Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create a package named zoo and execute the following instructions. You run a zoo which contains Animals. So each Animal will be represented by an
Create a package named zoo and execute the following instructions.
You run a zoo which contains Animals. So each Animal will be represented by an abstract class. Consider creating classes Animal, Cow, Horse, Snake, etc. Your Animal class will have the following instance variables: private String name; private double weight; private int age; Your Animal class will have the following constructors: Animal() Animal(String n, double weight, int age) Your Animal class will have the following methods: String makeNoise() - this will be an abstract method double getWeight() - returns the weight of this animal public String toString() - returns a String with information about this Animal *************************************** You will have the following classes which all extend Animal: Cow, Horse, Snake. Cow will have an instance variable called: private int num_spots All variables should be initialized after either of the 2 constructors: Cow() Cow(String name, double weight, int age, int num_spots) You will have the methods String makeNoise() which returns "Moooo" toString() - returns info about all variables including Animal things ********************************************** Horse will have an instance variable called: private double top_speed All variables should be initialized after either of the 2 constructors: Horse() Horse(String name, double weight, int age, double top_speed) You will have the methods String makeNoise() which returns "Whinny" toString() - returns info about all variables including Animal things ********************************************** Snake will have an instance variable called: private int num_fangs All variables should be initialized after either of the 2 constructors: Snake() Snake(String name, double weight, int age, int num_fangs) You will have the methods String makeNoise() which returns "Hisssssss" toString() - returns info about all variables including Animal things ********************************************** You will have a Zoo class that contains the following instance variables: private int actual_num_animals; private int num_cages; private Animal[] animals; You will have the constructors: Zoo() - default num_cages will be 3 Zoo(int num_cages) You will have the following methods: void add(Animal a) - adds an animal to your Zoo double total_weight() - returns the total weight of all animals in the zoo void make_all_noises() - Print out the noises made by all of the animals. In otherwords, it calls the makeNoise() method for all animals in the zoo. void print_all_animals() - prints the results of calling toString() on all animals in the zoo. ************************************************ Generate output by running the following main in the Zoo class: The results should go into the JH6_worksheet.txt public static void main(String[] args) { Zoo z = new Zoo(); Snake sly = new Snake("Sly", 5.0 , 2, 2); Snake sly2 = new Snake("Slyme", 10.0 , 1, 2); Cow blossy = new Cow("Blossy", 900., 5, 10); Horse prince = new Horse("Prince", 1000., 5, 23.2); // Following not allowed because Animal is abstract //Animal spot = new Animal("Spot", 10., 4); z.add(sly); z.add(sly2); z.add(blossy); z.add(prince); z.make_all_noises(); System.out.println("Total weight =" + z.total_weight()); System.out.println("**************************"); System.out.println("Animal Printout:"); z.print_all_animals(); System.out.println("********* Now we will make the Second Zoo"); Zoo z2 = new Zoo(5); z2.add(sly); z2.add(sly2); z2.add(blossy); z2.add(prince); z2.add( new Horse("Warrior", 1200, 6, 25.3)); z2.add( new Horse("Harry", 1100, 4, 21.3)); System.out.println("Total weight of z2="+z2.total_weight()); z2.make_all_noises(); z2.print_all_animals(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
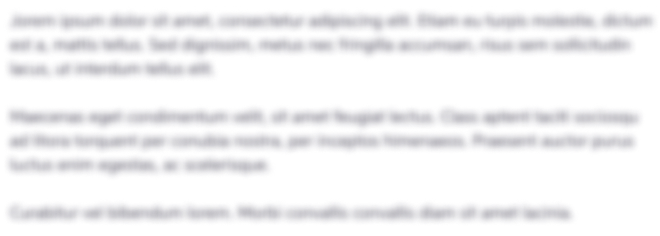
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started