Question
Create a class called DateNode which has fields for the data (a Date212) and next (DateNode) instance variables. Include a one-argument constructor which takes a
Create a class called DateNode which has fields for the data (a Date212) and next (DateNode) instance variables. Include a one-argument constructor which takes a Date212 as a parameter.
public DateNode (Date212 d) { . . }
The instance variables should have protected access. There will not be any get and set methods for the two instance variables.
Create an abstract linked list class called DateList. This should be a linked list with head node. Modify it so that the data type in the nodes is Date212. The no-argument constructor should create an empty list with first and last pointing to an empty head node, and length equal to zero. Include an append method in this class.
Create two more linked list classes that extend the abstract class DataList: One called UnsortedDateList and one called SortedDateList, each with appropriate no-argument constructors. Each of these classes should have a method called add(Date212) that will add a new node to the list. In the case of the UnsortedDateList it will add it to the end of the list by calling the append method in the super class. In the case of the SortedDateList it will insert the node in the proper position to keep the list sorted.
Instantiate two linked lists, and for every date read from the file, add it to the unsorted and sorted lists using the add method. You will end up with the first list having the dates from the input file in the order they were read, and in the second list the dates will be in sorted order. Display the unsorted and sorted dates in the GUI just as in project 1.
Create a GUI Add a file menu to your DateGUI with options to open a file for reading, and one to Quit the program.
This project is a modification of a previous one which contains Project1, which should be made into Project2, Date212, and DateGUI, which I will provide. These 3 classes also used an additional class TextFileInput to read the text file, which I will also provide.
Text file: Date.txt
20141001 20080912,20131120,19980927 20020202 20120104
So there should be a total of 7 classes in all:
Project2.java Date212.java DateGUI.java DateNode.java DateList.java UnsortedDateList.java SortedDateList.java
(additionally TextFileInput.java if used.)
_______________________________________________________________________________________
/* Project1.java */
/*Project1 reads dates from * a textfile, sorts and prints * those dates in both sorted * and unsorted format to a GUI. */ import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; import java.util.StringTokenizer; public class Project1 { static final int LIST_SIZE = 100; static TextFileInput inFile; static DateGUI myDateGUI; /*create two Date212 arrays of List size * to store unsorted information from textfile * and the sorted processed information. */ static Date212[] unsorted = new Date212[LIST_SIZE]; static Date212[] sorted = new Date212[LIST_SIZE];
public static void main(String[] args) {
myDateGUI = new DateGUI("Date List", 500, 300); Date212[] dataSource = new Date212[LIST_SIZE]; int numDate = readDatesFromFile("Date.txt", dataSource);
/*Takes the raw information * from the textfile and sorted * information from the format in * Date212 and prints it to GUI columns. */ myDateGUI.unsortedDates.append(getList(dataSource,numDate)); selectionSort(dataSource,numDate); myDateGUI.sortedDates.append(getListAsString(dataSource,numDate)); }
private static String getList(Date212[] dataSource, int numDate) { String datelist=""; for (int i = 0; i < numDate; i++) { datelist+=dataSource[i].toString()+" "; } return datelist; } /* * This method takes input from class Date212 * and returns string representation of Date212 * to be printed to GUI. */ public static String getListAsString(Date212[] dates,int count){ String datelist=""; for (int i = 0; i < count; i++) { datelist+=dates[i].getAsString()+" "; } return datelist; }
/* * This method reads data from file myFile into dateList * and returns the number of dates as integer values. */ public static int readDatesFromFile(String myFile, Date212[] dateList) { Scanner filescanner=null; int count=0; try {
filescanner=new Scanner(new File(myFile)); String line; /* read until no line is left */ while (filescanner.hasNextLine()) { line = filescanner.nextLine(); /* Tokenize the line and get date. * Necessary to create a line break every time * a comma(,) occurs. Whatever is after a comma * is printed to a new line */ StringTokenizer tokens = new StringTokenizer(line, ","); while (tokens.hasMoreTokens()) { String inStr = tokens.nextToken(); { Date212 date = new Date212(inStr); dateList[count]=date; } count++; } }
} catch (FileNotFoundException e) { System.out.println(e.getMessage()); } return count;
}
private static void swap(Date212[] a, int x, int y) { //helper method for the 'selectionSort' method below: Date212 temp; temp = a[x]; a[x] = a[y]; a[y] = temp; } /*Selection sort used to sort * the dates in ascending order */ public static void selectionSort(Date212[] array, int length) { for (int i = 0; i < length - 1; i++) { int indexLowest = i; for (int j = i + 1; j < length; j++) { if (array[j].compareTo(array[indexLowest]) < 0) { indexLowest = j; if (array[indexLowest].compareTo(array[i]) < 0) swap(array, indexLowest, i); } } } } }
_____________________________________________________________________________________________________________________________________
/* Date212.java
*/
/*Class Date212 converts the
* contents of the textfile
* read from class Project1 to
* the required format.
