Question
Create three files to submit. ContactNode.h - Struct definition, including the data members and related function declarations ContactNode.c - Related function definitions warmup.c - main()
Create three files to submit.
- ContactNode.h - Struct definition, including the data members and related function declarations
- ContactNode.c - Related function definitions
- warmup.c - main() function
2. The ContactNode struct as well as function declarations for the related functions described below have been provided in ContactNode.h. Please do not modify the provided ContactNode.h file. Details regarding each function as well as expected return values are included in the comments associated with each function declaration in ContactNode.h. The following is a summary of this content:
- Data members
- char contactName[50]
- char contactPhoneNum[50]
- struct ContactNode* nextNodePtr
- Related functions
- ContactNode* CreateContactNode(char nameInit[], char phoneNumInit[])
- Call malloc to allocate space for a ContactNode and return a pointer to the initialized object
- void InsertContactAfter(ContactNode* thisNode, ContactNode* newNode)
- Insert a new node after node
- ContactNode* GetNextContact(ContactNode* thisNode)
- Return location pointed by nextNodePtr
- void PrintContactNode(ContactNode* thisNode)
- Display the contents of this PlaylistNode on stdout using printf
- void DestroyContactNode(ContactNode* thisNode)
- Call free to release all memory dynamically allocated by malloc for the specified node
- ContactNode* CreateContactNode(char nameInit[], char phoneNumInit[])
Ex. of PrintContactNode() output:
Name: Roxanne Hughes Phone number: 443-555-2864
3. In main(), prompt the user for three contacts and output the user's input. Create three ContactNodes and use the nodes to build a linked list. Ex:
Person 1 Enter name: Roxanne Hughes Enter phone number: 443-555-2864 You entered: Roxanne Hughes, 443-555-2864 Person 2 Enter name: Juan Alberto Jr. Enter phone number: 410-555-9385 You entered: Juan Alberto Jr., 410-555-9385 Person 3 Enter name: Rachel Phillips Enter phone number: 310-555-6610 You entered: Rachel Phillips, 310-555-6610
4. Output the linked list. Ex:
CONTACT LIST Name: Roxanne Hughes Phone number: 443-555-2864 Name: Juan Alberto Jr. Phone number: 410-555-9385 Name: Rachel Phillips Phone number: 310-555-6610
ContactNode.h File
#ifndef __CONTACT_NODE_H | |
#define __CONTACT_NODE_H | |
#define CONTACT_FIELD_SIZE 50 | |
struct ContactNode_struct { | |
char contactName[CONTACT_FIELD_SIZE]; | |
char contactPhoneNum[CONTACT_FIELD_SIZE]; | |
struct ContactNode_struct* nextNodePtr; | |
}; | |
typedef struct ContactNode_struct ContactNode; | |
/* CreateContactNode: Allocate a ContactNode object in the heap using malloc | |
* the object will be initialized with the specified name and phone number | |
* and the nextNodePtr field will be set to NULL; | |
* name - NULL terminated string containing contact name | |
* phoneNum - NULL terminated string containing contact phone number | |
* returns - Pointer to ContactNode object allocated on the heap | |
*/ | |
ContactNode* CreateContactNode(char name[], char phoneNumInit[]); | |
/* InsertContactAfter: Insert a new ConactNode into the linked list | |
* immediately after the specified node. | |
* nodeInList - Pointer to the ContactNode that the new ContactNode | |
* should be inserted after in the list | |
* newNode - Pointer to the new ContactNode to be added | |
* returns - 0 on success, -1 on error | |
*/ | |
int InsertContactAfter(ContactNode* nodeInList, ContactNode* newNode); | |
/* GetNextContact: Return a pointer to the ContactNode that immediately | |
* follows the specified node in the list | |
* nodeInList - Pointer to ContactNode that we want to get the next node of | |
* returns - Pointer to next ContactNode, NULL on error or end of list | |
*/ | |
ContactNode* GetNextContact(ContactNode* nodeInList); | |
/* PrintContactNode: Write the fields of the ContactNode, nicely formatted, | |
* to stdout (console) using printf. | |
* thisNode - Pointer to ContactNode object to be displayed */ | |
void PrintContactNode(ContactNode* thisNode); | |
/* DestroyContactNode: Release memory allocated by malloc in the | |
* CreateContactNode function. Does nothing if thisNode is NULL | |
* thisNode - Pointer to ContactNode object to be freed. | |
*/ | |
void DestroyContactNode(ContactNode* thisNode); | |
#endif /* __CONTACT_NODE_H */ |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
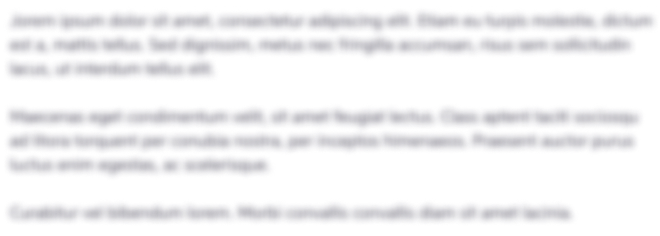
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started