Question
Create a GUI for your game. You are free in designing it the way you like. Must be able to: 1 vs 1 or 2
Create a GUI for your game. You are free in designing it the way you like.
Must be able to:
1 vs 1 or 2 vs 2
You have the freedom to add other formations.
Please do it in Java!
here is the code of the game with no gui, it is a battle royale, there are 4 character classes:
Character Classes: //Character.java package project3.characters; public abstract class Character { /** * The Name. */ protected String name; /** * The Strength. */ protected int strength; /** * The Health. */ protected int health; /** * Instantiates a new Character. * * @param name the name */ public Character(String name) { this.name = name; } /** * Gets name. * @return the name */ public String getName() { return name; } /** * Gets strength. * @return the strength */ public int getStrength() { return strength; } /** * Gets health. * @return the health */ public int getHealth() { return health; } /** * Attack int. * @return the int */ public abstract int attack(); /** * Hit. * * @param points the points */ public abstract void hit(int points); /** * Is alive boolean. * @return the boolean */ public abstract boolean isAlive(); @Override public String toString() { return "Name: " + name + ", Strength: " + strength + ", Health: " + health ; } }
//=>Hulk.java
//Hulk.java package project3.characters; public class Hulk extends Character { public Hulk(String name) { super(name); this.health = 100; this.strength = 10; } @Override public int attack() { return strength; } @Override public void hit(int points) { this.health -= points; } @Override public boolean isAlive() { return health>=0; } @Override public String toString() { return "[ Class: Hulk, "+super.toString()+" ]"; } }
//=>Monster.java
package project3.characters; public class Monster extends Character { public Monster(String name) { super(name); this.health = 100; this.strength = 5; } @Override public int attack() { return strength; } @Override public void hit(int points) { this.health -= points; } @Override public boolean isAlive() { return health>=0; } @Override public String toString() { return "[ Class: Monster, "+super.toString()+" ]"; } }
//=>Venom.java
package project3.characters; public class Venom extends Character { public Venom(String name) { super(name); this.health = 100; this.strength = 15; // more powerful to attack } @Override public int attack() { return strength; } @Override public void hit(int points) { this.health -= points; } @Override public boolean isAlive() { return health >= 0; } @Override public String toString() { return "[ Class: Venom, "+super.toString()+" ]"; } }
driver class that makes battle royale happen:
// Using the code of the above 4 given classes
package project3;
import project3.characters.Character; import project3.characters.Hulk; import project3.characters.Monster; import project3.characters.Venom;
import java.util.Random;
public class BattleRoyale {
// method that counts the number of alive characters and return it public static int numAlive(Character[] characters) { int count = 0; // initialize count to 0 // loop over the array of characters for(int i=0;i { if(characters[i].isAlive()) // ith character is alive, increment count by 1 count++; } return count; } public static void main(String[] args) {
// create an array of 4 characters Character[] characters = new Character[4]; // populate the array characters[0] = new Hulk("John"); characters[1] = new Monster("Harry"); characters[2] = new Venom("Tom"); characters[3] = new Monster("Charlie"); Random ran = new Random(); int attackerIdx, otherIdx; // display the characters at the start of battle System.out.println("Characters at the beginning Battle Royale: "); for(int i=0;i { System.out.println(characters[i]); } System.out.println(); // loop that continues until there is only 1 character alive while(numAlive(characters) > 1) { attackerIdx = ran.nextInt(characters.length); // randomly generate the attacker index // loop that continues until the character at attacker index is alive while(!characters[attackerIdx].isAlive()) attackerIdx = ran.nextInt(characters.length); // randomly generate the other character index otherIdx = ran.nextInt(characters.length); // loop that continues until the character at other index is alive and the indices of attacker and other is not the same while((attackerIdx == otherIdx) || (!characters[otherIdx].isAlive())) { otherIdx = ran.nextInt(characters.length); } // display the details of who attacked whom System.out.println(characters[attackerIdx].getName()+" attacked "+characters[otherIdx].getName()); characters[otherIdx].hit(characters[attackerIdx].attack()); // attack // display the updated health of the character who was attacked System.out.println(characters[otherIdx].getName()+" health is: "+characters[otherIdx].getHealth()+" "); } // display the characters at the end of the battle System.out.println("Characters at the end of Battle Royale: "); for(int i=0;i { System.out.println(characters[i]); } // display the character who won for(int i=0;i { if(characters[i].isAlive()) { System.out.println(characters[i].getName()+" is the winner."); break; } } }
}
// end of BattleRoyale.java
make this game into a gui please!
it's fine if it takes more than 2 hours, also doesn't have to be complicated.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
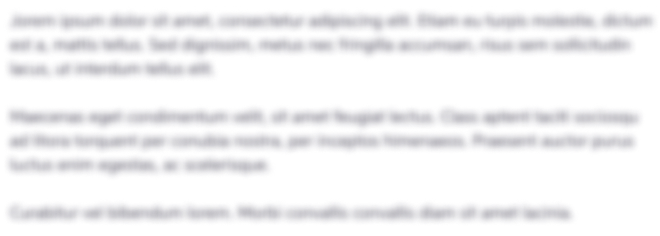
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started