Question
create a Coin class with instance variables private String name (like nickel), private double value (like .05), and private double weight (like 5 grams) and
create a Coin class with instance variables private String name (like nickel), private double value (like .05), and private double weight (like 5 grams) and a 3-parameter constructor to set all of them, and have that class implement Comparable:
The class has the single constructor mentioned above plus setters for all three instance variables; you can use those setters in the constructor if you want.
Also write a toString method whose String contains all three instance variables and returns this String, rounding the value and weight to 3 decimal places:
To implement Comparable, define a public int compareTo(Object other) method that works as follows:
It uses instanceof to check if Object other is a Coin; if not, it returns 1.
It uses downcasting to assign other to a new Coin reference variable coin.
It returns Strings compareTo result if not 0; if 0, it returns a result comparing the value of the two Coins; if they have the samevalue, it returns a result comparing the weight of the two Coins.
Write a main method that creates a small Coin[] (say, length 5) and fill it with Coin objects that are not ordered, then run Arrays.sort on the array and finally print the Coin objects from the array to see if they have been sorted properly based on the implementation of Comparable.
import java.util.*;
public class Coin implements Comparable { /* declare the 3 instance variables here */
/* write the 3-parameter Coin constructor here; you can use the setters below to set the instance variables */
/* write the 3 setters for the instance variables here */
@Override public String toString() { /* write the toString method here as required above */ }
@Override public int compareTo(Object other) { /* write the compareTo method here as required above */ }
public static void main(String[] args) { /* DO NOT MODIFY THIS MAIN METHOD */ Coin[] coins = new Coin[5]; coins[0] = new Coin("nickel", .05, 5); coins[1] = new Coin("penny", .011, 4); coins[2] = new Coin("penny", .01, 4); coins[3] = new Coin("nickel", .05, 5.1); coins[4] = new Coin("dime", .10, 3.5);
System.out.println("Coins before sorting:"); for (Coin coin : coins) System.out.println(coin); Arrays.sort(coins); System.out.println(); System.out.println("Coins after sorting:"); for (Coin coin : coins) System.out.println(coin); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
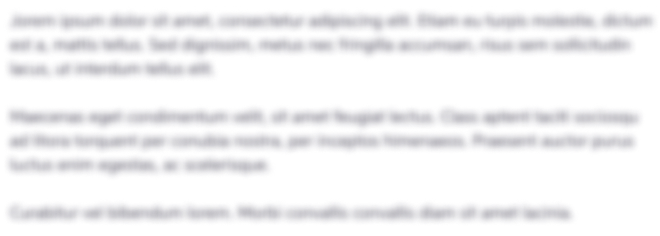
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started