Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Controlling Program Flow w/i an ATM Using selection statements & loops to complete the following: Validate the user's PIN -- only allow them to
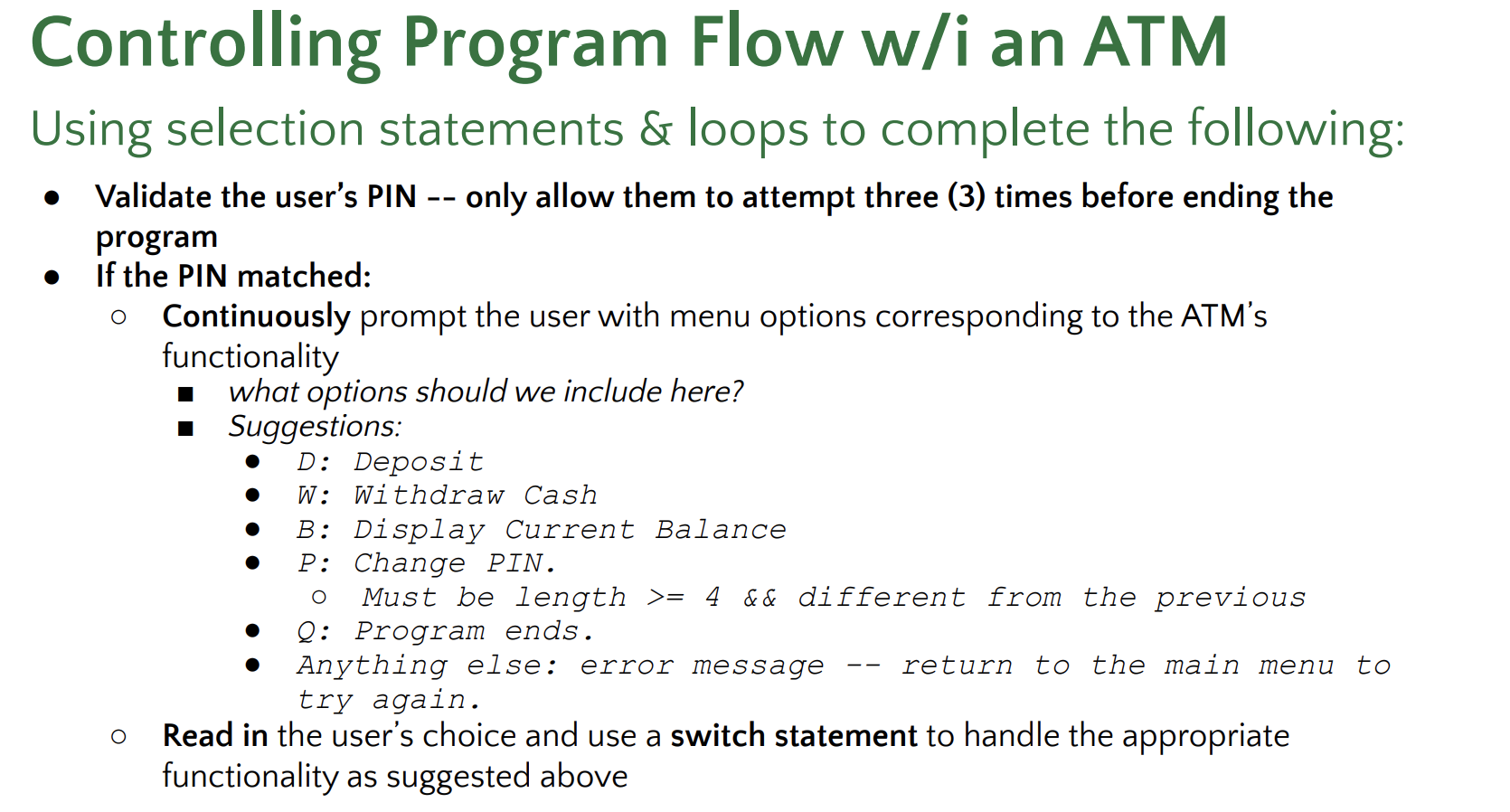
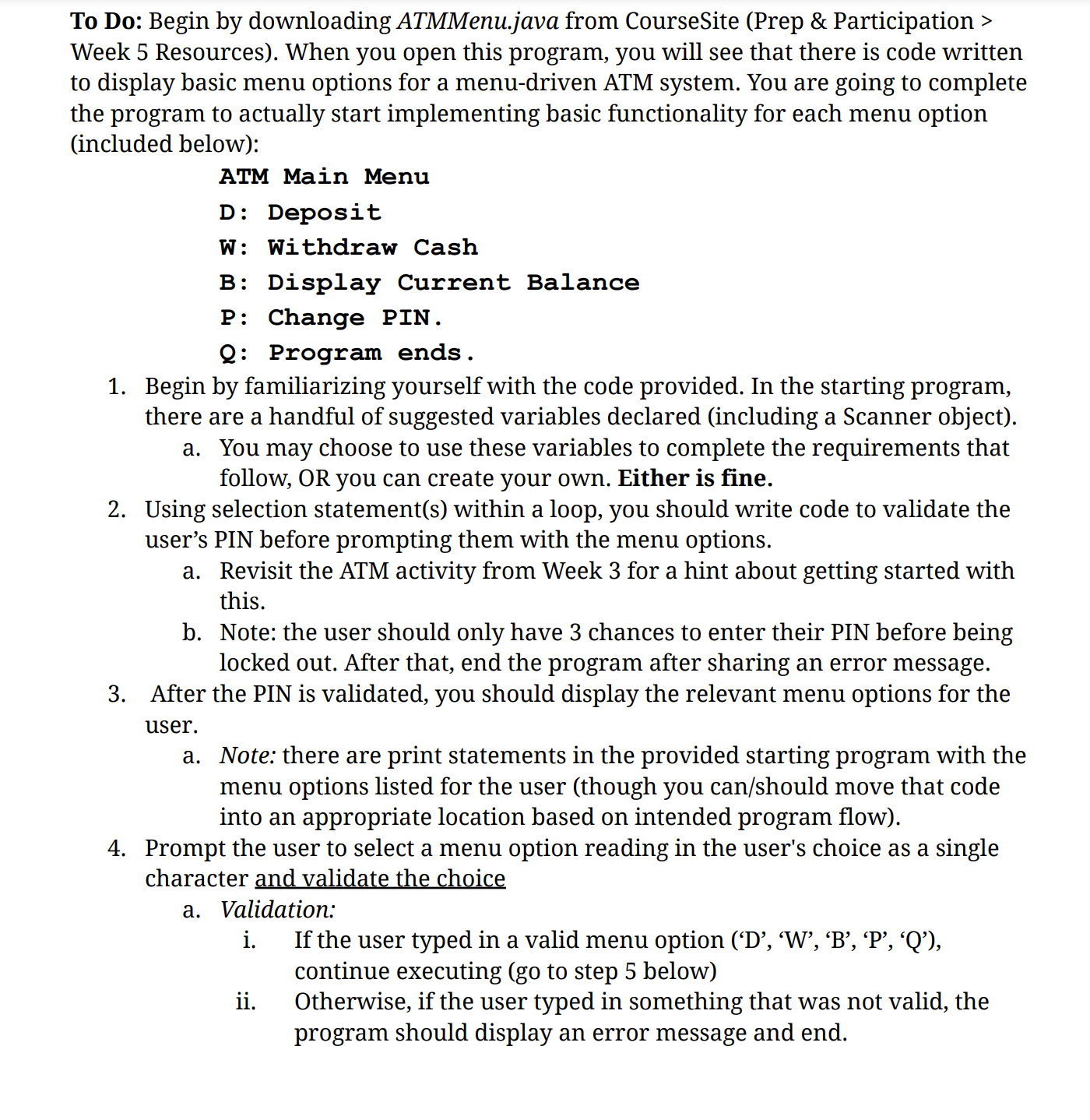
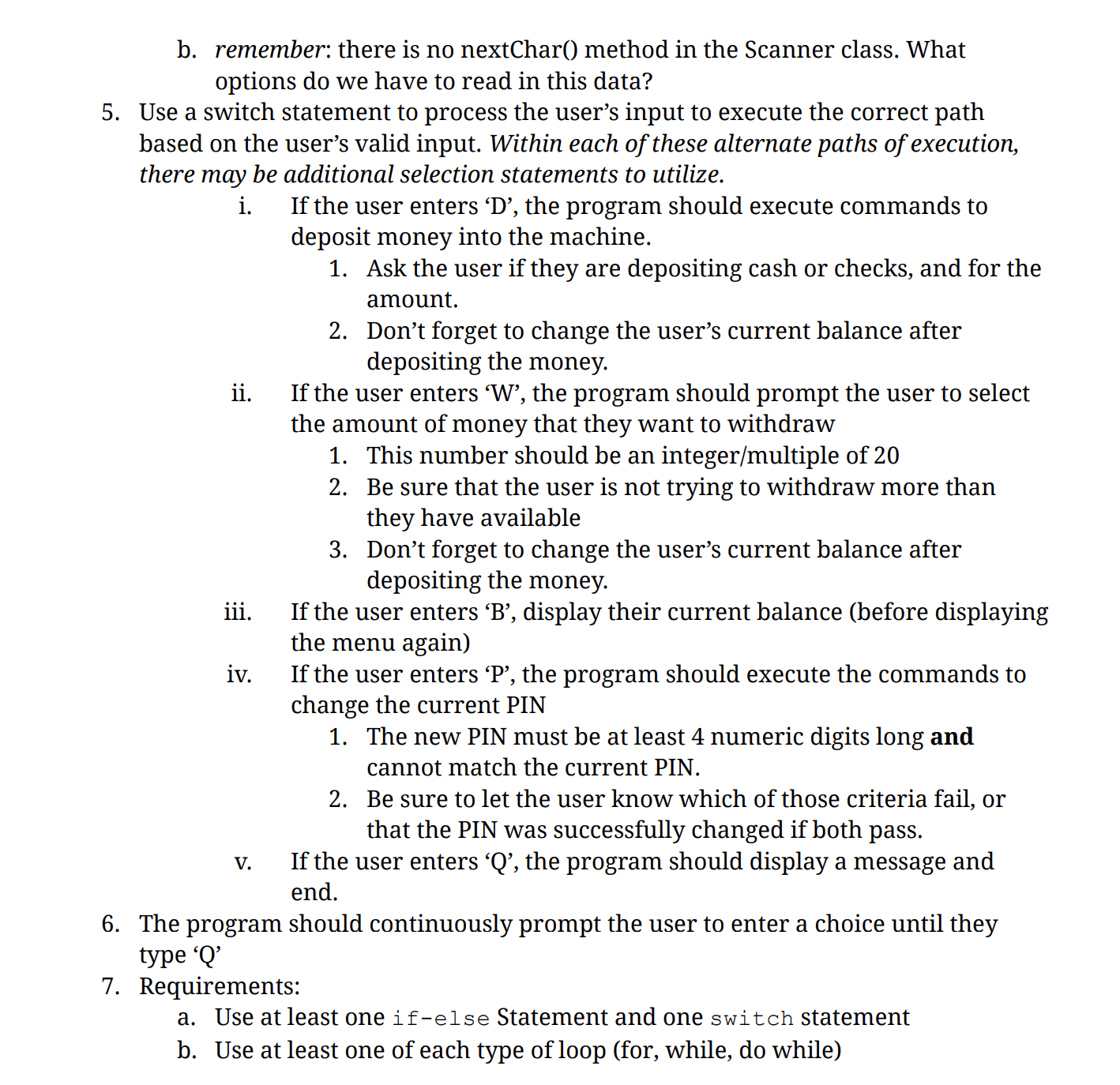
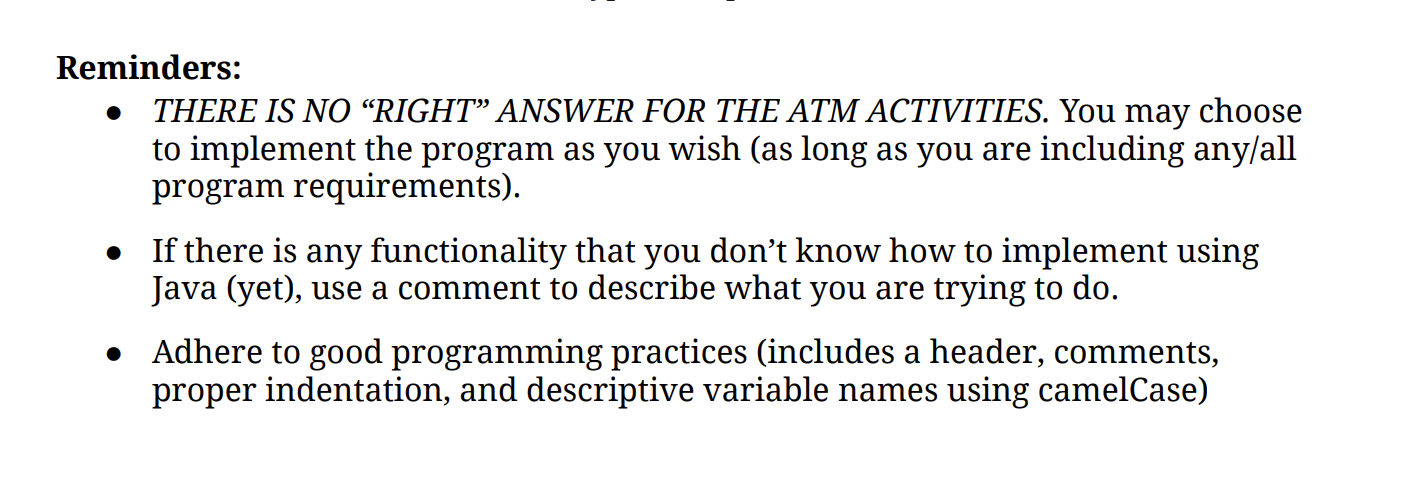
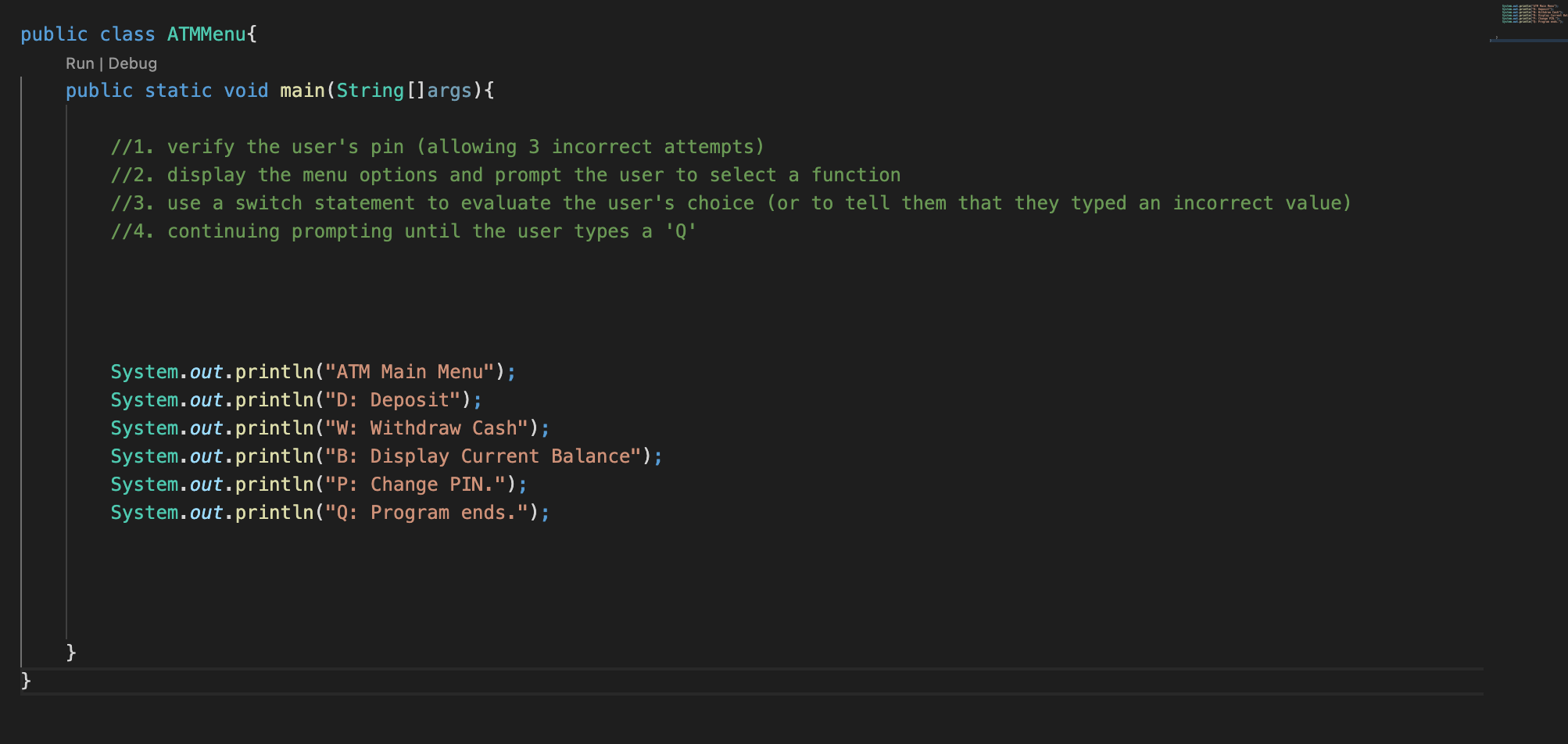
Controlling Program Flow w/i an ATM Using selection statements & loops to complete the following: Validate the user's PIN -- only allow them to attempt three (3) times before ending the program If the PIN matched: O Continuously prompt the user with menu options corresponding to the ATM's functionality what options should we include here? Suggestions: D: Deposit W: Withdraw Cash B: Display Current Balance P: Change PIN. Must be length >= 4 && different from the previous Q: Program ends. Anything else: error message -- return to the main menu to try again. Read in the user's choice and use a switch statement to handle the appropriate functionality as suggested above To Do: Begin by downloading ATMMenu.java from CourseSite (Prep & Participation > Week 5 Resources). When