Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create a new class that inherits the character class for an enemy e.g., a Dragon class that extends the character class In the new class,
Create a new class that inherits the character class for an enemy e.g., a Dragon class that extends the character class In the new class, create a function that provides an alternate attack for this enemy e.g., a breathe fire attack that inflicts extra damage In your class' getAction() implementation, have the new enemy class use its alternate attack 30% of the time Set up rand() to generate a number 1-100 and if the value is below 30, have the enemy use its special move Examples 1- #include "character.h" using namespace std; // Constructor // Initializer list must also be used to call constructor of member class objects Character::Character(string n, int hp, int str, int def, int spd):potions(3) { name = n; if(hp < 0) healthPoints = 0; else healthPoints = hp; if(str < 0) strength = 0; else strength = str; if(def < 0) defense = 0; else defense = def; if(spd < 0) speed = 0; else speed = spd; maxHp = healthPoints; } // Action Member Functions int Character::attack(void){ return strength; } void Character::defend(int damage){ int damageReceived = damage - defense; if(damageReceived < 0) damageReceived = 0; healthPoints = healthPoints - damageReceived; if(healthPoints < 0) healthPoints = 0; } // Status Member Functions: bool Character::isDead(void){ if(healthPoints <= 0) return true; else return false; } int Character::getSpeed(){ return speed; } string Character::getName(){ return name; } int Character::getHealthPoints(void){ return healthPoints; } void Character::heal(int healingAmount){ healthPoints += healingAmount; if(healthPoints > maxHp) healthPoints = maxHp; } int Character::getAction(Character & c){ string input; int temp; cout << "What would you like to do?" << endl; cout << "1: Attack" << endl; cout << "2: Use Potion (" << potions.getCount() << ")" << endl; cin >> input; char choice = input[0]; switch(choice){ case '1': cout << getName() << " attacks " << c.getName() << "!" << endl; c.defend(attack()); return 0; break; case '2': temp = potions.use(); if(temp != 0){ heal(temp); return 0; } else { cout << "Out of potions!" << endl; } break; default: cout << "Invalid option!" << endl; break; } return -1; } void Character::printStatus(void){ cout << getName() << " HP: " << getHealthPoints() << endl; } 2-#include #include "potion.h" class Character{ // Private data members (these are only accessible from inside the class) private: std::string name; int maxHp; int strength; int defense; int speed; int healthPoints; // Public data members and member functions (these are accessible anywhere) public: Potion potions; Character(std::string, int, int, int, int); int attack(void); void defend(int); void heal(int); bool isDead(void); int getSpeed(void); std::string getName(void); int getHealthPoints(void); virtual int getAction(Character & c); virtual void printStatus(void); }; 3-#include #include #include #include "wizard.h" using namespace std; void combat(Character&, Character&); int main(int argc, char * argv[]){ Wizard playerCharacter("Wizard", 10, 5, 3, 5, 100); Character npc("Dragon", 10, 4, 4, 3); // clear screen if (system("CLS")) system("clear"); while(!playerCharacter.isDead() && !npc.isDead()){ playerCharacter.printStatus(); npc.printStatus(); combat(playerCharacter, npc); // delay 1s and clear screen sleep(1); if (system("CLS")) system("clear"); } if(!playerCharacter.isDead()) cout << playerCharacter.getName() << " wins!" << endl; else cout << npc.getName() << " wins!" << endl; return 0; } 4-#include "potion.h" Potion::Potion(void){ } Potion::Potion(int amount){ count = amount; if(count < 0) count = 0; } int Potion::getCount(void) { return