Question
create a file in the problems directory named problem6.hpp . In that file, write a C++ function template called combine that takes six parameters: The
create a file in theproblemsdirectory namedproblem6.hpp. In that file, write a C++ function template calledcombinethat takes six parameters:
- The first and second parameters are a pair of begin/end iterators, which you can assume are forward iterators that need to be read from, representing a sequence of input values.
- The third and fourth parameters are another pair of begin/end iterators, which you can assume are forward iterators that also need to be read from, representing another sequence of input values.
- The fifth parameter is a forward iterator representing the starting point where output will be written.
- The sixth parameter is any kind of C++ function object that takes an element from the first sequence and an element from the second sequence, combining their values in whatever way it wants.
The function returns no value, but it writes the result of combining each element from the first and second sequences by calling the given function object. A couple of examples of usage follow.
std::vector<int> v1{1, 3, 5, 7, 9}; std::list<int> list1{2, 4, 6, 8, 10}; std::vector<int> v2(5, 0); std::list<int> list2; combine( v1.begin(), v1.end(), list1.begin(), list1.end(), v2.begin(), [](int a, int b) { return a + b; }); // At this point, v2 will contain five elements: 3, 7, 11, 15, 19 combine( list1.begin(), list1.end(), v1.begin(), v1.end(), std::back_inserter(list2), [](int a, int b) { return a * b; }); // At this point, list2 will contain five elements: 2, 12, 30, 56, 90
(Note thatstd::back_inserterreturns an iterator that adds elements to the end of the data structure, rather than writing into an existing position already in the data structure.)
Combining values stops when one of the two iterator ranges (either the range specified by the first and second parameters or the range specified by the third and fourth parameters) runs out of elements, so that the result of combining, say, a vector of three elements and a list of five elements would be to have three elements written to the output iterator.
The function template can take as many template parameters as you think are necessary to solve the problem. Impose as few constraints on the template's parameters as possible; any two pairs of input iterators should be allowed and any output iterator should be allowed, as long as they're forward iterators
Step by Step Solution
There are 3 Steps involved in it
Step: 1
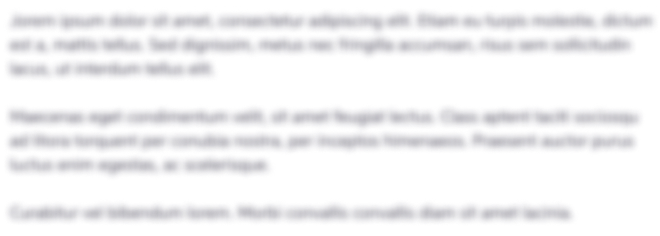
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started