Question
Conway's Game of Life Finally, we will model how the game evolves over time in a single plot. Modify your code from the previous step.
Conway's Game of Life
Finally, we will model how the game evolves over time in a single plot.
Modify your code from the previous step. Instead of storing the time steps as separate elements in a list, each time step should be added to an accumulator array pd_time. This will give us a "heat map" of how the system has evolved over time. Use pentadecathlon again.
pentadecathlon = np.zeros( ( 24,24 ) ) pentadecathlon[ 10:20,10 ] = 1 pentadecathlon[ 12, 9 ] = 1 pentadecathlon[ 12,10 ] = 0 pentadecathlon[ 12,11 ] = 1 pentadecathlon[ 17, 9 ] = 1 pentadecathlon[ 17,10 ] = 0 pentadecathlon[ 17,11 ] = 1
Your submission for this part should include the ndarray named pd_time.
Starter code:
import numpy as np import matplotlib.pyplot as plt pentadecathlon = np.zeros( ( 24,24 ) ) pentadecathlon[ 10:20,10 ] = 1 pentadecathlon[ 12, 9 ] = 1 pentadecathlon[ 12,10 ] = 0 pentadecathlon[ 12,11 ] = 1 pentadecathlon[ 17, 9 ] = 1 pentadecathlon[ 17,10 ] = 0 pentadecathlon[ 17,11 ] = 1 pd_time = ??? evoln = pentadecathlon[ : ] nt = 30 for t in ???: ??? # evolve the system forward in `evoln` pd_time += evoln plt.imshow( pd_time ) plt.show()
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Code from previous step:
import numpy as np import matplotlib.pyplot as plt
pentadecathlon = np.zeros( ( 24,24 ) ) pentadecathlon[ 10:20,10 ] = 1 pentadecathlon[ 12, 9 ] = 1 pentadecathlon[ 12,10 ] = 0 pentadecathlon[ 12,11 ] = 1 pentadecathlon[ 17, 9 ] = 1 pentadecathlon[ 17,10 ] = 0 pentadecathlon[ 17,11 ] = 1
pd_list = [] pd_list.append( pentadecathlon ) nt = 30 for t in range(nt): pentadecathalon = evolve( pentadecathlon ) pd_list.append( pentadecathlon ) # evolve the simulation and append it to `pd_list` plt.imshow( pd_list[ -1 ] ) plt.show()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
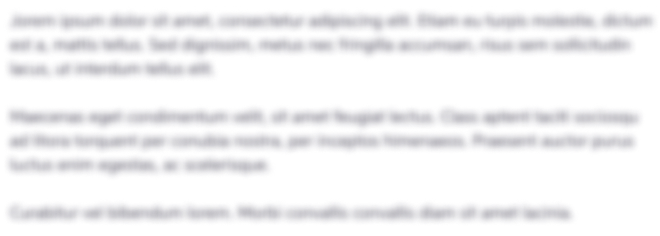
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started