count; } void Potion::setCount(int n) { count = n; } int Potion::use(void){ if(count > 0){ count--; return healingAmount; } else { return 0; } } 5-#include "wizard.h" using namespace std; // Constructor for Wizard // Initializer list must be used to call constructor of base class Wizard::Wizard(string name, int hp, int str, int def, int spd, int mn) : Character(name, hp, str, def, spd){ mana = mn; maxMana = mn; } int Wizard::getMana(void){ return mana; } void Wizard::restoreMana(int amount){ mana += amount; if(mana > maxMana) mana = maxMana; } int Wizard::castMagicSpell(void){ int spellCost = 20; int spellDamage = 10; if(mana < spellCost){ return 0; } else { mana = mana - spellCost; return spellDamage; } } int Wizard::getAction(Character & c){ string input; int temp; cout << "What would you like to do?" << endl; cout << "1: Attack" << endl; cout << "2: Use Potion (" << potions.getCount() << ")" << endl; cout << "3: Cast Magic Spell" << endl; cin >> input; char choice = input[0]; switch(choice){ case '1': cout << getName() << " attacks " << c.getName() << "!" << endl; c.defend(attack()); return 0; break; case '2': temp = potions.use(); if(temp != 0){ heal(temp); return 0; } else { cout << "Out of potions!" << endl; } break; case '3': temp = castMagicSpell(); if(temp != 0){ cout << getName() << " casts magic spell at " << c.getName() << "!" << endl; c.defend(temp); return 0; } else { cout << "Not enough mana!" << endl; } default: cout << "Invalid option!" << endl; break; } return -1; } void Wizard::printStatus(void){ cout << getName() << " HP: " << getHealthPoints() << " Mana: " << getMana() << endl; } 6-#include #include "character.h" class Wizard : public Character{ private: int mana; int maxMana; public: Wizard(std::string, int, int, int, int, int); int castMagicSpell(void); int getMana(void); void restoreMana(int); virtual int getAction(Character & c); virtual void printStatus(void); };
Create a new class that inherits the character class for an enemy
e.g., a Dragon class that extends the character class
In the new class, create a function that provides an alternate attack for this enemy
e.g., a breathe fire attack that inflicts extra damage
In your class' getAction() implementation, have the new enemy class use its alternate attack 30% of the time
Set up rand() to generate a number 1-100 and if the value is below 30, have the enemy use its special move
Examples
1- #include "character.h"
using namespace std;
// Constructor
// Initializer list must also be used to call constructor of member class objects
Character::Character(string n, int hp, int str, int def, int spd):potions(3) {
name = n;
if(hp < 0) healthPoints = 0;
else healthPoints = hp;
if(str < 0) strength = 0;
else strength = str;
if(def < 0) defense = 0;
else defense = def;
if(spd < 0) speed = 0;
else speed = spd;
maxHp = healthPoints;
}
// Action Member Functions
int Character::attack(void){
return strength;
}
void Character::defend(int damage){
int damageReceived = damage - defense;
if(damageReceived < 0)
damageReceived = 0;
healthPoints = healthPoints - damageReceived;
if(healthPoints < 0)
healthPoints = 0;
}
// Status Member Functions:
bool Character::isDead(void){
if(healthPoints <= 0)
return true;
else
return false;
}
int Character::getSpeed(){
return speed;
}
string Character::getName(){
return name;
}
int Character::getHealthPoints(void){
return healthPoints;
}
void Character::heal(int healingAmount){
healthPoints += healingAmount;
if(healthPoints > maxHp)
healthPoints = maxHp;
}
int Character::getAction(Character & c){
string input;
int temp;
cout << "What would you like to do?" << endl;
cout << "1: Attack" << endl;
cout << "2: Use Potion (" << potions.getCount() << ")" << endl;
cin >> input;
char choice = input[0];
switch(choice){
case '1':
