Question
Could you help me fix my code to be more efficient and cleaner. The objective in this homework was to display all of the following
Could you help me fix my code to be more efficient and "cleaner." The objective in this homework was to display all of the following courses in the textfile once so that you are able take without repeating the prerequites again. I also ran to this error which I need help getting rid of:
Assignment #13(1571,0x1003b0380) malloc: *** error for object 0x10200f140: pointer being freed was not allocated
*** set a breakpoint in malloc_error_break to debug
//test.txt----------------------------------
2380 1370 calc1
1370 1101
3333 3310 2380
seniorproject softwareeng 3333
molecularcomputing 3333
calc2 calc1
calc3 calc2
diffeq calc3
automata 3310 3333 3332
3332 calc1
calc1 kidmath
kidmath
1101 ged
3310 1101
softwareeng 3333 3310
ged
//main.cpp-------------------------------------------------------------------------------------------------------------------------------------------
#include "graph.h"
#include "bst.h"
#include
#include
//#include
//#include
#include
#include
int main()
{
/* STEPS TO TAKE
1) Read in file.
2) Create directed graph
3) Per line...
-> 3.1) Tokenize word
-> 3.2) First word: Create a vertex (iff not inside graph already)
-> 3.3) 2+ word: Create vertex
-> 3.4) Add diredges from 2+ to first
STEPS TO TAKE */
digraph scheduler;
std::ifstream schedule;
schedule.open("test.txt");
// Scheduler
for (std::string line; std::getline(schedule, line); ) //line by line
{
//unsigned int count{ 0 };
std::stringstream ss(line);
std::string course, main;
for (int count = 0; ss >> course; count++)
{
//Input course
if (count == 0)
{
main = course;
if (scheduler.findVertex(course) == nullptr)
{
scheduler.addVertex(course); //Add first, main
}
}
else
{
if (scheduler.findVertex(course) == nullptr)
{
scheduler.addVertex(course); //Add first, main
}
scheduler.addDirectedEdge(course, main);
}
}
}
// Display
scheduler.displaySchedule();
return 0;
}
//bst.h---------------------------------------------------------------------------------------------
#ifndef bst_h
#define bst_h
#pragma once
#include
template
class binarySearchTree
{
private:
class node
{
public:
T data;
node * left;
node * right;
node(T x)
{
data = x;
left = nullptr;
right = nullptr;
}
};
//attributes?
node * root;
//insert x into tree rooted at p
void recInsert(node * &p, T x)
{
if (p == nullptr)
{
p = new node(x);
}
else
{
if (x->data < p->data->data) // if insertion is smaller
{
recInsert(p->left, x);
}
else // if insertion is greater or equal
{
recInsert(p->right, x);
}
}
}
void inOrder(node * p)
{
if (p == nullptr)
{
//nothing!
}
else
{
inOrder(p->left);
std::cout << p->data->data << ' ';
inOrder(p->right);
}
}
void recDisplayAllData(node * p)
{
if (p == nullptr)
{
return;
}
else
{
recDisplayAllData(p->left);
std::cout << "Course: " << p->data->data << " \t Pre-Requsites: ";
p->data->prereqs.display();
std::cout << ' ';
recDisplayAllData(p->right);
}
}
unsigned int count;
public:
binarySearchTree()
{
count = 0;
root = nullptr;
}
void insert(T x)
{
count++;
recInsert(root, x);
}
T recfindNode(node * p, std::string x)
{
if (p == nullptr)
{
return nullptr;
}
else if (p->data->data == x)
{
return p->data;
}
else
{
if (x < p->data->data)
{
return recfindNode(p->left, x);
}
else
{
return recfindNode(p->right, x);
}
}
}
T findVinN(std::string x)
{
return recfindNode(root, x);
}
void displayAllData()
{
recDisplayAllData(root);
}
bool empty()
{
if (count == 0)
{
return true;
}
return false;
}
void inOrderFinder(std::vector
{
if (p == nullptr)
{
return;
}
else
{
inOrderFinder(found, p->left);
if (p->data->prereqs.empty())
{
found.push_back(p->data);
}
inOrderFinder(found, p->right);
}
}
std::vector
{
std::vector
inOrderFinder(easyCourses, root);
return easyCourses;
}
void display()
{
inOrder(root);
}
};
#endif /* bst_h */ //graph.h--------------------------------------------------------------------------------------------------------------------------
#ifndef graph_h
#define graph_h
#pragma once
#include
#include
#include
#include
//#include
//#include
#include "bst.h"
/* NOTES
vertex: course
neighbors: what you can take after this vertex
visited: taken
prereqs: prerequisites for this vertex
NOTES */
class digraph
{
private:
class vertex
{
public:
std::string data;
std::vector
std::vector
bool visited;
vertex(std::string x)
{
data = x;
visited = false;
}
bool canTake()
{
for (int i = 0; i < prereqs.size(); i++)
{
if (prereqs[i]->visited == false) // If any pre-requisite has not been taken/visited. return can't take (false).