you open this program, you will see that there is code written to display basic menu options for a menu-driven ATM system. You are going to complete the program to actually start implementing basic functionality for each menu option (included below): ATM Main Menu D: Deposit W: Withdraw Cash B: Display Current Balance P: Change PIN. Q: Program ends. 1. Begin by familiarizing yourself with the code provided. In the starting program, there are a handful of suggested variables declared (including a Scanner object). a. You may choose to use these variables to complete the requirements that follow, OR you can create your own. Either is fine. 2. Using selection statement(s) within a loop, you should write code to validate the user's PIN before prompting them with the menu options. a. Revisit the ATM activity from Week 3 for a hint about getting started with this. b. Note: the user should only have 3 chances to enter their PIN before being locked out. After that, end the program after sharing an error message. 3. After the PIN is validated, you should display the relevant menu options for the user. a. Note: there are print statements in the provided starting program with the menu options listed for the user (though you can/should move that code into an appropriate location based on intended program flow). 4. Prompt the user to select a menu option reading in the user's choice as a single character and validate the choice a. Validation: i. ii. If the user typed in a valid menu option ('D', 'W', B', P', Q'), continue executing (go to step 5 below) Otherwise, if the user typed in something that was not valid, the program should display an error message and end. b. remember: there is no nextChar() method in the Scanner class. What options do we have to read in this data? 5. Use a switch statement to process the user's input to execute the correct path based on the user's valid input. Within each of these alternate paths of execution, there may be additional selection statements to utilize. i. ii. iii. iv. V. If the user enters 'D', the program should execute commands to deposit money into the machine. 1. Ask the user if they are depositing cash or checks, and for the amount. 