Question
Create a new C++ String Class You are to implement a class that stores a list of strings* and, in part 2, write functions that
Create a new C++ String Class
You are to implement a class that stores a list of strings* and, in part 2, write functions that use objects of your class. The class should use a dynamic array as its underlying representation.
- default constructor creates an array of strings of size 4 in dynamic memory and assigns its address to the class' string pointer, sets maximum size to 4, and current size to 0.
- copy constructor creates a deep copy of its constant StringList reference parameter.
- destructor deallocates dynamic memory associated with the objects string (array) pointer.
- overloaded assignment operator deep copies its constant StringList reference parameter to the calling object; deallocates dynamic memory associated with the calling object's original array; returns a reference to the calling object; should behave correctly during self-assignment.
- insert inserts its string parameter (the first parameter) at the index specified by its integer parameter (the second parameter). Before insertion all array element at or above the insertion point are moved up one position in the array (to make space for the inserted value). If the underlying array is full before the insertion it first doubles the maximum size attribute, creates an array of twice the size of the current array, copies the contents of the old array to the new array, frees memory associated with the old array, and assigns new arrays address to objects array pointer, then inserts the string and increments current size. If the index parameter is less than 0 or greater than the current size the method should throw an out_of_range exception.
- remove returns the string stored at the index given by its integer parameter. Moves down all array elements above the index by one position in the array (thereby overwriting the removed value) and decrements current size. If the index parameter is less than 0 or greater than current size-1 the method should throw an out_of_range exception.
- swap - swaps the two array elements stored at the indexes given by its two integer parameters. If either index parameter is less than 0 or greater than current size-1 the method should throw an out_of_range exception.
- retrieve returns the string stored at the index given by its integer parameter. If the index parameter is less than 0 or greater than current size-1 the method should throw an out_of_range exception.
- size returns an integer equal to the number of values in the calling object.
- concatenate returns a StringList object that contains the contents of the calling object followed by the contents of its constant StringList reference parameter; the size of the returned object's underlying array should be the greater of 4 and the number of values stored in it.
Attributes
Your class should be implemented with a dynamic array and should have the following private member variables
A pointer to a string that will be used to point to an array of strings in dynamic memory An int that records the current size of the array (the number of values stored in the array) An int that records the maximum size of the array
Your class should consist of a header file called StringList.h that contains the class definition and an implementation file called StringList.cpp that contains the method definitions.
Libraries
The only libraries that should be included are
Step by Step Solution
There are 3 Steps involved in it
Step: 1
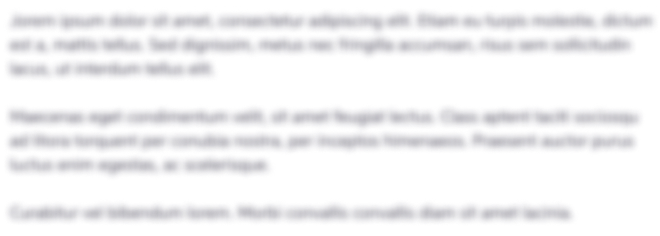
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started