{
return false;
}
}
return true;
}
};
void bfs(vertex * p)
{
}
//std::vector
binarySearchTree
public:
digraph()
{
}
vertex * findVertex(std::string x) // O(log(|v|))
{
return masterList.findVinN(x);
}
void addVertex(std::string x) //add vertex into master masterList
{
masterList.insert(new vertex(x));
}
void addDirectedEdge(std::string x, std::string y) //X is prereq of Y O(log(n))
{
vertex * xVertex = findVertex(x);
vertex * yVertex = findVertex(y);
if (xVertex != nullptr && yVertex != nullptr)
{
//Made two for now to see how I would traverse, then will erase one if it isn't needed.
xVertex->neighbors.push_back(yVertex); // After X, you can take (can go to) Y (a neighbor)
yVertex->prereqs.push_back(xVertex); // X is a prerequisite of Y.
//std::cout << xVertex->data << " is a pre-requisite of " << yVertex->data << ' '; Test code.
}
else
{
std::cout << "You misspelled something. ";
}
}
void BFS(vertex * p)
{
std::queue
p->visited = true;
Q.push(p);
while (!Q.empty())
{
vertex * x = Q.front();
Q.pop();
std::cout << x->data << ' ';
for (int i = 0; i < x->neighbors.size(); i++) //check all of p's neighbors
{
if (x->neighbors[i]->canTake() && x->neighbors[i]->visited == false) //push em in if all prereqs taken
{
Q.push(x->neighbors[i]);
x->neighbors[i]->visited = true;
}
}
}
}
void displaySchedule()
{
// 1) Insert dummy vertex to connect any w/o prereqs. Didn't do this as default const since you won't know until after.
//DISPLAY DATA TEST
//masterList.displayAllData();
std::vector
addVertex("DUMMY CONNECTOR");
for (unsigned int i = 0; i < noPrereqs.size(); i++)
{
addDirectedEdge("DUMMY CONNECTOR", noPrereqs[i]->data);
}
noPrereqs.~vector();
//BFS FROM DUMMY CONNECTOR AND ONLY SHOW VERTEX WHERE PREREQS ARE ALL MARKED VISITED
BFS(findVertex("DUMMY CONNECTOR"));
}
};
#endif /* graph_h */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
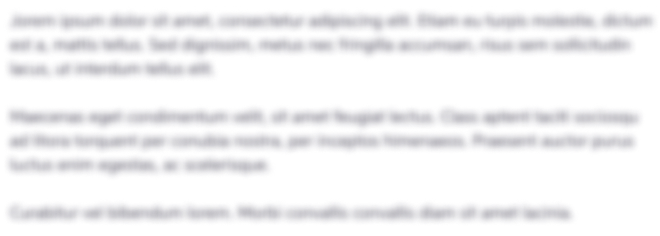
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started