Question
Create a binary search tree (you may choose to use the Graphics class in C# OR just display an inorder traversal of nodes as a
Create a binary search tree (you may choose to use the Graphics class in C# OR just display an inorder traversal of nodes as a console output) from a given array of numbers. For Example, if the given array is 11, 6, 4, 5, 8, 10, 19, 17, 43, 31, 49. You are supposed to program an inorder traversal displaying 4,5,6,8,10,11,17,19,31,43,49.
Design a program that: accepts any a sequence of numbers in an array (10 points) draws a binary search tree using the insertion method (30 points) o NOTE: insertion method uses a tree search method for locating the position of the new node. allows user to insert any new number as a new node in the BST (10 points) allows user to delete any existing node whose child nodes are internal nodes (30 points) after each insertion or deletion from the user, displays the inorder traversal of the tree (20 points)
Current Code (I know it is jumbled and all over the place, trying to get functional and then will clean it up.)
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace Binary_Search_Assignment { //Node Creation public class Node { public int data; public Node left, right; private object value;
public Node(int data) { this.data = data; left = right = null; }
public override string ToString() { return value.ToString(); }
}
//treeNode Creation public class TreeNode { object data; TreeNode left; TreeNode right; }
class BinaryTree
public class BNode
//Creating index value class Index { public int index = 0; }
class Program { //inputs public string inputNum; public string deleteNum; public double addNum; public double byeNum;
//This is where we will call each method within our program for testing static void Main(string[] args) { /*Console.WriteLine("What number would you like to insert?"); inputNum = Console.ReadLine; try { addNum = double.Parse(inputNum); } catch { Console.WriteLine("Error, No number entered!"); } insert();
Console.WriteLine("What number would you like to insert?"); deleteNum = Console.ReadLine; try { byeNum = double.Parse(deleteNum); } catch { Console.WriteLine("Error, No number entered!"); } delete();*/ //inOrderSort(); var tree = new BinaryTree(); int[] given = new int[] { 1, 7, 5, 50, 40, 10 }; int size = given.Length;
Node root = tree.constructTree(given, size);
Console.WriteLine("Inorder traversal of" + "the constructed tree:"); tree.printInorder(root);
//Console.WriteLine("sorted === " + bst); //search(); }
//method for insertion private static void insert() { }
//method for deletion private static void delete() { }
//method for sorting - Sheldon private static void inOrderSort() { if (v.left != null) { inOrderSort(leftNode, v); } return v.element; if (v.right != null) { inOrderSort(rightNode, v); } }
//method for searching private BNode
}
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
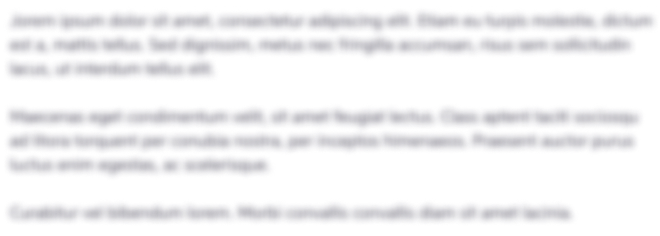
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started