Question
Create an inheritance hierarchy that a bank might use to represent customers bank accounts. All customers at this bank can deposit (i.e., credit) money into
Create an inheritance hierarchy that a bank might use to represent customers bank accounts. All customers at this bank can deposit (i.e., credit) money into their accounts and withdraw (i.e., debit) money from their accounts. More specific types of accounts also exist. Savings accounts, for instance, earn interest on the money they hold. Checking accounts, on the other hand, charge a fee per transaction (i.e., credit or debit). When an account is setup the date is recorded. When there is an update the date is recorded.
Create an inheritance hierarchy containing base class Account and derived classes SavingsAccount and CheckingAccount that inherit from class Account. Account class has Date class as compositions.
Account class
Base class Account should include one data member of type double to represent the account balance, two string objects to represent first name and last name, and two Date objects to represent account starting date and last account update date.
The class should provide a constructor that receives an initial balance, first name, last name, starting date and last account update date and uses it to initialize the data members. The constructor should validate the initial balance to ensure that it is greater than or equal to 0.0. If not, the balance should be set to 0.0 and the constructor should display an error message, indicating that the initial balance was invalid.
The class should provide several member functions. The following is just a list of four. You can add more function as needed.
Member function credit should add an amount to the current balance.
Member function debit should withdraw money from the Account and ensure that the debit amount does not exceed the Accounts balance. If it does, the balance should be left unchanged and the function should print the message "Debit amount exceeded account balance." Either way the last account update date will be changed.
Member function getBalance should return the current balance.
Print function displays customers name, A/C Open date, A/C Update date, and A/C balance.
SavingsAccount class
Derived class SavingsAccount should inherit the functionality of an Account, but also include a data member of type double indicating the interest rate assigned to the Account.
SavingsAccounts constructor should receive the initial balance, first name and last name of a customer, account starting date, last account update date, as well as an initial value for the SavingsAccounts interest rate.
SavingsAccount should have four member functions. You can create more functions when there is a need.
A public member function calculateInterest that returns a double indicating the amount of interest earned by an account. Member function calculateInterest should determine this amount by multiplying the interest rate by the account balance. [Note: SavingsAccount should inherit member functions credit and debit as is without redefining them.]
addInterest function add interest to the balance, update the last update date information and display customer name, interest rate , old balance, new balance information.
Print function prints out Savings Account:, calls the base class print function and display the interest rate information.
setinterestRate function validates the interest rate and set the interest rate to the private data member. If the rate is less than zero, set the rate to zero and display the rate and customer information.
CheckingAccount class
Derived class CheckingAccount should inherit from base class Account and include an additional data member of type double that represents the fee charged per transaction.
CheckingAccounts constructor should receive the initial balance, first name and last name of a customer, account starting date, last account update date, as well as a parameter indicating a fee amount.
Class CheckingAccount should redefine member functions credit and debit so that they subtract the fee from the account balance whenever either transaction is performed successfully. CheckingAccounts versions of these functions should invoke the base-class Account version to perform the updates to an account balance. CheckingAccounts debit function should charge a fee only if money is actually withdrawn (i.e., the debit amount does not exceed the account balance). [Hint: Define Accounts debit function so that it returns a bool indicating whether money was withdrawn. Then use the return value to determine whether a fee should be charged.] If a transaction is a credit there is no transaction fee.
CheckingAccount should have three member functions. You can create more functions when there is a need.
chargeFee function subtracts fee from the account balance , displays the fee charge information, updates the last update date information.
Print function displays Checking Account:, calls base class print function and shows transaction fee information.
settransactionFee function validates the fee and set the fee to the private data member. If the fee is less than zero, set the fee to zero and display the fee and customer information.
Date class
The Date class(attached) is a good start. You need to incorporate this Date class into the Account class as private data members. The Date class needs to have setDate function which provides a tool to update a Date object private data member. It also needs to validate the data.
CISP400V10AD5.cpp
In the CISP400V10AD5.cpp file we need to declare 10 element array (account) pointer points to Account objects.
Then declare some SavingsAccounts and CheckingAccounts object and assign them to the array pointer based on the following schedule and information.
