Question
Create a C project named login _l06t4. Add a function named move_front_to_rear to the queue definition. This function should move a node (not the data!)
Create a C project named login_l06t4. Add a function named move_front_to_rear to the queue definition. This function should move a node (not the data!) from the front of a source queue to the rear of a target queue. The function may not call the enqueue or dequeue functions. The prototype is:
void move_front_to_rear(queue *target, queue *source);
If the source queue is empty, print an error message. Make sure that the front and rear pointers are properly updated!
Test this function by creating two queues and move two nodes of one to the rear of the other. Test again with a target queue that is not empty. Does your function properly update both the front and rear pointers as necessary? Print the contents of the queues before and after the move.
///////////////////////////////////////////
The Queue Definition
/* * HBF */ #include#include typedef struct node { int data; struct node *next; } qnode; typedef struct queue { qnode *front; qnode *rear; } queue; void enqueue(queue *, int); int dequeue(queue *); qnode *dequeue1(queue *); void dequeue2(queue *, qnode**); void display(queue); void clean(queue *); int main() { int i, val; queue myq = {0}; myq.front = NULL; myq.rear = NULL; for (i=1; i<=5; i++) { printf("Enqueue value:%d ", i); enqueue(&myq, i); } printf(" Display all: "); display(myq); printf(" Dequeue value: %d ", dequeue(&myq)); qnode *qnp = dequeue1(&myq); printf(" Dequeue1: %d ", qnp->data); free(qnp); dequeue2(&myq, &qnp); printf(" Dequeue2: %d ", qnp->data); free(qnp); printf(" Display all: "); display(myq); clean(&myq); return 0; } void enqueue(queue *q, int val) { qnode *ptr; ptr = (qnode*) malloc(sizeof(qnode)); ptr->data = val; if (q->front == NULL) { q->front = ptr; q->rear = ptr; q->front->next = q->rear->next = NULL; } else { q->rear->next = ptr; q->rear = ptr; q->rear->next = NULL; } } int dequeue(queue *q) { int val = -1; if (q->front == NULL) printf(" UNDERFLOW"); else { qnode *ptr = q->front; val = ptr->data; q->front = ptr->next; free(ptr); } return val; } qnode *dequeue1(queue *q) { qnode *ptr = NULL; if (q->front) { ptr = q->front; if (q->front == q->rear) { q->front = NULL; q->rear = NULL; } else { q->front = ptr->next; } } return ptr; } void dequeue2(queue *q, qnode **npp) { qnode *ptr = NULL; if (q->front) { ptr = q->front; if (q->front == q->rear) { q->front = NULL; q->rear = NULL; } else { q->front = ptr->next; } } *npp = ptr; } int peek(queue q) { if (q.front == NULL) { printf(" QUEUE IS EMPTY"); return -1; } else return q.front->data; } void display(queue p) { qnode *ptr = p.front; if (ptr == NULL) printf(" QUEUE IS EMPTY"); else { while (ptr != p.rear) { printf("%d\t", ptr->data); ptr = ptr->next; } printf("%d\t", ptr->data); } } void clean(queue *q) { if (q->front != NULL) { qnode *temp, *ptr = q->front; while (ptr != NULL) { temp = ptr; ptr = ptr->next; free(temp); } q->front = NULL; q->rear = NULL; } } /* Enqueue value:1 Enqueue value:2 Enqueue value:3 Enqueue value:4 Enqueue value:5 Display all: 1 2 3 4 5 Dequeue value: 1 Dequeue1: 2 Dequeue2: 3 Display all: 4 5 */
Step by Step Solution
There are 3 Steps involved in it
Step: 1
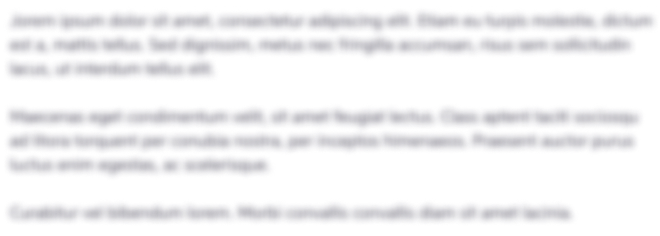
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started