Question
Create a C++ project with 2 classes, Person and Birthdate. The description for each class is shown below: Birthdate class: private members: year, month and
Create a C++ project with 2 classes, Person and Birthdate. The description for each class is shown below:
Birthdate class:
private members: year, month and day
public members: copy constructor, 3 arguments constructor, destructor, setters, getters and age (this function should return the age)
Person class:
private members: firstName, lastName, dateOfBirth, SSN
public members: 4 arguments constructor (firstName, lastName, datOfBirth and SNN), destructor and printout
Implementation:
- use the separated files approach
- implement all the methods for the 2 classes
- make sure to use the member initialization list
create 3 objects and test the implemented methods.
Using the files:
BirthDate.h:
#ifndef BIRTHDATE_H
#define BIRTHDATE_H
#include
using namespace std;
class birthDate{
private:
int year, month, day;
public:
birthDate(birthDate&);
birthDate(int, int, int);
~birthDate(){}
void setYear(int);
void setMonth(int);
void setDay(int);
int getYear();
int getMonth();
int getDay();
int age();
};
#endif
Person.h
#ifndef PERSON_H
#define PERSON_H
#include "birthDate.h"
#include
class Person{
private:
string firstName, lastName;
birthDate DOB;
int SSN;
public:
Person(string, string, birthDate, int);
~Person(){}
void printout();
};
#endif
BirthDate.cpp:
#include "birthDate.h"
Person.cpp:
#include"Person.h"
Source.cpp:
#include"Person.h"
void main(){
system("pause");
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
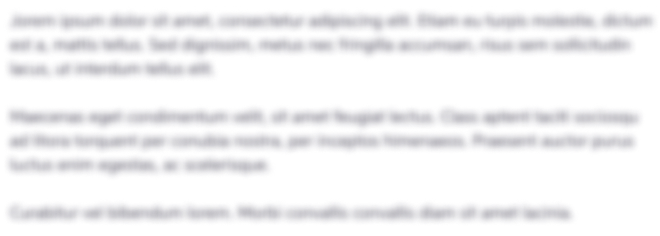
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started