*/
class Date212
{
/*Establishes the names of the months
*that the number representations of
*the months will be converted to.
*/
private String monthsInYear[]=
{"","January","February",
"March","April","May",
"June","July","August",
"September","October",
"November","December"};
/*All variables set to
* private to prevent
* user tampering.
*/
private int month;
private String monthString; //Needed to convert the number representation of month to the name of the month.
private int day;
private int year;
/*Assigns variables year,
* month, and day.
*/
public Date212 (int y, int m, int d) {
year=y;
month=m;
day=d;
monthString = monthsInYear[m];
}
/*Gets and sets the information
* from the day, month, and year
* sections of the string respectively.
*/
public int getDay() {
return day;
}
public int getMonth() {
return month;
}
public int getYear() {
return year;
}
public void setDay(int d) {
day=d;
}
public void setMonth(int m) {
month=m;
}
public void setYear(int y) {
year=y;
}
public Date212 (String d)
{
/*Calling three argument constructor
* Substring reads the data from the textfile
* and separates them, with years being the first
* 4 numbers, months being the fifth and sixth numbers,
* and days being the seventh and eighth numbers.
*/
this(Integer.parseInt(d.substring(0, 4)), Integer.parseInt(d.substring(4, 6)), Integer.parseInt(d.substring(6, 8)));
}
//Method that returns date in the required format mm dd, yyyy
public String getAsString()
{
return monthString + " " + String.format("%02d", day) + ", " + String.format("%04d", year);
}
/*
* Returns the string representation of the Date212 object
*/
public String toString() {
String result = "";
result += + this.year;
result += this.month < 10 ? "0" + this.month : this.month;
result += this.day < 10 ? "0" + this.day : this.day;
return result;
}
/*
* Override compareTo method
* Comparision of date objects.
* Compares date values to find
* out which is larger for
* sorting
*/
public int compareTo(Date212 other) {
if (this.year < other.year) {
return -1;
} else if (this.year > other.year) {
return 1;
}
if (this.month < other.month) {
return -1;
} else if (this.month > other.month) {
return 1;
}
if (this.day < other.day) {
return -1;
} else if (this.day > other.day) {
return 1;
}
return 0;
}
}
__________________________________________________________________________________________________________________________________
/* DateGUI.java
*/
/*Class DateGUI creates the
* GUI to which the sorted
* and unsorted dates will
* be printed.
*/
import java.awt.GridLayout;
import java.awt.TextArea;
import javax.swing.*;
public class DateGUI extends JFrame
{
/**
*
*/
private static final long serialVersionUID = 1L;
//declare TextArea class
TextArea unsortedDates, sortedDates;
public DateGUI(String title, int height, int width) {
setSize(400, 400); // set the size of the JFrame
setLocation(100, 100); // set the location of the JFrame
setTitle("Project 1"); // set the title of the JFrame
setLayout(new GridLayout(1, 2)); // Creates the 1 row, 2 column layout
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
unsortedDates = new TextArea();//Allows the unsorted text to be displayed on the left column.
sortedDates = new TextArea();//Allows the sorted text to be displayed on the right column.
getContentPane().add(unsortedDates); //Used to create left column in GUI
getContentPane().add(sortedDates); //Used to create right column in GUI
setVisible(true);
}
}
____________________________________________________________________________________________________________________________________
// TextFileInput.java // Copyright (c) 2000, 2005 Dorothy L. Nixon. All rights reserved.
