Question
Convert this code into C++, provide the full execution of each function and library so that final code given by you gives the same output
Convert this code into C++, provide the full execution of each function and library so that final code given by you gives the same output as python code is giving. The output which i got from python code is - Python code -
from abc import ABC, abstractmethod import random from sympy import symbols, Eq, solve # Step 1: Define Abstract Components (Interfaces) class NetworkFunction(ABC): @abstractmethod def process_packet(self, packet): pass @abstractmethod def get_performance_metrics(self): pass @abstractmethod def get_implementations(self): pass class Classifier(ABC): @abstractmethod def classify(self, data): pass @abstractmethod def get_model_details(self): pass @abstractmethod def get_implementations(self): pass # Step 2: Implement Multiple Backing Implementations class PISASwitch(NetworkFunction): def process_packet(self, packet): # Symbolic execution for PISA switch symbolic_result = symbols('symbolic_result') return symbolic_result def get_performance_metrics(self): # Symbolic execution for performance metrics latency = symbols('latency') throughput = symbols('throughput') return {'Latency': latency, 'Throughput': throughput} def get_implementations(self): return [PISASwitch] class X86CPU(NetworkFunction): def process_packet(self, packet): # Symbolic execution for x86 CPU symbolic_result = symbols('symbolic_result') return symbolic_result def get_performance_metrics(self): # Symbolic execution for performance metrics latency = symbols('latency') throughput = symbols('throughput') return {'Latency': latency, 'Throughput': throughput} def get_implementations(self): return [X86CPU] class DecisionTreeClassifier(Classifier): def classify(self, data): # Symbolic execution for decision tree classifier symbolic_result = symbols('symbolic_result') return symbolic_result def get_model_details(self): # Symbolic execution for model details model_complexity = symbols('model_complexity') return {'Model Complexity': model_complexity} def get_implementations(self): return [DecisionTreeClassifier] class NeuralNetworkClassifier(Classifier): def classify(self, data): # Symbolic execution for neural network classifier symbolic_result = symbols('symbolic_result') return symbolic_result def get_model_details(self): # Symbolic execution for model details model_complexity = symbols('model_complexity') return {'Model Complexity': model_complexity} def get_implementations(self): return [NeuralNetworkClassifier] # Step 3: Search Process class SynthesisSearch: def __init__(self, components): self.components = components def search(self): best_solution = None best_score = float('-inf') for _ in range(10): # Number of iterations for the sake of example solution = self.generate_random_solution() score = self.evaluate_solution(solution) if score > best_score: best_solution = solution best_score = score return best_solution def generate_random_solution(self): solution = {} for component in self.components: implementation = random.choice(component.get_implementations()) solution[component] = implementation return solution def evaluate_solution(self, solution): # Simulate an evaluation process and return a score # This could involve performance metrics based on your objectives return random.randint(1, 100) # Step 4: Simulator class NetworkSimulator: def simulate(self, network_function, packets): for packet in packets: network_function.process_packet(packet) # Step 5: Performance Prediction Model class PerformancePredictionModel: def predict_performance(self, network_function, classifier): # A simple model that combines different factors to predict performance hardware_power = symbols('hardware_power') model_complexity = symbols('model_complexity') latency = symbols('latency') throughput = symbols('throughput') prediction = hardware_power - model_complexity + latency / throughput return prediction # Step 6: Example Usage if __name__ == "__main__": # Create instances of abstract components and their implementations pisa_switch = PISASwitch() x86_cpu = X86CPU() decision_tree_classifier = DecisionTreeClassifier() neural_network_classifier = NeuralNetworkClassifier() # Step 6: Search for the best deployment components = [pisa_switch, x86_cpu, decision_tree_classifier, neural_network_classifier] search_process = SynthesisSearch(components) best_solution = search_process.search() # Step 7: Simulate and evaluate the best solution best_network_function = best_solution[pisa_switch]() best_classifier = best_solution[decision_tree_classifier]() # Simulate packet processing symbolic_result_nf = best_network_function.process_packet({'symbolic_data': 42}) # Provide an actual packet # Simulate classification symbolic_result_classifier = best_classifier.classify({'symbolic_data': 42}) # Provide an actual data for classification # Symbolic execution for performance metrics and model details performance_metrics_nf = best_network_function.get_performance_metrics() model_details_classifier = best_classifier.get_model_details() # Solve symbolic equations to obtain concrete values solved_latency = solve(Eq(performance_metrics_nf['Latency'], 10)) # Replace 10 with an actual value solved_throughput = solve(Eq(performance_metrics_nf['Throughput'], 100)) # Replace 100 with an actual value solved_model_complexity = solve(Eq(model_details_classifier['Model Complexity'], 5)) # Replace 5 with an actual value prediction_model = PerformancePredictionModel() performance_prediction = prediction_model.predict_performance(best_network_function, best_classifier) print("Best Solution for Network Function:", best_network_function) print("Symbolic Result for Network Function:", symbolic_result_nf) print("Solved Latency:", solved_latency) print("Solved Throughput:", solved_throughput) print(" Best Solution for Classifier:", best_classifier) print("Symbolic Result for Classifier:", symbolic_result_classifier) print("Solved Model Complexity:", solved_model_complexity) print(" Performance Prediction:") print("Estimated Overall Performance:", performance_prediction)
Best Solution for Network Function: <_-main_-.pisaswitch object at> Symbolic Result for Network Function: symbolic_result Solved Latency: [10] Solved Throughput: [100] Best Solution for Classifier: <_-main_-.decisiontreeclassifier object at> Symbolic Result for Classifier: symbolic_result Solved Model Complexity: [5] Performance Prediction: Estimated Overall Performance: hardware_power + latency/throughput - model_complexity Process finished with exit code 0Step by Step Solution
There are 3 Steps involved in it
Step: 1
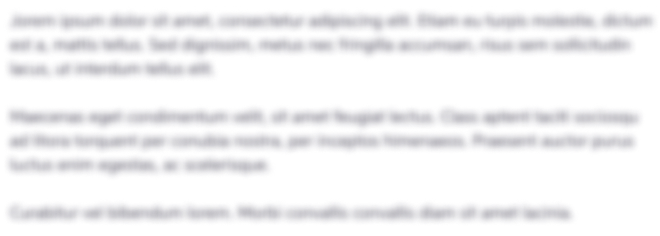
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started