Question
Create a Python Program that uses IBM Watson to translate text from one language to another. Install the Requests Python library from the MS DOS
Create a Python Program that uses IBM Watson to translate text from one language to another.
Install the Requests Python library from the MS DOS command prompt. Theres a video in Canvas that instructs you on how to do this.
Test that the requests library is installed correctly by entering import requests at the Python command prompt. Contact the instructor if you need assistance.
Now create a new Python program and name it Watson_Translate.py
Now place import requests below the comments
Now create a function named cls(). The job of cls() is to print 5 new s when called.
Now create a function named translate_text() as follows: def translate_text(text,source,target):
username = '614105fe-6379-4bcd-8253-c3a76ba92c1f'
password = 'KZ7msi8EqnmD'
watsonUrl = 'https://gateway.watsonplatform.net/language-translation/api/v2/translate?source=' + source + '&target=' + target + '&text=' + text
try:
r = requests.get(watsonUrl,auth=(username,password))
return r.text
except:
return False
In the function you just created what are the three formal parameters? What purpose do they serve in the function? Place your answer as a comment in your code.
In a comment answer the following question Explain how the try/except works by placing a comment in the function. Be as specific as possible.
Now create a function named welcome() that has the following code in it:
message = "Welcome to the IBM Watson Translator "
print(message + "-" * len(message) + " ")
print("Have fun! ")
In your code answer the following question by placing a comment in the welcome() function What is the purpose of the len() around the message? What specifically is it doing?
Now create a main function.
In the main function call the cls() and welcome() functions
Now copy and paste this code into the main function: data = input("Enter some text to be translated: ")
print()
print("What language should I translate it to?")
print("1) Spanish")
print("2) Arabic")
print("3) French")
print("4) Portuguese")
print()
target = input("Select a language from the list above: ")
if target == "1":
target = 'es'
elif target == "2":
target = 'ar'
elif target == "3":
target = 'fr'
elif target == "4":
target = 'pt'
results = translate_text(data,'en',target)
print()
print("Here is the text translated for you:")
print(results)
Now test your code and see if it runs.
Question: Explain what this line of code does: results = translate_text(data,'en',target). Place the answer as comment in your code
Step by Step Solution
There are 3 Steps involved in it
Step: 1
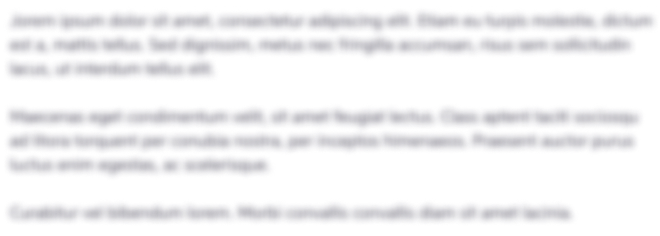
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started