- The name of the fruit in an item. Ex: Apple, Banana, ..
- The unit price of the fruit in the item. Ex: 0.99 ($/lb), 1.29 ($/lb), .
- The quantity of the fruit that the customer buys. Ex. 2 lbs, 3.5 lbs, ..
- Set the name, unit price, and quantity of the fruit in the item.
- Set the name of the fruit in the item only.
- Set the unit price of the fruit in the item only.
- Set the quantity of the fruit in the item only.
- Return the name of the fruit in the item.
- Return the unit price of the fruit in the item.
- Return the quantity of the fruit in the item.
- Return the cost of the item. (cost = unit price * quantity)
- Constructor with three default value
name = , unit price = 0.0, quantity = 0.0.
- Overloading the operator << to print the item in the following format:
Ex:
Apple $0.99/lb 3 lb $2.97
- Write a function to show the name and unit price of each kind of fruit sold in the store.
- Write a function to return the total cost in the shopping list.
- Write a function to show all items in the shopping list, including the number, the name, the unit price, the quantity, and the cost of each item.
Ex:
Item Name Unit Price Quantity Cost
1 Apple $0.99/lb 3 lb $2.97
2 Grape $1.79/lb 2 lb $3.58
3 Banana $0.45/lb 2.2lb $0.99
:
- Do the following operations in the main function:
- Create an array of type fruit as a shopping list. Suppose the max size of array is 100.
- Show the selection of the fruit in the storeAsk to select a kind of fruit and the quantity to buy, and store in an element of the array. Repeat the same operation until select 9 exit.
- Show all items in the shopping list, including the number, the name, the unit price, the quantity, and the cost of each item, and also show the total cost.
- Show all items in the shopping list and ask whether to change the quantity. If yes, ask to select the number of the item needed to change. Write a function to change the quantity of the selected item by inputting the number of the item and the new quantity.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
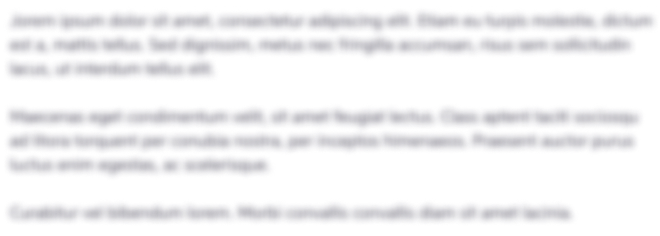
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started