Question
create a program with the following specifications: Beginning with the file named Proj01.java, the new file named Proj01Runner.java Note that you must not modify code
create a program with the following specifications:
Beginning with the file named Proj01.java, the new file named Proj01Runner.java
Note that you must not modify code in the file named Proj01.java.
Be sure to display your name in the output as indicated.
When you place both files in the same folder, compile them both, and run the file named Proj01.java with a command-line argument of 5, the program must display the text shown below on the command line screen.
I certify that this program is my own work and is not the work of others. I agree not to share my solution with others.
Replace this line with your name
1 4 3 3 3 1
1 4 3 3 3 1
1 3 4
Sum = 8
The output text must match my output text for a command-line argument of any numeric value that you choose.
When you place both files in the same folder, compile them both, and run the file named Proj01.java without a command-line argument, the program must display text that is similar to, but not necessarily the same as the text shown below on the command line screen. In this case, the numeric values are based on a random number generator that will change from one run to the next. In all cases, the values in the first two lines of numbers must match. The values in the third line of numbers must be the unique values from the first line and must be in ascending numeric order. The value of the last line must be the sum of the numbers in the third line.
I certify that this program is my own work and is not the work of others. I agree not to share my solution with others.
Replace this line with your name
1 2 1 0 2 1
1 2 1 0 2 1
0 1 2
Sum = 3
/*File Proj01.java
The purpose of this assignment is to assess the student's
ability to write a program dealing with basic Collection
Framework concepts and methods.
***********************************************************/
// Student must not modify the code in this file. //
import java.util.*;
class Proj01{
public static void main(String[] args){
//Create a pseudo-random number generator
Random generator = null;
if(args.length != 0){
generator = new Random(Long.parseLong(args[0]));
}else{
generator = new Random(new Date().getTime());
};
//Generate some small positive random numbers and store
// them in an array object of type int.
int[] vals = {Math.abs(generator.nextInt())%5,
Math.abs(generator.nextInt())%5,
Math.abs(generator.nextInt())%5,
Math.abs(generator.nextInt())%5,
Math.abs(generator.nextInt())%5,
Math.abs(generator.nextInt())%5
};
System.out.println();//blank line
//Instantiate an object from the student's code.
// Pass the vals array as a parameter.
Proj01Runner obj = new Proj01Runner(vals);
//Display the data in the vals array.
for(int cnt = 0;cnt < vals.length;cnt++){
System.out.print(vals[cnt] + " ");
}//end for loop
System.out.println();//blank line
//Call the runA method on the student's object. Store
// the return value in a reference variable named ref
// of type Collection.
Collection ref = obj.runA();
//Display the return values from the runA method
Iterator iter = ref.iterator();
while(iter.hasNext()){
System.out.print(iter.next() + " ");
}//end while loop
System.out.println();//blank line
//Call the runB method on the student's object. Store
// the return value in the reference variable named ref
// of type Collection.
ref = obj.runB();
//Display the return values and the sum of the
// return values from the runB method
int sum = 0;
iter = ref.iterator();
while(iter.hasNext()){
Integer myObj = (Integer)(iter.next());
System.out.print(myObj + " ");
sum += myObj.intValue();
}//end while loop
System.out.println("\nSum = " + sum);
System.out.println();//blank line
}//end main
}//end class Proj01
//End program specifications.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
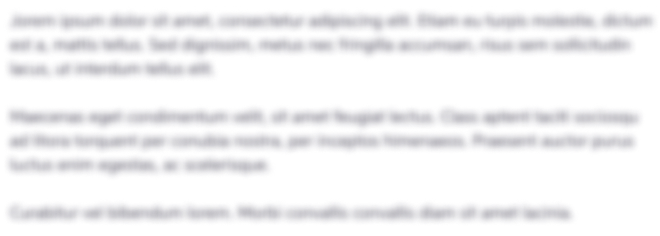
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started