Question
Create a NON-RECURSIVE version of this function, it stores any value less than or equal to k on the left side and the rest of
Create a NON-RECURSIVE version of this function, it stores any value less than or equal to k on the left side and the rest of the numbers on the right.
***INCLUDE TIME COMPLEXITY
#include
// Use the standard namespace.
using namespace std;
// Define the function to rearrange the elements.
void rearrange(int A[], int k, int start, int end)
{
// Return if the starting index is equal
// to the last index.
if (start == end)
{
return;
}
// Otherwise, do the following.
else
{
// Swap the element at A[start] with the
// element at A[end] if the value of A[start]
// is greater than k.
if (A[start] > k)
{
int temp = A[start];
A[start] = A[end];
A[end] = temp;
// Decrement the value of end by 1
// and call the function recursively.
rearrange(A, k, start, end - 1);
}
// Otherwise, increment the value of start
// by 1 and call the function recursively.
else
{
rearrange(A, k, start + 1, end);
}
}
}
// Define the main() function.
int main()
{
// Declare the required variables.
int A[] = { 100,-1, 4,3,2,0,23,34,6,7,102 };
int n = sizeof(A) / sizeof(A[0]);
int k = 20;
cout << "k = " << k << endl;
cout << "The original array is as follows:" << endl;
// Start the loop to print the original array.
for (int i = 0; i < n; i++)
{
cout << A[i] << " ";
}
// Call the function to rearrange the elements.
rearrange(A, k, 0, n - 1);
cout << endl;
cout << " The modified array is as follows:" << endl;
// Start the loop to print the modified array.
for (int i = 0; i < n; i++)
{
cout << A[i] << " ";
}
// Return 0 and exit the program.
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
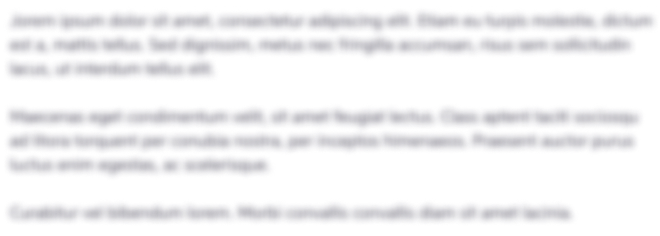
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started