Question
Could someone help me with this: implement adjustHeap( int index) from this class(percolate down) public class Heap extends Queue { private Vector _items = new
Could someone help me with this: implement adjustHeap(int index) from this class("percolate down")
public class Heap
@Override public T getFirst() { // TODO Auto-generated method stub return _items.getElementAt(0); }
@Override public void enqueue(T item) { // TODO Auto-generated method stub //Adds a new item to the heap //calculate positions //NOTE: in this MA, we STARTED AT 0!!!!! int current_position = _items.getSize(); int parent_position = (current_position - 1) /2; //insert element (note: may get erased if we hit the WHILE loop) //for simplicity purpose, in this MA, we are using the "swapping" method //i.e, insert the item first, then swap it all the way up _items.addElement(item); //get parent element if it exists T parent = null; if (parent_position >= 0) { parent = _items.getElementAt(parent_position); } if (parent != null) { //bubble up while (current_position > 0 && item.compareTo(parent) < 0) { _items.setElementAt(parent, current_position); current_position = parent_position; parent_position = (current_position - 1) / 2; if (parent_position >= 0) { parent = _items.getElementAt(parent_position); } } //after finding the correct location, we can finally place our item _items.setElementAt(item, current_position); } }
@Override public T dequeue() { // TODO Auto-generated method stub int last_position = _items.getSize() - 1; T last_item = _items.getElementAt(last_position); T top = _items.getElementAt(0); _items.setElementAt(last_item, 0); _items.removeElementAt(last_position); //percolate down after placing the last element in the root for simplicity purpose adjustHeap(0); return top; }
@Override public void addElement(T item) { // TODO Auto-generated method stub enqueue(item); }
@Override public boolean isEmpty() { // TODO Auto-generated method stub return _items.getSize() == 0; }
@Override public int getSize() { // TODO Auto-generated method stub return _items.getSize(); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
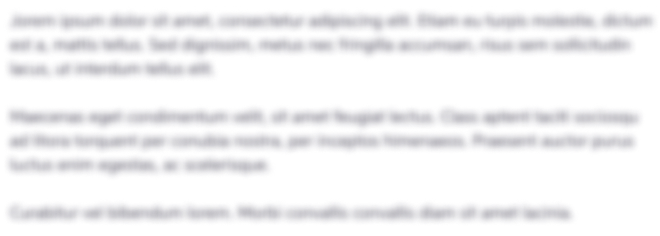
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started