Question
Create a constructor for each class that takes all the fields Create additional overloaded constructors where it makes sense to do so Create getters for
Create a constructor for each class that takes all the fields
Create additional overloaded constructors where it makes sense to do so
Create getters for all fields.
Create setters for fields where it makes sense to do so
Create the additional specified class methods
Use the project and file names specified
Mini-lecture:
Last week, we reviewed how to save and read data records to and from a CSV text file. This week we are looking at how to create objects in java, so we will create java classes that correspond to our data records, Person and Product. We will modify the programs from the previous lab to use our new object classes.
Part 1: Person:
Project: Person Files: Person.java PersonReader.java // Reads Person records from a file into an ArrayList PersonGenerator.java // Creates an ArrayList of Person objects and writes to a file SafeInput.java // Library of console input routines PersonTest.java // Junit test file for Person class
Fields: (No change here from the Practicum)
String firstName String lastName String ID // should never change sequence of digits String title // a prefix: Mr. Mrs. Ms, Prof. Dr. Hon. Etc. int YOB // Year of birth // Range should be 1940 - 2000
Additional methods (All should be tested in JUnit):
public String fullName() // returns firstName, space, lastName public String formalName() // returns title, space, fullName
public String getAge() // returns the age assuming the current year public String getAge(int year) // uses YOB to calculate age for a specified year // use the Calendar object to do these. Requires a bit of a web search.
Public String toCSVDataRecord() // returns a comma separated value (csv) String suitable to writing to a java text file. Be sure to sue this function when you save data to the file. You can used the for each loop to traverse the ArrayList and use this function to generate the CSV record to write.
When you read the data in from the CSV text file, instantiate a Person object from each record and store the Person objects within an ArrayList of type
Make sure that your program uses all the methods here.
Create the new class files first (i.e. Person for part 1 and Product for part 2) Create the JUnit test for the class at that time. IntelliJ will stub out the tests. You have to construct tests instances in setup to use in the tests and fill out the stubs. Test the constructors, all modifier/setter methods, and any additional special methods. You do not test the getter/read methods. Then modify the java main code that your forked. That is, make sure you fork the code before you change the code in main. So you will have a working version of the last lab and a new fork which is this lab.
In the Generator, as soon as the user inputs the field data, instantiate an object that contains that data and save it in an arrayList of either Product or Person. After you have all the data input, write it to disk from the arrayList using the toCSVRecordMethod you implemented. In the Reader, as you read a line of the data file use the java String split function to separate the fields of this single record into an array. Then use the array data to create an object and add it to the arrayList. Your table of Person or Product data should be generated from the ArrayList.
****QUESTION****
I have already started the problem but im getting confused as to why its not working. I will be attaching my code but I also need a visual of the code back for a answer because Im getting confused on what pieces of code should be placed where. I greatly appreciate the help. Also if you could explain
//Person Generator
//Person Reader
//Getters and setters
Step by Step Solution
There are 3 Steps involved in it
Step: 1
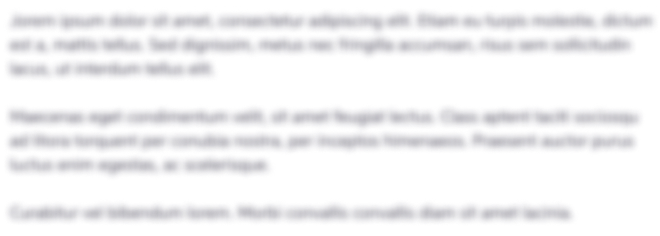
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started