2. Don't forget to change the user's current balance after depositing the money. If the user enters 'W', the program should prompt the user to select the amount of money that they want to withdraw 1. This number should be an integer/multiple of 20 2. Be sure that the user is not trying to withdraw more than they have available 3. Don't forget to change the user's current balance after depositing the money. If the user enters 'B', display their current balance (before displaying the menu again) If the user enters P, the program should execute the commands to change the current PIN 1. The new PIN must be at least 4 numeric digits long and cannot match the current PIN. 2. Be sure to let the user know which of those criteria fail, or that the PIN was successfully changed if both pass. If the user enters Q', the program should display a message and end. 6. The program should continuously prompt the user to enter a choice until they type 'Q' 7. Requirements: a. Use at least one if-else Statement and one switch statement b. Use at least one of each type of loop (for, while, do while) Reminders: THERE IS NO RIGHT ANSWER FOR THE ATM ACTIVITIES. You may choose to implement the program as you wish (as long as you are including any/all program requirements). If there is any functionality that you don't know how to implement using Java (yet), use a comment to describe what you are trying to do. Adhere to good programming practices (includes a header, comments, proper indentation, and descriptive variable names using camelCase) } public class ATMMenu{ Run | Debug public static void main(String[] args) { //1. verify the user's pin (allowing 3 incorrect attempts) //2. display the menu options and prompt the user to select a function //3. use a switch statement to evaluate the user's choice (or to tell them that they typed an incorrect value) //4. continuing prompting until the user types a 'Q' } System.out.println("ATM Main Menu"); System.out.println("D: Deposit"); System.out.println("W: Withdraw Cash"); System.out.println("B: Display Current Balance"); System.out.println("P: Change PIN."); System.out.println("Q: Program ends.");
Step by Step Solution
★★★★★
3.42 Rating (146 Votes )
There are 3 Steps involved in it
Step: 1
Heres a basic implementation of the ATMMenu class based on the requirements provided import javautilScanner public class ATMMenu public static void ma...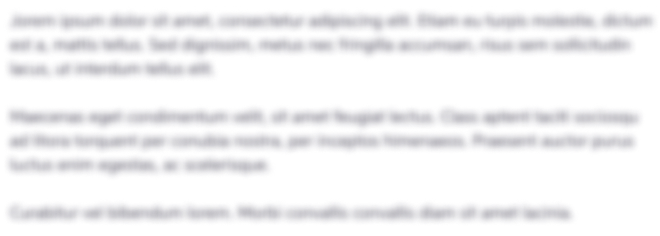
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started