Question
Could you split this Java code into multiple files? ```java import java.util.*; public class PokerGame { private static final String[] RANKS = { 2, 3,
Could you split this Java code into multiple files? ```java import java.util.*;
public class PokerGame {
private static final String[] RANKS = {
"2",
"3",
"4",
"5",
"6",
"7",
"8",
"9",
"10",
"Jack",
"Queen",
"King",
"Ace",
};
private static final String[] SUITS = { "", "", "", "" };
public static void main(String[] args) {
System.out.println("Welcome to the Five-Card Draw Poker Game!");
playGame();
}
private static void playGame() {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println(" Hand Rankings:");
System.out.println("1. Royal Flush");
System.out.println("2. Straight Flush");
System.out.println("3. Four of a Kind");
System.out.println("4. Full House");
System.out.println("5. Flush");
System.out.println("6. Straight");
System.out.println("7. Three of a Kind");
System.out.println("8. Two Pair");
System.out.println("9. One Pair");
System.out.println("10. High Card");
System.out.print(" Please enter the number of players (2-4): ");
int numPlayers = scanner.nextInt();
if (numPlayers < 2 || numPlayers > 4) {
System.out.println(
"Invalid number of players. Please enter a number between 2 and 4."
);
continue;
}
scanner.nextLine(); // Consume the newline character
String[] playerNames = new String[numPlayers];
for (int i = 0; i < numPlayers; i++) {
System.out.print("Enter Player " + (i + 1) + " username: ");
playerNames[i] = scanner.nextLine();
}
playRound(playerNames);
System.out.print(" Would you like to play again? (yes/no): ");
String playAgain = scanner.nextLine();
if (!playAgain.equalsIgnoreCase("yes")) {
break;
}
}
System.out.println("Thank you for playing!");
scanner.close();
}
private static void playRound(String[] playerNames) {
List
Collections.shuffle(deck);
Map
for (String playerName : playerNames) {
List
playerHands.put(playerName, hand);
}
System.out.println(" Shuffling the deck...");
System.out.println("Dealing the cards... ");
for (String playerName : playerNames) {
System.out.println("Each player receives 5 cards:");
System.out.println("Player " + playerName + ":");
List
printHand(hand);
}
for (String playerName : playerNames) {
System.out.println(" It's " + playerName + "'s turn.");
List
List
if (!cardsToChange.isEmpty()) {
changeCards(deck, hand, cardsToChange);
System.out.println(" " + playerName + "'s cards after changing:");
printHand(hand);
}
String highestRank = evaluateHand(hand);
System.out.println(
" " + playerName + "'s highest hand rank: " + highestRank
);
}
String winner = compareHands(playerHands);
System.out.println(" The winner is " + winner + "!");
}
private static List
List
for (String suit : SUITS) {
for (String rank : RANKS) {
deck.add(rank + " of " + suit);
}
}
return deck;
}
private static List
List
for (int i = 0; i < numCards; i++) {
hand.add(deck.remove(deck.size() - 1));
}
return hand;
}
private static void printHand(List
for (int i = 0; i < hand.size(); i++) {
System.out.println("[Card " + (i + 1) + "]: " + hand.get(i));
}
}
private static List
Scanner scanner = new Scanner(System.in);
System.out.println(
"Do you want to change any cards? Enter the numbers of the cards you want to change:"
);
for (int i = 0; i < hand.size(); i++) {
System.out.println((i + 1) + ". " + hand.get(i));
}
System.out.println(
"e.g. 1 2 3 4 5 -> Replace all cards 1 2 -> Replace Card 1 and 2"
);
List
String input = scanner
.nextLine()
.replaceAll(",", "")
.replaceAll("\\s+", "");
String[] cardIndices = input.split("");
for (String index : cardIndices) {
cardsToChange.add(Integer.parseInt(index) - 1);
}
return cardsToChange;
}
private static void changeCards(
List
List
List
) {
for (int index : cardsToChange) {
hand.set(index, deck.remove(deck.size() - 1));
}
}
private static String evaluateHand(List
if (isRoyalFlush(hand)) {
return "Royal Flush";
} else if (isStraightFlush(hand)) {
return "Straight Flush";
} else if (isFourOfAKind(hand)) {
return "Four of a Kind";
} else if (isFullHouse(hand)) {
return "Full House";
} else if (isFlush(hand)) {
return "Flush";
} else if (isStraight(hand)) {
return "Straight";
} else if (isThreeOfAKind(hand)) {
return "Three of a Kind";
} else if (isTwoPair(hand)) {
return "Two Pair";
} else if (isOnePair(hand)) {
return "One Pair";
} else {
return "High Card";
}
}
private static boolean isRoyalFlush(List
// Check if the hand is a Royal Flush
// A Royal Flush is a Straight Flush consisting of Ace, King, Queen, Jack, and 10 of the same suit
boolean isFlush = isFlush(hand);
boolean isStraight = isStraight(hand);
boolean hasAce = false;
boolean hasKing = false;
boolean hasQueen = false;
boolean hasJack = false;
boolean hasTen = false;
for (String card : hand) {
if (card.contains("Ace")) {
hasAce = true;
} else if (card.contains("King")) {
hasKing = true;
} else if (card.contains("Queen")) {