cout << getName() << " attacks " << c.getName() << "!" << endl;
c.defend(attack());
return 0;
break;
case '2':
temp = potions.use();
if(temp != 0){
heal(temp);
return 0;
} else {
cout << "Out of potions!" << endl;
}
break;
default:
cout << "Invalid option!" << endl;
break;
}
return -1;
}
void Character::printStatus(void){
cout << getName() << " HP: " << getHealthPoints() << endl;
}
2-#include
#include "potion.h"
class Character{
// Private data members (these are only accessible from inside the class)
private:
std::string name;
int maxHp;
int strength;
int defense;
int speed;
int healthPoints;
// Public data members and member functions (these are accessible anywhere)
public:
Potion potions;
Character(std::string, int, int, int, int);
int attack(void);
void defend(int);
void heal(int);
bool isDead(void);
int getSpeed(void);
std::string getName(void);
int getHealthPoints(void);
virtual int getAction(Character & c);
virtual void printStatus(void);
};
3-#include
#include
#include
#include "wizard.h"
using namespace std;
void combat(Character&, Character&);
int main(int argc, char * argv[]){
Wizard playerCharacter("Wizard", 10, 5, 3, 5, 100);
Character npc("Dragon", 10, 4, 4, 3);
// clear screen
if (system("CLS")) system("clear");
while(!playerCharacter.isDead() && !npc.isDead()){
playerCharacter.printStatus();
npc.printStatus();
combat(playerCharacter, npc);
// delay 1s and clear screen
sleep(1);
if (system("CLS")) system("clear");
}
if(!playerCharacter.isDead())
cout << playerCharacter.getName() << " wins!" << endl;
else
cout << npc.getName() << " wins!" << endl;
return 0;
}
4-#include "potion.h"
Potion::Potion(void){
}
Potion::Potion(int amount){
count = amount;
if(count < 0)
count = 0;
}
int Potion::getCount(void) {
return count;
}
void Potion::setCount(int n) {
count = n;
}
int Potion::use(void){
if(count > 0){
count--;
return healingAmount;
} else {
return 0;
}
}
5-#include "wizard.h"
using namespace std;
// Constructor for Wizard
// Initializer list must be used to call constructor of base class
Wizard::Wizard(string name, int hp, int str, int def, int spd, int mn) : Character(name, hp, str, def, spd){
mana = mn;
maxMana = mn;
}
int Wizard::getMana(void){
return mana;
}
void Wizard::restoreMana(int amount){
mana += amount;
if(mana > maxMana)
mana = maxMana;
}
int Wizard::castMagicSpell(void){
int spellCost = 20;
int spellDamage = 10;
if(mana < spellCost){
return 0;
} else {
mana = mana - spellCost;
return spellDamage;
}
}
int Wizard::getAction(Character & c){
string input;
int temp;
cout << "What would you like to do?" << endl;
cout << "1: Attack" << endl;
cout << "2: Use Potion (" << potions.getCount() << ")" << endl;
cout << "3: Cast Magic Spell" << endl;
cin >> input;
char choice = input[0];
switch(choice){
case '1':
cout << getName() << " attacks " << c.getName() << "!" << endl;
c.defend(attack());
return 0;
break;
case '2':
temp = potions.use();
if(temp != 0){
heal(temp);
return 0;
} else {
cout << "Out of potions!" << endl;
}
break;
case '3':
temp = castMagicSpell();
if(temp != 0){
cout << getName() << " casts magic spell at " << c.getName() << "!" << endl;
c.defend(temp);
return 0;
} else {
cout << "Not enough mana!" << endl;
}
default:
cout << "Invalid option!" << endl;
break;
}
return -1;
}
void Wizard::printStatus(void){
cout << getName() << " HP: " << getHealthPoints() << " Mana: " << getMana() << endl;
}
6-#include
#include "character.h"
class Wizard : public Character{
private:
int mana;
int maxMana;
public:
Wizard(std::string, int, int, int, int, int);
int castMagicSpell(void);
int getMana(void);
void restoreMana(int);
virtual int getAction(Character & c);
virtual void printStatus(void);
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
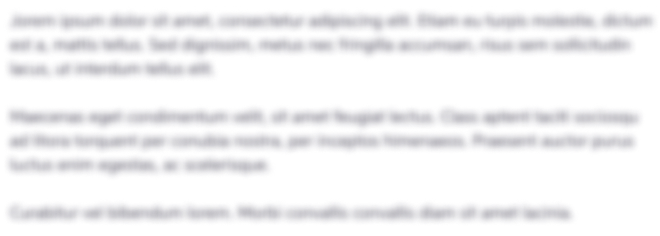
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started