Question
Create this program in C++ In linear algebra, a matrix is an array of numbers that can be used to operate on groups of numbers,
Create this program in C++
In linear algebra, a matrix is an array of numbers that can be used to operate on groups of numbers, such as 2D or 3D coordinate systems. It allows operations to be applied to each coordinate in the system, such as rotating, scaling, and translating. These operations form the basis for graphics engines and scientific computation on large sets of numbers.
A matrix is written as a 2D array of numbers. For example, a matrix containing 4 3-dimensional coordinates could be written as follows, with each coordinate written vertically:
1 4 7 10 2 5 8 11 3 6 9 12
These coordinates can then be manipulated in space usually by multiplying them by another matrix, such as a rotation matrix, which will rotate each coordinate about an axis.
The elements are specified using row i and column j, and for us we will start the indices at 0. If the matrix above were named a, then the element a(2,1) would be 6.
Instructions
Use the attached file Matrices-3.h for the project specification. Store the elements of your matrix in a private 2D vector of doubles. Some of the functions are already done, including an assignment operator to assign a(i,j), an access operator to read a(i,j), and accessors to get the number of rows and columns in the matrix. The following function headers:
double& operator()(int i, int j) const double& operator()(int i, int j) const
act as operators for access and assignment. The operator() syntax allows usage of the form a(i,j) in your code. The first line returns a reference to the double at row i, column j within the private vector so that element can be changed. The second line returns a const reference to the same element so it can be read. Since they are declared from within the class, the compiler will automatically use the calling object for the operation. These operators are done for you.
The rest of the functions you must implement:
- (1 pt) Matrix(int _rows, int _cols)
- Construct a matrix of the specified size
- Initialize each element to 0
- You will want to use the vector resize function here
- (1 pt) Matrix operator+(const Matrix& a, const Matrix& b)
- Add each corresponding element
- Construct a local matrix to store the result and return it
- usage: c = a + b;
- If a and b do not have the same number of rows and columns, throw an error
- Example:
b: 1 0.866025 1 0.5 0 0.5 1 0.866025 c = b + b: 2 1.73205 2 1 0 1 2 1.73205
- (2 pts) Matrix operator*(const Matrix& a, const Matrix& b);
- Perform a matrix multiplication
- This will be the most difficult part of the project. It does NOT consist of just multiplying each corresponding element. You may have to do some research on what this operation is:
- This Khan Academy (Links to an external site.) page explains it well
- Wolfram (Links to an external site.) also has a good, but very concise, description
- This will be the most difficult part of the project. It does NOT consist of just multiplying each corresponding element. You may have to do some research on what this operation is:
- For a matrix a with row i and column j, and a matrix b with row j and column k, the product c of two matrices a and b is defined as:
- c(i,k) = a(i,0) * b(0,k) + a(i,1) * b(1,k) + a(i,2) * b(2,k) + ...
- The elements at row i of matrix a are multiplied and summed with the elements in column k of matrix b, storing the resulting sum in element (i,k) in matrix c.
- This can be done with a triple nested for loop
- Let the outermost loop control k, the column index for b
- Let the loop inside of that control i, the row for a
- The innermost loop will control j, which will determine which column to use from a and which row to use from b.
- Multiply each a(i,j) * b(j,k) and create a running sum.
- When the j loop is finished, store the resulting sum in c(i,k)
- This can be done with a triple nested for loop
- In order for this to work, the number of columns in a have to equal the number of rows in b. If they do not, throw an error.
- Test your calculated results against handwritten examples to make sure it is working
- The elements at row i of matrix a are multiplied and summed with the elements in column k of matrix b, storing the resulting sum in element (i,k) in matrix c.
- Construct a local matrix to store the result and return it
- usage: c = a * b;
- In the example below,
- c(0,0)=a(0,0)*b(0,0)+a(0,1)*b(1,0) = 0*1+(-1)*0 = 0
- c(0,1)=a(0,0)*b(0,1)+a(0,1)*b(1,1) = 0*0.866025+-1*0.5 = -0.5
- c(0,2)=a(0,0)*b(0,2)+a(0,1)*b(1,2) = 0*1+-1*1 = -1
- ...
- c(1,3)=a(1,0)*b(0,3)+a(1,1)*b(1,3) = 1*0.5+0*0.866025=0.5
- Perform a matrix multiplication
a: 0 -1 1 0 b: 1 0.866025 1 0.5 0 0.5 1 0.866025 c = a * b: 0 -0.5 -1 -0.866025 1 0.866025 1 0.5
- (1 pt) bool operator==(const Matrix& a, const Matrix& b)
- If the rows and columns are not equal, return false
- If any element (i,j) does not match, return false
- Otherwise return true
- (1 pt) bool operator!=(const Matrix& a, const Matrix& b)
- Opposite of ==
- (1 pt) ostream& operator
- Output operator
- Output matrices in the format shown above
- Separate columns by ' ' and rows by ' '
- If you want to you can specify the width of your columns using setw from
- (2 pts) Output Test
- Construct matrices a and b in your main.cpp file and output the following operations:
a: 0 -1 1 0 b: 1 0.866025 1 0.5 0 0.5 1 0.866025 c = b + b: 2 1.73205 2 1 0 1 2 1.73205 c = a * b: 0 -0.5 -1 -0.866025 1 0.866025 1 0.5
Scoring
- Your code must compile and link to receive credit
- Put your code in a subfolder called M04:
- main.cpp
- Matrices.h
- Matrices.cpp
- makefile
- Your makefile must will build Matrices.o, main.o, and an executable named M04
- Write the code in Matrices.cpp inside namespace Matrices{...}
- On the Power Server, from the directory with your source file, header file, and makefile, run the following command:
- /home1/hermler/scripts/M04/M04Grader
- This will test your makefile and each operand and give you a score out of 9
- Shortly after the due date I will run the same script to record your score and then upload it to Canvas.
Closing Remarks
- It is interesting to note that a from the example output above is actually a rotation matrix that creates a 90 degree rotation to any set of (x,y) coordinates in a 2D plane.
- b represents a set of coordinates on the unit circle, written vertically as column vectors
- If you graph the original coordinates for b: (1.0,0.0), (sqrt(3) / 2.0, 1.0 / 2.0), (1.0, 1.0), (1.0 / 2.0, sqrt(3) / 2.0), and then graph the coordinates from c = a * b, you will see that they have all rotated 90 degrees counter-clockwise
Step by Step Solution
There are 3 Steps involved in it
Step: 1
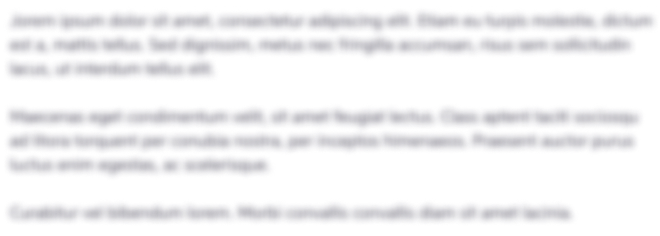
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started