Question
Create a package named file_operations and a class named FileOperations which will receive one or more command line pathnames to command files. Each command file
Create a package named file_operations and a class named FileOperations which will receive one or more command line pathnames to command files. Each command file will be opened and processed line by line. Each line can contain one of the following commands:
? - This command will print out the legal commands available
createFile - The first string following "createFile" will be treated as a filename, and the remaining strings will be written to the file separated by new lines.
printFile - The first string following "printFile" will be treated as a filename that will be opened up and printed out to the screen.
lastModified - The first string following "lastModified" will be treated as a filename for which we will print out the date when this file was last modified.
size - The first string following "size" will be treated as a filename for which we will print out the number of bytes it contains.
rename - The first string following "rename" will be treated as the current filename and the second string will be treated as the new filename we desire.
mkdir - The first string following "mkdir" will be treated as the name of a directory that should be created.
delete - The first string following "delete" will be treated as the name of a file that should be deleted.
list - The first string following "list" will be treated as the name of a directory for which we want a list of the files it contains.
quit - exit program
Anything else is a bad command
Here is a template that you can use.
// figure out your imports public class FileOperations { StringTokenizer parseCommand; public void delete() { // code for handling the delete command // Make sure you check the return code from the // File delete method to print out success/failure status } public void rename() { // code for handling the rename command // Make sure you check the return code from the // File rename method to print out success/failure status } public void list() { // code for handling the list command } public void size() { // code for handling the size command } public void lastModified() { // code for handling the lastModified command } public void mkdir() { // code for handling the mkdir command // Make sure you check the return code from the // File mkdir method to print out success/failure status } public void createFile() { // code for handling the createFile command } public void printFile() { // code for handling the printFile command } void printUsage() { // process the "?" command } // useful private routine for getting next string on the command line private String getNextToken() { if (parseCommand.hasMoreTokens()) return parseCommand.nextToken(); else return null; } // useful private routine for getting a File class from the next string on the command line private File getFile() { File f = null; String fileName = getNextToken(); if (fileName == null) System.out.println("Missing a File name"); else f = new File(fileName); return f; } public boolean processCommandLine(String line) { // if line is not null, then setup the parseCommand StringTokenizer. Pull off the first string // using getNextToken() and treat it as the "command" to be processed. // It would be good to print out the command you are processing to make your output more readable. // If you are using at least java 1.7, you can use a switch statement on command. // Otherwise, resort to if-then-else logic. Call the appropriate routine to process the requested command: // i.e. delete, rename, mkdir list, etc. // return false if command is quit or the line is null, otherwise return true. } void processCommandFile(String commandFile) { // Open up a scanner based on the commandFile file name. Read the commands from this file // using the Scanner line by line. For each line read, call processCommandLine. Continue reading // from this file as long as processCommandLine returns true and there are more lines in the file. // At the end, close the Scanner. } public static void main(String[] args) { FileOperations fo= new FileOperations(); for (int i=0; i < args.length; i++) { System.out.println(" ============ Processing " + args[i] +" ======================= "); fo.processCommandFile(args[i]); } System.out.println("Done with FileOperations"); } }
Once you have your program written, I want you to add the following files to the top level of your project and run the test that I request:
cmd1.txt
? createFile file1.txt a b c d e f g printFile file1.txt lastModified file1.txt size file1.txt rename file1.txt newFile1.txt mkdir dir createFile dir/file2.txt 1 2 3 4 5 6 7 8 9 printFile dir/file2.txt rename dir/file2.txt dir/newFile2.txt list . list dir
cmd2.txt
createFile file2.txt x y z printFile file2.txt list . delete newFile1.txt delete file2.txt delete dir/newFile2.txt delete dir printFile unknown.txt size unknown.txt lastModified unknown.txt rename unknown.txt newUnknown.txt list . quit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
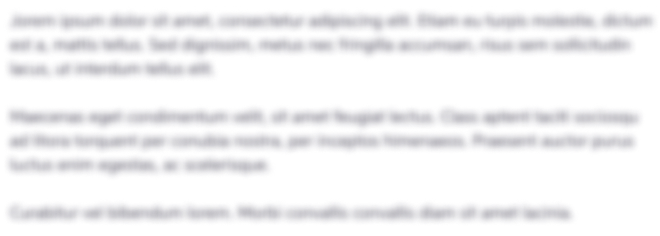
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started