Question
Create a MyPoint class to model a point in a two-dimensional space. The MyPoint class contains: Two data fields x and y that represent the
Create a MyPoint class to model a point in a two-dimensional space.
The MyPoint class contains:
Two data fields x and y that represent the coordinates.
A no-arg constructor that creates a point (0,0).
A constructor that constructs a point with specified coordinates.
Two get functions for data fields x and y, respectively.
Two set functions for data fields x and y, respectively.
A function named distance that returns the distance from this point to another point of the MyPoint type.
Create ThreeDPoint class to model a point in a three-dimensional space.
Let ThreeDPoint be derived from MyPoint with the following additional features:
A data field named z that represents the z-coordinate.
A no-arg constructor that constructs a point with coordinates (0,0,0).
A constructor that constructs a point with three specified coordinates.
A get function that returns the z value. A set function that that sets the z value.
Override the distance function to return the distance between two points in the threedimensional space.
Put both of your classes in a file called point.cpp.
the code will be tested with code similar to below.
#include "point.cpp"
int main()
{
MyPoint p1(1, 2);
MyPoint p2(4, 2.5);
cout << p1.distance(p2) << endl; p1.setX(1);
p1.setY(1); p2.setX(2); p2.setY(2);
cout << p1.distance(p2) << endl;
ThreeDPoint p3(2,3,1);
ThreeDPoint p4(8,-5,0);
cout << p3.distance(p4) << endl; return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
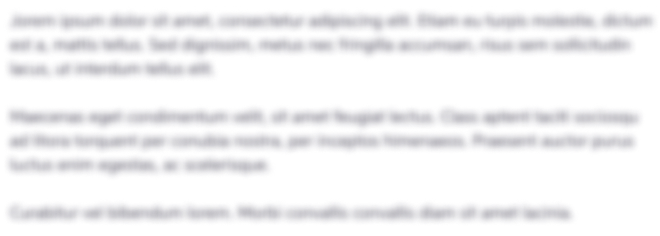
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started