Question
Could someone help me I am trying to make my program below read my input files into 6 test cases that the program will run
Could someone help me I am trying to make my program below read my input files into 6 test cases that the program will run for each expression separately. At the bottom are the 6 test cases. Thank you so much for the help.
//main.cpp
#include
#include
#include
using namespace std;
#include "expression.h"
#include "Subexpression.h"
#include "symboltable.h"
#include "parse.h"
#include
#include
SymbolTable symbolTable;
void parseAssignments(stringstream &in);
int main()
{
const int SIZE = 256;
Expression* expression;
char paren, comma, line[SIZE];
ifstream fin("input.txt");
//fin.open("input.txt");
// Read file
while(true) {
symbolTable.init();
// gets a line from teh file no bigger than SIZE
fin.getline(line, SIZE);
if(!fin) {
break;
}
// converts the line into a stringstream so we can do things to it later as a string
stringstream in(line, ios_base::in);
// like removing parens
in >> paren;
cout << line << " ";
expression = SubExpression::parse(in);
in >> comma;
parseAssignments(in);
int result = expression->evaluate();
cout << "Value = " << result << endl;
}
/*
string program;
string input = "";
// Sample (( a + b) * c), a = 1 , b = 2, c = 3;
// (1 : 2 ? a > b), a = 1, b = 2;
cout << "Enter expression: ";
//((x < y) & ((x + y) = 10)), x = 2, y = 8;
// Get rid of leading paren
cin >> paren;
// This is what actually starts the expression tree.
expression = SubExpression::parse();
cin >> comma;
parseAssignments();
cout << "Value = " << expression->evaluate() << endl;
*/
return 0;
}
void parseAssignments(stringstream &in)
{
char assignop, delimiter, junk;
string variable;
int value;
// Clear out the symbol table
symbolTable.init();
do
{
variable = parseName(in);
in >> ws >> assignop >> value >> delimiter;
symbolTable.insert(variable, value);
}
while (delimiter == ',');
}
---------------------------------------------------------------------------------------------------------
//Subexpression.cpp
#include
using namespace std;
#include "expression.h"
#include "Subexpression.h"
#include "operand.h"
#include "plus.h"
#include "minus.h"
#include "times.h"
#include "divide.h"
#include "ge.h"
#include "geq.h"
#include "le.h"
#include "leq.h"
#include "eq.h"
#include "not.h"
#include "and.h"
#include "or.h"
#include "tern.h"
#include
#include
SubExpression::SubExpression(Expression* left, Expression* right)
{
this->left = left;
this->right = right;
}
Expression* SubExpression::parse(stringstream &in)
{
Expression* left;
Expression* right;
Expression *tern;
char operation, paren, junk;
left = Operand::parse(in); // x
in >> operation;// <
right = Operand::parse(in);// y
in >> ws;
if(in.peek() == '?') {
// get rid of question mark
in >> junk;
// get rid of paren
in >> paren;
tern = SubExpression::parse(in);
}
in >> paren;
switch (operation)
{
case '+':
return new Plus(left, right);
case '-':
return new Minus(left, right);
case '*':
return new Times(left, right);
case '/':
return new Divide(left, right);
// Hnadling in the event it's greater than or equal
case '>':
if(in.peek() == '=') {
in >> junk;// get rid of =
return new Geq(left, right);
}
else {
return new Ge(left, right);
}
// handling if it's less than or less than or equal too
case '<':
if(in.peek() == '=') {
in >> junk;// Get rid of =
return new Leq(left, right);
}
else {
return new Le(left, right);
}
case '=':
return new Eq(left, right);
case '&':
return new And(left, right);
case '|':
return new Or(left, right);
case '!':
return new Not(left, right);
case ':':
//tern = SubExpression::parse();
return new Tern(left, right, tern);
}
//f
return 0;
}
-------------------------------------------------------------------------------------
//Subexpression.h
#include
#include
class SubExpression: public Expression
{
public:
SubExpression(Expression* left, Expression* right);
static Expression* parse(stringstream &in);
protected:
Expression* left;
Expression* right;
};
-----------------------------------------------------------------------------------
//variable.cpp
#include
#include
using namespace std;
#include "expression.h"
#include "operand.h"
#include "variable.h"
#include "symboltable.h"
extern SymbolTable symbolTable;
int Variable::evaluate()
{
return symbolTable.lookUp(name);
}
----------------------------------------------------------------------------
//variable.h
class Variable: public Operand
{
public:
Variable(string name)
{
this->name = name;
}
int evaluate();
private:
string name;
};
-----------------------------------------------------------------------------
//SymbolTable.cpp
#include
#include
using namespace std;
#include "symboltable.h"
void SymbolTable::insert(string variable, int value)
{
const Symbol& symbol = Symbol(variable, value);
elements.push_back(symbol);
}
int SymbolTable::lookUp(string variable) const
{
for (int i = 0; i < elements.size(); i++)
if (elements[i].variable == variable)
return elements[i].value;
