Question
Create a C program which can disassemble this file. That is, print out assembly language source for this program. Explanation: Assembly language is a 1:1
Create a C program which can disassemble this file. That is, print out assembly language source for this program.
Explanation:
Assembly language is a 1:1 encoding between binary instructions and assembly source lines, with exceptions for certain derived pseudo-instructions which are assembled into more than one actual instruction. You dont need to worry about pseudo-instructions for this assignment, and may print out the instructions as encoded in memory. The RiSC-16 instruction set is ideal for this,
- All instructions are fixed size (16-bits, or two bytes)
- There are three instruction formats. All instructions will be in one of these three.
- The operation code (or opcode) is in the first 3 bits of the instruction in all formats
- There are only 8 instructions
The algorithm is as follows:
for each 16-bit word { extract the 3-bit opcode from the high-order 3 bits of the word determine the format based on the opcode extract the operands from the rest of the instruction format and print these values }
The essential skills that you need are:
- How to write a C program
- How to access command-line arguments in C
- How to read binary data from a file
- How to use C bitwise operators to extract fields from a multibit binary value
- How to format each instruction type
There are 8 opcodes for this processor which tell the processor which instruction to perform. Each has a unique 3-bit opcode (shown below in binary):
ADD: 000 | ADDI: 001 | NAND: 010 | LUI: 011 |
SW: 100 | LW: 100 | BEQ: 110 | JALR: 111 |
For this task you do not need to understand what these instructions do. The opcodes just correspond to short strings you will print out. Look up the string and print it out (along with operands described below).
There are 8 registers, numbered 0-7. They are encoded in binary: 000-111, and you should decode them as R0-R7.
Immediate values are any bit-pattern which fits in the field. RRI_type instructions take a signed 7-bit immediate value (-64 to 63). These values are small integers, such as one might use to increment/decrement a register:
ADDI R1, R1, 4 # Add 4 to R1
RI_type instructions take an unsigned 10-bit address offset (0-0x3FF) or (0-1023). These longer values are only used to refer to memory locations:
LUI, R1, 0x8000 # Create a mask with the high order bit set
Please decode the signed RRI values as decimal constants, and the unsigned RI values as hexadecimal constants (like 0x3FF).
3-bits | 3-bits | 3-bits | 4-bits | 3-bits | |
RRR_type | Opcode | regA | regB | 0 | regC |
RRI_type | Opcode | regA | regB | Signed immediate | |
RI_type | Opcode | regA | Unsigned immediate |
Youll probably want to create a table of structures to hold the opcodes, the mnemonics, and the format. For example: opcode 000 has mnemonic ADD, and is an RRR_type instruction. You would initialize this table with values from the Instruction Set document.
After you read a 16-bit value from a file, you figure out what the leading 3 bits are, then lookup the opcode in your table. Youll format the remainder of the word differently, depending on the format.
Do not begin output in the first column. Start each line with a tab, so that labels stand out. You will not be writing labels in this assignment.
Sample output:
ADD R1, R0, R0 LW R5, R1, 18 ADD R5, R5, R3 SW R5, R1, 20
The binary (from the input file) would be:
0000010000000000 # 0x0400 1011010010010010 # 0xB692 0001011010000011 # 0x1483 1001010010010100 # 0x9494
You will be reading 16-bit unsigned integers from the binary file (such as the hex values in the second column), and producing text similar to the sample output.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
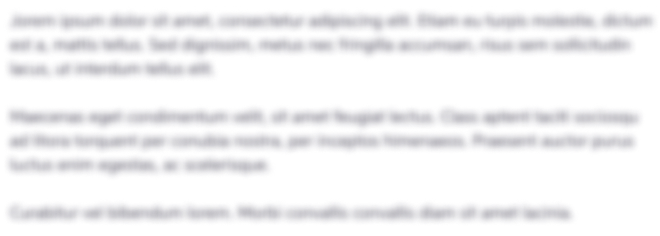
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started