Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Create a recursive Java method getProduct to compute the product of two positive integers, m and n , using only addition and subtraction. Implement the
Create a recursive Java method getProduct to compute the product of two positive integers, m and n using only addition and subtraction. Implement the Java code. Test the method by calling it in the main method of the relevant class. Hint: You need subtraction to count down from m or n and addition to do the arithmetic needed to get the right answer. The linearSum method may be helpful.
public class ArraySum
Returns the sum of the first n integers of the given array.
public static int linearSumint data, int n
if n
return ;
else
return linearSumdata n datan;
Returns the sum of subarray datalow through datahigh inclusive.
public static int binarySumint data, int low, int high
if low high zero elements in subarray
return ;
else if low high one element in subarray
return datalow;
else
int mid low high;
return binarySumdata low, mid binarySumdata mid high;
public static void mainString args
for int limit ; limit ; limit
int data new intlimit;
for int k; k limit; k
datak k;
int answer limit limit ;
int linear linearSumdata limit;
if linear answer
System.out.printlnProblem with linear sum for n limit;
int binary binarySumdata limit;
if binary answer
System.out.printlnProblem with binary sum for n limit;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
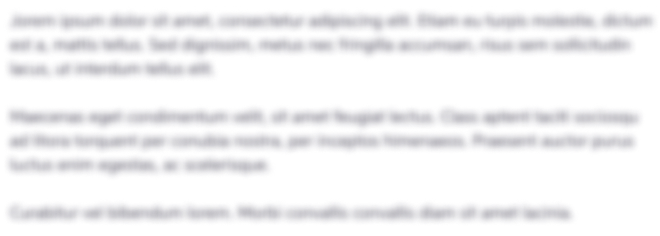
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started