return -1;
}
void SymbolTable::init()
{
elements.clear();
}
--------------------------------------------------------------------------------------
//SymbolTable.h
class SymbolTable
{
public:
SymbolTable() {}
void insert(string variable, int value);
int lookUp(string variable) const;
void init();
private:
struct Symbol
{
Symbol(string variable, int value)
{
this->variable = variable;
this->value = value;
}
string variable;
int value;
};
vector
};
-------------------------------------------------------------------------------------
//parse.cpp
#include
#include
#include
using namespace std;
#include "parse.h"
#include
#include
string parseName(stringstream &in)
{
char alnum;
string name = "";
in >> ws;
while (isalnum(in.peek()))
{
in >> alnum;
name += alnum;
}
return name;
}
------------------------------------------------------------------------------------
//parse.h
#include
string parseName(stringstream &in);
-----------------------------------------------------------------------------------
//and.h
class And: public SubExpression
{
public:
And(Expression* left, Expression* right): SubExpression(left, right)
{
}
int evaluate()
{
if( (left->evaluate() && right->evaluate() > 0)) {
return 1;
}
return 0;
}
};
---------------------------------------------------------------------------------
//divide.h
class Divide : public SubExpression
{
public: Divide(Expression* left, Expression* right) : SubExpression(left, right){
}
int evaluate()// evaluates the expression
{
return left->evaluate() / right->evaluate();// if true will return the evaluate expression
}
};
--------------------------------------------------------------------------------
//eq.h
class Eq: public SubExpression
{
public:
Eq(Expression* left, Expression* right): SubExpression(left, right)
{
}
int evaluate()
{
const char *ltype = typeid(left).name();
const char *rtype = typeid(right).name();
if(std::strcmp(ltype, "Literal") > 0 && std::strcmp(rtype, "Literal") > 0)
{
if(left->evaluate() == right->evaluate()) {
return 1;
}
else {
return 0;
}
}
return 0;
}
};
--------------------------------------------------------------------------------------
//equalTo.h
#include "expression.h"
#include "Subexpression.h"
class EqualTo : public SubExpression
{
public:EqualTo(Expression* left, Expression* right) : SubExpression(left, right){
}
int evaluate()// evaluates the expression
{
if (left->evaluate() == right->evaluate())// if the left side of the expression equals the right side
{
return 1;// return 1. True!
}
else // else
{
return 0;// return 0. False!
}
}
};
----------------------------------------------------------------------------------------
//expression.h
class Expression
{
public:
virtual int evaluate() = 0;
};
-----------------------------------------------------------------------------------------
//ge.h
class Ge: public SubExpression
{
public:
Ge(Expression* left, Expression* right): SubExpression(left, right)
{
}
int evaluate()
{
// cin.peek() is = ?
if(left->evaluate() > right->evaluate()) {
return 1;
}
return 0;
}
};
-----------------------------------------------------------------------------------
//geq.h
class Geq: public SubExpression
{
public:
Geq(Expression* left, Expression* right): SubExpression(left, right)
{
}
int evaluate()
{
if(left->evaluate() >= right->evaluate()) {
return 1;
}
return 0;
}
};
--------------------------------------------------------------------------------
//greaterThan.h
#include "Subexpression.h"
#include "expression.h"
class GreaterThan : public SubExpression
{
public:GreaterThan(Expression* left, Expression* right) : SubExpression(left, right){
}
int evaluate()// use to evaluate the expression
{
if (left->evaluate() > right->evaluate())// if the left side of the expression is greater than the left side
{
return 1;// return 1, which is true!
}
else // else
{
return 0;// return 0, which is false!
}
}
};
-----------------------------------------------------------------------------------
//le.h
class Le: public SubExpression
{
public:
Le(Expression* left, Expression* right): SubExpression(left, right)
{
}
int evaluate()
{
if(left->evaluate() < right->evaluate()) {
return 1;
}
return 0;
}
};
--------------------------------------------------------------------------------
//leq.h
class Leq: public SubExpression
{
public:
Leq(Expression* left, Expression* right): SubExpression(left, right)
{
}
int evaluate()
{
if(left->evaluate() <= right->evaluate()) {
return 1;
}
return 0;
}
};
-------------------------------------------------------------------------------------
//lessThan.h
#include "Subexpression.h"
#include "expression.h"
class LessThan : public SubExpression
{
public: LessThan(Expression* left, Expression* right) : SubExpression(left, right){
}
int evaluate()// used to evaluate the expression
{
if (left->evaluate() < right->evaluate())// if the left side of expression is less than the right side
{
return 1;// return 1, which is true!
}
else // else
{
return 0;// return 0, which is false!
