Question
Create a UML of: public class SinglyLinkedListImpl { private Node head; private Node tail; public void add(T element){ Node nd = new Node (); nd.setValue(element);
Create a UML of:
public class SinglyLinkedListImpl
System.out.println("Adding: "+element); if(head == null){ head = nd;
tail = nd;
} else {
tail.setNextRef(nd); } tail = nd;
}
}
public void adding_After(T element, T after){ Node
Node
System.out.println("Traversing to all nodes..");
while(true){
if(tmp == null){
break;
}
if(tmp.compareTo(after) == 0){
refNode = tmp;
break;
}
tmp = tmp.getNextRef();
}
if(refNode != null){
Node
nd.setValue(element);
nd.setNextRef(tmp.getNextRef());
if(tmp == tail){
tail = nd;
}
tmp.setNextRef(nd);
} else {
System.out.println("Unable to find the given element...");
}
}
public void delete_Front(){
if(head == null){
System.out.println("Underflow...");
}
Node
head = tmp.getNextRef();
if(head == null){
tail = null;
}
System.out.println("Deleted: "+tmp.getValue());
}
public void deleteAfter(T after){
Node
Node
System.out.println("Traversing to all nodes..");
while(true){
if(tmp == null){
break;
}
if(tmp.compareTo(after) == 0){
refNode = tmp;
break;
}
tmp = tmp.getNextRef();
}
if(refNode != null){
tmp = refNode.getNextRef();
refNode.setNextRef(tmp.getNextRef());
if(refNode.getNextRef() == null){
tail = refNode;
}
System.out.println("Deleted: "+tmp.getValue());
} else {
System.out.println("Unable to find the given element...");
}
}
public void traverse(){
Node
while(true){
if(tmp == null){
break;
}
System.out.println(tmp.getValue());
tmp = tmp.getNextRef();
}
}
public static void main(String a[]){
SinglyLinkedListImpl
sl.add(3);
sl.add(32);
sl.add(54);
sl.add(89);
sl.adding_After(76, 54);
sl.delete_Front();
sl.deleteAfter(76);
sl.traverse();
}
}
class Node
private T value;
private Node
public T getValue() {
return value;
}
public void setValue(T value) {
this.value = value;
}
public Node
return nextRef;
}
public void setNextRef(Node
this.nextRef = ref;
}
public int compareTo(T arg) {
if(arg == this.value){
return 0;
} else {
return 1;
}
}
}
This program helps to implement the concept of single linked list.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
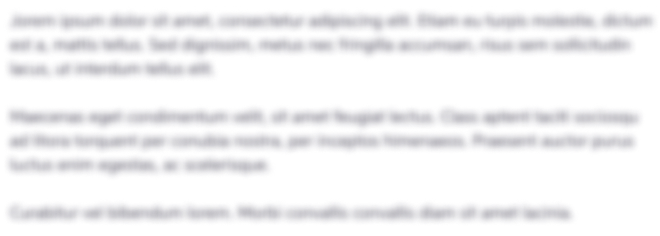
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started