Array element | Object type | Parameters information |
1 | SavingsAccount | ( 25.0 , .03, "Susan", "Baker", (1, 1, 2015), (2,19,2016)) |
2 | SavingsAccount | ( 100 , .04, "Chao", "Zulueta", (5, 31,2016), (2,14,2017)) |
3 | CheckingAccount | ( 80.0, 1.0, "Robert", "Jones", (2,29,2017), (3,19,2017)) |
4 | CheckingAccount | ( 250.0, 2.0, "Kenneth", "Ramirez", (6,6,2016), (1,12,2017) ) |
5 | SavingsAccount | ( 125 , .02, "Vue", "Thomas",(11, 30, 2016), (2,24,2017) ) |
6 | CheckingAccount | ( 180.0, 3.0, "Angela", "Wilma", (2, 27, 2017), (3,19,2017) ) |
7 | SavingsAccount | ( 600 , .04, "Romeo", "Turner", (11,19,2016), (1,19,2017) ) |
8 | SavingsAccount | ( 95 , .02, "Oscar ", "Udonkanga", (10,10,2016), (2,6,2017)) |
9 | CheckingAccount | ( 255.0, 3.0, "Jimmy ", "Untalasco", (7, 32,2016), (7,32,2016)) |
10 | CheckingAccount | ( 80.0, 1.0, "Terrence", "Trocino", (11, 16, 2016), (2,4,2017)) |
Use cout << " ***Initial Accounts information*** "; and for (int i=0; i<10; i++) account[i]->print(); to display the accounts information.
Adjust accounts on 3/25/2017 based on the following schedule, call functions to update the account information:
Array element | Object type | Parameters information |
1 | SavingsAccount | Susan Baker had a $40 debit. |
2 | SavingsAccount | Chao Zulueta had a $30 credit |
3 | CheckingAccount | Robert Jones had a $40 debit. |
4 | CheckingAccount | Kenneth Ramirez had a $50 credit. |
5 | SavingsAccount | Vue Thomas had an accrued interest for a period. |
6 | CheckingAccount | Angela Wilma had a transaction fee charged.\ |
7 | SavingsAccount | Romeo Turner had an accrued interest for a period. |
8 | SavingsAccount | Oscar Udonkanga had a $60 debit.\ |
9 | CheckingAccount | Jimmy Untalasco had a transaction fee charged |
10 | CheckingAccount | Trocino Terrence had a $100 debit. |
Use cout << " ***After the 3/25/2017 update, the Accounts information*** ;" and for (int i=0; i<10; i++) account[i]->print(); to display the accounts information.
On 4/8/2017 all the saving used the same interest rate(0.05) and all the checking used the same transaction fee($5). Use program to decide the object is saving or checking to do the adjustment. No man made adjustment allowed.
Use cout << " ***After the 4/8/2017 update, the Accounts information*** and for (int i=0; i<10; i++) account[i]->print(); to display the accounts information.
The code that is given:
Date.H::::
//Date.h // Date class definition; Member functions defined in Date.cpp #ifndef DATE_H #define DATE_H
class Date { public: static const unsigned int monthsPerYear = 12; // months in a year explicit Date(int = 1, int = 1, int = 1900); // default constructor void print() const; // print date in month/day/year format ~Date(); // provided to confirm destruction order private: unsigned int month; // 1-12 (January-December) unsigned int day; // 1-31 based on month unsigned int year; // any year
// utility function to check if day is proper for month and year unsigned int checkDay(int) const; }; // end class Date
#endif
Date.cpp::::::
// Date.cpp // Date class member-function definitions. #include
// constructor confirms proper value for month; calls // utility function checkDay to confirm proper value for day Date::Date(int mn, int dy, int yr) { if (mn > 0 && mn <= monthsPerYear) // validate the month month = mn; else throw invalid_argument("month must be 1-12");
year = yr; // could validate yr day = checkDay(dy); // validate the day
// output Date object to show when its constructor is called cout << "Date object constructor for date "; print(); cout << endl; } // end Date constructor
// print Date object in form month/day/year void Date::print() const { cout << month << '/' << day << '/' << year; } // end function print
// output Date object to show when its destructor is called Date::~Date() { cout << "Date object destructor for date "; print(); cout << endl; } // end ~Date destructor
// utility function to confirm proper day value based on // month and year; handles leap years, too unsigned int Date::checkDay(int testDay) const { static const array< int, monthsPerYear + 1 > daysPerMonth = { 0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
// determine whether testDay is valid for specified month if (testDay > 0 && testDay <= daysPerMonth[month]) return testDay;
// February 29 check for leap year if (month == 2 && testDay == 29 && (year % 400 == 0 || (year % 4 == 0 && year % 100 != 0))) return testDay;
throw invalid_argument("Invalid day for current month and year"); } // end function checkDay
Step by Step Solution
There are 3 Steps involved in it
Step: 1
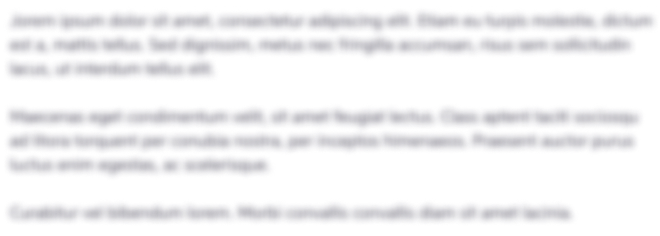
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started