Question
Could someone explain this mainly in Java, I understand how to do this mainly within a main function alone but using multiple methods is causing
Could someone explain this mainly in Java, I understand how to do this mainly within a main function alone but using multiple methods is causing a lot of errors.
Step 1: In the pseudocode below, declare the following variables under the documentation for Step 1.
- A variable called totalBottles that is initialized to 0
- This variable will store the accumulated bottle values
- A variable called counter and that is initialized to 1
- This variable will control the loop
- A variable called todayBottles that is initialized to 0
- This variable will store the number of bottles returned on a day
- A variable called totalPayout that is initialized to 0
- This variable will store the calculated value of totalBottles times .10
- A variable called keepGoing that is initialized to y
- This variable will be used to run the program again
Step 2: In the pseudocode below, make calls to the following functions under the documentation for Step 2.
- A function call to getBottles that passes totalBottles, todayBottles, and counter.
- A function called calcPayout that passes totalPayout and totalBottles.
- A function called printInfo that passes totalBottles and totalPayout
Step 3: In the pseudocode below, write a condition controlled while loop around your function calls using the keepGoing variable under the documentation for Step 3.
Complete Steps 1-3 below:
Module main ()
//Step 1: Declare variables below
Declare Integer totalBottles = 0
Declare Integer counter = 1
Declare Integer todayBottles = 0
Declare Real totalPayout = 0
Declare String keepGoing = y
//Step 3: Loop to run program again
While keepGoing == y
//Step 2: Call functions
getBottles (totalBottles, todayBottles, counter)
calcPayout (totalPayout, totalBottles)
printInfo (totalBottles, totalPayout)
Display Do you want to run the program again? (Enter y for yes).
Input keepGoing
End While
End Module
Step 4: In the pseudocode below, write the missing lines, including:
- The missing parameter list
- The missing condition (Hint: should run seven iterations)
- The missing input variable
- The missing accumulator
- The increment statement for the counter
//getBottles module
Module getBottles(Integer totalBottles, Integer todayBottles, Integer counter)
While counter <=7
Display Enter number of bottles returned for the day:
Input todayBottles
d. totalBottles = totalBottles + todayBottles
e. counter = counter + 1
End While
End Module
Step 5: In the pseudocode below, write the missing lines, including:
- The missing parameter list
- The missing calculation
//getBottles module
Module calcPayout(totalPayout, totalBottles)
totalPayout = 0 //resets to 0 for multiple runs
totalpayout = totalBottles * .10
End Module
Step 6: In the pseudocode below, write the missing lines, including:
- The missing parameter list
- The missing display statement
- The missing display statement
//printInfo module
Module printInfo(totalBottles, totalPayout)
Print Your total number of bottles is +totalBottles
Print Your payout is +totalPayout
End Module
Step by Step Solution
There are 3 Steps involved in it
Step: 1
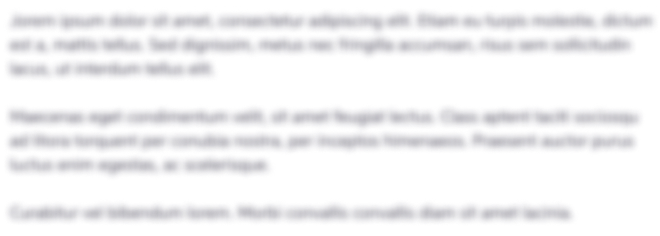
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started