Question
CPU Simulation we will simulate the execution of a CPU . The CPU will execute assembly instructions and will manage internal registers and a stack,
CPU Simulation
we will simulate the execution of a CPU . The CPU will execute assembly instructions and will manage internal registers and a stack, and external memory. The following diagram provides an outline of the data structure layout of the CPU:
Not shown in this diagram is an array of instruction objects that represent the assembly code. This will be a fixed array of length 200 and will be created and initialized when the CPU is created and is passed a fileName. The CPU will read in the file, parse each command line, create a corresponding instruction object, and store it into the instruction array.
The following table lists the opcodes the CPU will support:
OP Code | arg1 | arg2 | arg3 | Description |
ADD | Reg1 | Reg 2 | Takes the contents of the two registers and puts the sum into the first register listed | |
MULT | Reg1 | Reg2 | Takes the contents of the two registers and puts the product into the first register listed | |
STORE | Reg1 | Memory | Stores the contents of the register in memory location | |
FETCH | Reg1 | Memory | Get the contents of the memory location and store it in the register | |
EQU | Reg1 | Reg2 | Reg3 | Stores 1 in third register, Reg3, if the first two registers are equal, otherwise it stores a 0 into Reg3. |
PUSH | Reg1 | Push contents of the given register onto stack | ||
POP | Reg1 | Pop the top of the stack into the given register | ||
GOTO | Reg1 | Skip the number of instructions indicated in the register, note it can be a negative number | ||
LOAD | Reg1 | Value | Load the value into the given register | |
OUT | Reg1 | Display the contents of the given register | ||
IF | Reg1 | Reg2 | If register Reg1 is set to 1, skip the number of instructions specified in the second register, Reg2. Otherwise execute the next instruction. Note, this is different logic that we are used to. | |
DEBUG | Value | =1, display registers, =2 display memory, =3 display stack, =4 all 3 | ||
END | Ends the program |
Requirements:
The CPU will have internal data structures for the registers and the stack. It will run concurrently with other CPUs, will implement the Runnable interface, and will run within a Thread environment. Your simulator will support upto 4 CPUs running concurrently.
You will have an external memory module that will not be thread safe*. This will include a constructor which will specify how many memory locations your system will support. We will only support storing the integer data type. The Simulator should allocate each CPU its own memory array.
*NOTE: Since it takes 3 external assembly instructions to increment a value within memory, it is not possible to prevent simultaneous reads/writes to the same location with the techniques we discussed in class.
The CPU will have a 3 argument constructor specifying the fileName of the instruction commands, the cpu number, and a reference to the memory module. The CPU will read the file, parse the commands, create an instruction object, and then store them into an internal instruction array. The CPUs program counter (PC) will be the index to the instruction array.
The following diagram shows multiple CPUs running with a shared memory module:
Instruction file
The CPU will parse the instruction file consisting of 1 command per line. Your parser will ignore comments and blank lines. The following is an example of an instruction file:
LOAD R0, 1
LOAD R1, 2
LOAD R2, 3
LOAD R3, 4
LOAD R4, 5
OUT R0
DEBUG 1
END
Sample run
The following is a list of instructions with the expected output displayed in italics:
LOAD R0, 1
LOAD R1, 2
LOAD R2, 3
LOAD R3, 4
OUT R0
cpu: 1, reg: 0=1
OUT R1
cpu: 1, reg: 1=2
DEBUG 1
cpu: 1, registers: 1 2 3 4 0 pc=6
ADD R0, R1
MULT R2, R3
DEBUG 1
cpu: 1, registers: 3 2 12 4 0 pc=9
//test memory
LOAD R0, 1
LOAD R1, 2
LOAD R2, 3
LOAD R3, 4
LOAD R4, 5
STORE R0, 0
STORE R1, 5
STORE R2, 10
STORE R3, 20
STORE R4, 29
DEBUG 1
DEBUG 2
cpu: 1, registers: 1 2 3 4 5 pc=20
cpu: 1, memory:
1 0 0 0 0 2 0 0 0 0
3 0 0 0 0 0 0 0 0 0
4 0 0 0 0 0 0 0 0 5
FETCH R4, 0
FETCH R3, 5
FETCH R2, 10
FETCH R1, 20
FETCH R0, 29
DEBUG 1
cpu: 1, registers: 5 4 3 2 1 pc=27
//test stack
LOAD R0, 11
LOAD R1, 22
LOAD R2, 33
LOAD R3, 44
LOAD R4, 55
DEBUG 1
cpu: 1, registers: 11 22 33 44 55 pc=33
PUSH R0
PUSH R2
PUSH R1
PUSH R4
PUSH R3
DEBUG 3
cpu: 1, stack: 44 55 22 33 11
POP R0
POP R1
POP R2
POP R3
POP R4
DEBUG 1
cpu: 1, registers: 44 55 22 33 11 pc=45
DEBUG 4
cpu: 1, registers: 44 55 22 33 11 pc=46
cpu: 1, memory:
1 0 0 0 0 2 0 0 0 0
3 0 0 0 0 0 0 0 0 0
4 0 0 0 0 0 0 0 0 5
cpu: 1, stack:
END
//Loop, tests the IF statement
//Compute pow(R0, R1) ex: pow(2,6) = 64
LOAD R0, 2
LOAD R1, 6
//decrement mem(0) until == 0
//use R2 as the sum
LOAD R2, 1
DEBUG 1
//skip 6 instructions if done with loop: R1 == 0
LOAD R4, 0
EQU R1, R4, R3
LOAD R4, 6
IF R3, R4
MULT R2, R0
LOAD R3, -1
ADD R1, R3
LOAD R3, -8
GOTO R3
//End of loop
DEBUG 1
END
cpu: 1, registers: 2 0 64 1 6 pc=13
execution halted
Notes
Parsing the command line
|
Formatting output
For the toString() method, or when you want to format your output into a String before you display it, you can use the String.format( ) command. Here is an example of how you display the registers in the CPU class:
private String formatArray(int [] a) { String str = ""; for (int k=0; k { str += String.format("%4d ", a[k]); if ((k+1) % 10 == 0) str += " "; } return str; } private void dumpRegisters() { String str = "cpu: " + myNum + ", registers: "; str += formatArray(registers); str += " pc="+pc; System.out.println(str); } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
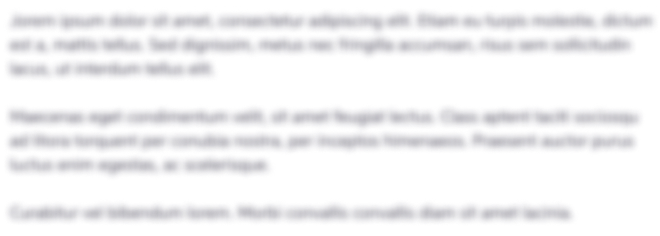
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started