hasQueen = true;
} else if (card.contains("Jack")) {
hasJack = true;
} else if (card.contains("10")) {
hasTen = true;
}
}
return (
isFlush &&
isStraight &&
hasAce &&
hasKing &&
hasQueen &&
hasJack &&
hasTen
);
}
private static boolean isStraightFlush(List
// Check if the hand is a Straight Flush
// A Straight Flush is any sequence of five cards of the same suit
boolean isFlush = isFlush(hand);
boolean isStraight = isStraight(hand);
return isFlush && isStraight;
}
private static boolean isFourOfAKind(List
// Check if the hand is Four of a Kind
// Four of a Kind is four cards of the same rank, with one unmatched card
Map
for (String card : hand) {
String rank = card.split(" ")[0];
rankCounts.put(rank, rankCounts.getOrDefault(rank, 0) + 1);
}
for (int count : rankCounts.values()) {
if (count == 4) {
return true;
}
}
return false;
}
private static boolean isFullHouse(List
// Check if the hand is a Full House
// A Full House is three cards of the same rank and two cards of the same rank
Map
for (String card : hand) {
String rank = card.split(" ")[0];
rankCounts.put(rank, rankCounts.getOrDefault(rank, 0) + 1);
}
boolean hasThreeOfAKind = false;
boolean hasPair = false;
for (int count : rankCounts.values()) {
if (count == 3) {
hasThreeOfAKind = true;
} else if (count == 2) {
hasPair = true;
}
}
return hasThreeOfAKind && hasPair;
}
private static boolean isFlush(List
// Check if the hand is a Flush
// A Flush is any five cards of the same suit
String suit = hand.get(0).split(" ")[2];
for (String card : hand) {
if (!card.contains(suit)) {
return false;
}
}
return true;
}
private static boolean isStraight(List
// Check if the hand is a Straight
// A Straight is any sequence of five cards in consecutive ranks
List
for (String card : hand) {
String rank = card.split(" ")[0];
ranks.add(getRankValue(rank));
}
Collections.sort(ranks);
for (int i = 0; i < ranks.size() - 1; i++) {
if (ranks.get(i) + 1 != ranks.get(i + 1)) {
return false;
}
}
return true;
}
private static boolean isThreeOfAKind(List
// Check if the hand is Three of a Kind
// Three of a Kind is three cards of the same rank, with two unmatched cards
Map
for (String card : hand) {
String rank = card.split(" ")[0];
rankCounts.put(rank, rankCounts.getOrDefault(rank, 0) + 1);
}
for (int count : rankCounts.values()) {
if (count == 3) {
return true;
}
}
return false;
}
private static boolean isTwoPair(List
// Check if the hand is Two Pair
// Two Pair is two pairs of cards with the same rank, and one unmatched card
Map
int pairCount = 0;
for (String card : hand) {
String rank = card.split(" ")[0];
rankCounts.put(rank, rankCounts.getOrDefault(rank, 0) + 1);
}
for (int count : rankCounts.values()) {
if (count == 2) {
pairCount++;
}
}
return pairCount == 2;
}
private static boolean isOnePair(List
// Check if the hand is One Pair
// One Pair is a pair of cards with the same rank, and three unmatched cards
Map
for (String card : hand) {
String rank = card.split(" ")[0];
rankCounts.put(rank, rankCounts.getOrDefault(rank, 0) + 1);
}
for (int count : rankCounts.values()) {
if (count == 2) {
return true;
}
}
return false;
}
private static String compareHands(Map
String winner = "";
int maxRank = 0;
for (Map.Entry
String playerName = entry.getKey();
List
String handRank = evaluateHand(hand);
int rankValue = getRankValue(handRank);
if (rankValue > maxRank) {
maxRank = rankValue;
winner = playerName;
} else if (rankValue == maxRank) {
// If two players have the same hand rank, compare the highest cards in their hands
if (compareHighestCards(hand, playerHands.get(winner)) == 1) {
winner = playerName;
}
}
}
return winner;
}
private static int getRankValue(String handRank) {
switch (handRank) {
case "Royal Flush":
return 10;
case "Straight Flush":
return 9;
case "Four of a Kind":
return 8;
case "Full House":
return 7;
case "Flush":
return 6;
case "Straight":
return 5;
case "Three of a Kind":
return 4;
case "Two Pair":
return 3;
case "One Pair":
return 2;
default:
return 1; // High Card
}
}
private static int compareHighestCards(
List
List
) {
for (int i = hand1.size() - 1; i >= 0; i--) {
int rankComparison = compareCardRank(hand1.get(i), hand2.get(i));
if (rankComparison != 0) {
return rankComparison;
}
}
return 0; // Both hands have the same cards
}
private static int compareCardRank(String card1, String card2) {
int rank1 = getCardRank(card1);
int rank2 = getCardRank(card2);
if (rank1 > rank2) {
return 1;
} else if (rank1 < rank2) {
return -1;
} else {
return 0;
}
}
private static int getCardRank(String card) {
String rank = card.substring(0, card.indexOf(" "));
switch (rank) {
case "Ace":
return 14;
case "King":
return 13;
case "Queen":
return 12;
case "Jack":
return 11;
default:
return Integer.parseInt(rank);
}
}
}
```
Step by Step Solution
There are 3 Steps involved in it
Step: 1
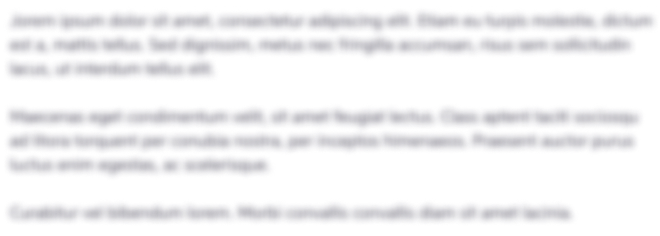
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started