}
}
};
--------------------------------------------------------------------------------------------
//literal.h
class Literal: public Operand
{
public: Literal(int value)
{
this->value = value;
}
int evaluate()// used to evaluate the expression
{
return value;// returns the value of expression
}
private: int value;// value declared as data type integer
};
-----------------------------------------------------------------------------------------
//minus.h
class Minus : public SubExpression
{
public:Minus(Expression* left, Expression* right) : SubExpression(left, right){
}
int evaluate()// used to evaluate expression
{
return left->evaluate() - right->evaluate();// returns the value from subtracting the left side of the expression from the right side
}
};
---------------------------------------------------------------------------------------
//not.h
class Not: public SubExpression
{
public:
Not(Expression* left, Expression* right): SubExpression(left, right)
{
}
int evaluate()
{
if( ( (left->evaluate() > 0) || (right->evaluate() ) > 0)) {
return 0;
}
return 1;
}
};
------------------------------------------------------------------------------------
//operand.cpp
#include
#include
#include
#include
using namespace std;
#include "expression.h"
#include "Subexpression.h"
#include "operand.h"
#include "variable.h"
#include "literal.h"
#include "parse.h"
Expression* Operand::parse(stringstream &in)
{
char paren;
int value;
in >> ws;
if (isdigit(in.peek()))
{
in >> value;
Expression* literal = new Literal(value);
return literal;
}
if (in.peek() == '(')
{
in >> paren;
return SubExpression::parse(in);
}
else
return new Variable(parseName(in));
return 0;
}
------------------------------------------------------------------------------
//operand.h
#include
#include
class Operand: public Expression
{
public:
static Expression* parse(stringstream &in);
};
------------------------------------------------------------------------------
//or.h
class Or : public SubExpression
{
public: Or(Expression* left, Expression* right) : SubExpression(left, right){
}
int evaluate()// used to evaluate the expression
{
if (left->evaluate() != 0 || right->evaluate() != 0)// if left and right side of expression not equal to zero
{
return 1;// return 1, which is true!
}
else // else
{
return 0;// return 0, which is false!
}
}
};
----------------------------------------------------------------------------------
//plus.h
class Plus: public SubExpression
{
public: Plus(Expression* left, Expression* right): SubExpression(left, right){
}
int evaluate()// used to evaluate the expression
{
return left->evaluate() + right->evaluate();// adds the rgiht and left side fo the expression
}
};
-----------------------------------------------------------------------------------
//tern.h
#include
#include
// Follows the grammar '('
class Tern: public SubExpression
{
public:
Expression *tern;
char qmark;
Tern(Expression* left, Expression* right, Expression *tern): SubExpression(left, right)
{
// There is alwasy an expression after the ? so we need to get it
this->tern = tern;
}
int evaluate()
{
// upon creation get rid of ? and whitespace
//cin >> qmark;
// left = 1
int tvalue = left->evaluate();
// op = :
// right = 2
int fvalue = right->evaluate();
// left (a > b)
//newExp = SubExpression::parse();
// Ge object
//newExp = SubExpression::parse();
if(tern->evaluate() > 0) {
return tvalue;
}
else {
return fvalue;
}
}
};
------------------------------------------------------------------------------------------------
//ternary.h
#include "expression.h"
#include "Subexpression.h"
class Ternary : public SubExpression
{
public: Ternary(Expression* left, Expression* right, Expression* condition) : SubExpression(left, right)
{
this->condition = condition;
}
int evaluate()// used to evaluate expression
{
return condition->evaluate() ? left->evaluate() : right->evaluate();// returns left if true, else it returns the right
}
/** private method */
private: Expression* condition;// class Expression points to the variable condition
};
-------------------------------------------------------------------------------------
//times.h
class Times : public SubExpression
{
public:Times(Expression* left, Expression* right) : SubExpression(left, right){
}
int evaluate()// used to evaluate the expression
{
return left->evaluate() * right->evaluate();// returns the value of the left times the right side of the expression
}
}
testinputfiles:
Test1:
((x+y)/z), x = 1, y = 4, z = 6;
Test2:
(((x - (y*z)) = 6)!), x = 10, y = 4, z = 2;
Test3:
(20 : 40 ? ((x+y) > 10)), x = 4, y = 4;
Test4:
((x + 10) = 10 | ((x + 4) = 6)), x = 4;
Test5:
((x < y) & ((x + y) = 20)), x = 4, y = 16;
Test6:
(5 : 10 ? (a > b)), a = 2, b=3;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
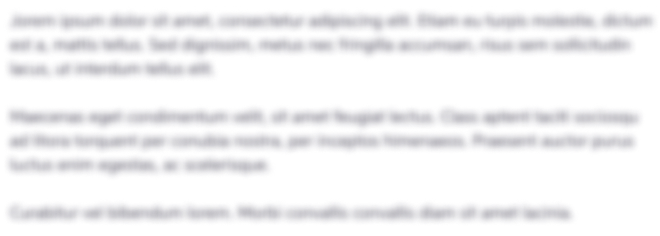
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started