import java.io.BufferedReader; import java.io.FileInputStream; import java.io.InputStreamReader; import java.io.IOException;
/** * Simplified buffered character input * stream from an input text file. * Manages an input text file, * handling all IOExceptions by generating * RuntimeExcpetions (run-time error * messages). * * If the text file cannot be created, * a RuntimeException is thrown, * which by default results an an * error message being printed to * the standard error stream. * * @author D. Nixon */ public class TextFileInput {
/** Name of text file */ private String filename;
/** Buffered character stream from file */ private BufferedReader br;
/** Count of lines read so far. */ private int lineCount = 0;
/** * Creates a buffered character input * strea, for the specified text file. * * @param filename the input text file. * @exception RuntimeException if an * IOException is thrown when * attempting to open the file. */ public TextFileInput(String filename) { this.filename = filename; try { br = new BufferedReader( new InputStreamReader( new FileInputStream(filename))); } catch ( IOException ioe ) { throw new RuntimeException(ioe); } // catch } // constructor
/** * Closes this character input stream. * No more characters can be read from * this TextFileInput once it is closed. * @exception NullPointerException if * the file is already closed. * @exception RuntimeException if an * IOException is thrown when * closing the file. */ public void close() { try { br.close(); br = null; } catch ( NullPointerException npe ) { throw new NullPointerException( filename + "already closed."); } catch ( IOException ioe ) { throw new RuntimeException(ioe); } // catch } // method close
/** * Reads a line of text from the file and * positions cursor at 0 for the next line. * Reads from the current cursor position * to end of line. * Implementation does not invoke read. * * @return the line of text, with * end-of-line marker deleted. * @exception RuntimeException if an * IOException is thrown when * attempting to read from the file. */ public String readLine() { return readLineOriginal(); } // method readLine()
/** * Returns a count of lines * read from the file so far. */ public int getLineCount() { return lineCount; }
/** * Tests whether the specified character is equal, * ignoring case, to one of the specified options. * * @param toBeChecked the character to be tested. * @param options a set of characters * @return true if toBeChecked
is * equal, ignoring case, to one of the * options
, false otherwise. */ public static boolean isOneOf(char toBeChecked, char[] options) { boolean oneOf = false; for ( int i = 0; i < options.length && !oneOf; i++ ) if ( Character.toUpperCase(toBeChecked) == Character.toUpperCase(options[i]) ) oneOf = true; return oneOf; } // method isOneOf(char, char[])
/** * Tests whether the specified string is one of the * specified options. Checks whether the string * contains the same sequence of characters (ignoring * case) as one of the specified options. * * @param toBeChecked the String to be tested * @param options a set of Strings * @return true if toBeChecked
* contains the same sequence of * characters, ignoring case, as one of the * options
, false otherwise. */ public static boolean isOneOf(String toBeChecked, String[] options) { boolean oneOf = false; for ( int i = 0; i < options.length && !oneOf; i++ ) if ( toBeChecked.equalsIgnoreCase(options[i]) ) oneOf = true; return oneOf; } // method isOneOf(String, String[]) /** * Reads a line from the text file and ensures that * it matches one of a specified set of options. * * @param options array of permitted replies * * @return the line of text, if it contains the same * sequence of characters (ignoring case for * letters) as one of the specified options, * null otherwise. * @exception RuntimeException if the line of text * does not match any of the specified options, * or if an IOException is thrown when reading * from the file. * @exception NullPointerException if no options are * provided, or if the end of the file has been * reached. */ public String readSelection(String[] options) { if ( options == null || options.length == 0 ) throw new NullPointerException( "No options provided for " + " selection to be read in file " + filename + ", line " + (lineCount + 1) + ".");
String answer = readLine();
if ( answer == null ) throw new NullPointerException( "End of file " + filename + "has been reached.");
if ( !TextFileInput.isOneOf(answer, options) ) { String optionString = options[0]; for ( int i = 1; i < options.length; i++ ) optionString += ", " + options[i]; throw new RuntimeException("File " + filename + ", line " + lineCount + ": \"" + answer + "\" not one of " + optionString + "."); } // if return answer; } // method readSelection
/** * Reads a line from the text file and ensures that * it matches, ignoring case, one of "Y", "N", "yes", * "no", "1", "0", "T", "F", "true", or "false". * There must be no additional characters on the line. * * @return true
if the line matches * "Y", "yes", "1" "T", or "true". * false
if the line matches * "N", "no", "0", "F", or "false". * @exception RuntimeException if the line of text * does not match one of "Y", "N", "yes", * "no", "1", "0", "T", "F", "true", or "false", * or if an IOException is thrown when reading * from the file. * @exception NullPointerException if the end of the * file has been reached. */ public boolean readBooleanSelection() { String[] options = {"Y", "N", "yes", "no", "1", "0", "T", "F", "true", "false"}; String answer = readSelection(options); return isOneOf(answer, new String[] {"Y", "yes", "1", "T", "true"} ); } // method askUserYesNo
/** * Reads a line of text from the file and * increments line count. (This method * is called by public readLine and is * final to facilitate avoidance of side * effects when public readLine is overridden.) * * @return the line of text, with * end-of-line marker deleted. * @exception RuntimeException if an * IOException is thrown when * attempting to read from the file. */ protected final String readLineOriginal() { try { if ( br == null ) throw new RuntimeException( "Cannot read from closed file " + filename + "."); String line = br.readLine(); if ( line != null ) lineCount++; return line; } catch (IOException ioe) { throw new RuntimeException(ioe); } // catch } // method readLineOriginal } // class TextFileInput
Step by Step Solution
There are 3 Steps involved in it
Step: 1
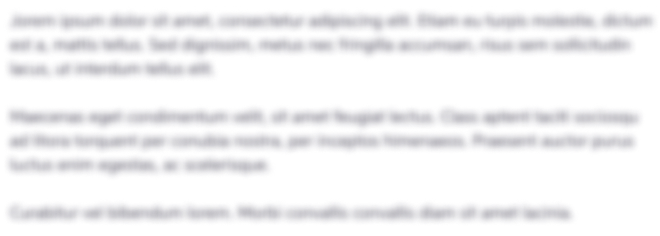
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started