Question
Creating a simple database Java Most of this lab has been written for you, with comments. Read over the code and the comments carefully, so
Creating a simple database Java
Most of this lab has been written for you, with comments. Read over the code and the comments carefully, so that you understand what is being done. Note especially, how the Movie class and the MovieDB class have been set up. Note also the movieFile.txt data file, so you understand how the data is to be formatted. Your task is to complete the sections of code that have been omitted rom the main program so that it works correctly. Files:
Movie.java: Class to represent a Movie. This has already been completed for you, but read the comments carefully.
MovieDB.java: Class to represent a simple database of Movies. This also has been completed for you, but again read it carefully to see how it implements the various operations.
Lab07.java: Main program class. Much of this has also been implemented, but you must complete 3 sections to get it to work. movieFile.txt: Example file used for the program
output.txt: Demo runs to show how program should work
Movie class
// CS 401 Lab 7 Movie class
import java.text.*;
import java.util.*;
public class Movie
{
private String title;
private String director;
private String studio;
private double gross;
// Constructor -- take 4 arguments and make a new Movie
public Movie(String t, String d, String s, double g)
{
title = new String(t);
director = new String(d);
studio = new String(s);
gross = g;
}
// Return a formatted string version of this Movie
public String toString()
{
StringBuffer B = new StringBuffer();
B.append("Title: " + title + " ");
B.append("Director: " + director + " ");
B.append("Studio: " + studio + " ");
NumberFormat formatter = NumberFormat.getCurrencyInstance(Locale.US);
B.append("Gross: " + formatter.format(gross) + " ");
return B.toString();
}
// Return an unformatted string version of this Movie
public String toStringFile()
{
StringBuffer B = new StringBuffer();
B.append(title + " ");
B.append(director + " ");
B.append(studio + " ");
B.append(gross + " ");
return B.toString();
}
// Accessor to return title of this Movie
public String getTitle()
{
return title;
}
}
Movie DB class
// CS 0401 Lab 7 MovieDB class
// This class is a simple database of Movie objects. Note the
// instance variables and methods and read the comments carefully.
public class MovieDB
{
// Note that we have 2 instance variables here -- an array of Movie and
// an int. Since Java arrays are of fixed size once they are created,
// we creating the array of a certain (large) size and then using the
// int variable to keep track of how many actual movies are in it. We
// don't resize here, but we could if we wanted to (as discussed in class).
private Movie [] theMovies;
private int numMovies;
// Initialize this MovieDB
public MovieDB(int size)
{
theMovies = new Movie[size];
numMovies = 0;
}
// Take already created movie and add it to the DB. This is simply putting
// the new movie at the end of the array, and incrementing the int to
// indicate that a new movie has been added. If no room is left in the
// array, indicate that fact.
public void addMovie(Movie m)
{
if (numMovies < theMovies.length)
{
theMovies[numMovies] = m;
numMovies++;
}
else
System.out.println("No room to add movie");
// Alternatively, as we discussed in lecture, we could resize the
// array to make room - feel free to try this as an additional
// exercise.
}
// Iterate through the array until the movie is found or the end of the
// array is reached. Note that even though a Movie object has several
// components we are searching based on the title alone. We call the
// title the "key value" for the Movie. If the Movie is not found we
// indicate that fact by returning null.
public Movie findMovie(String title)
{
for (int i = 0; i < numMovies; i++)
{
if (theMovies[i].getTitle().equals(title))
return theMovies[i];
}
return null;
}
// Return a formatted string containing all of the movies' info. Note
// that we are calling the toString() method for each movie in the DB.
public String toString()
{
StringBuffer B = new StringBuffer();
B.append("Movie List: ");
for (int i = 0; i < numMovies; i++)
B.append(theMovies[i].toString() + " ");
return B.toString();
}
// Similar to the method above, but now we are not formatting the
// string, so we can write the data to the file.
public String toStringFile()
{
StringBuffer B = new StringBuffer();
B.append(numMovies + " ");
for (int i = 0; i < numMovies; i++)
B.append(theMovies[i].toStringFile());
return B.toString();
}
}
Here is an example output:
> java Lab7
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
1
Movie List:
Title: Star Wars
Director: George Lucas
Studio: 20th Century Fox
Gross: $798,000,000.00
Title: Jaws
Director: Steven Spielberg
Studio: Universal
Gross: $470,600,000.00
Title: The Return of the King
Director: Peter Jackson
Studio: New Line
Gross: $1,129,200,000.00
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
2
Movie name?
Spider-Man
Director?
Sam Raimi
Studio?
Sony
Gross?
821600000
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
1
Movie List:
Title: Star Wars
Director: George Lucas
Studio: 20th Century Fox
Gross: $798,000,000.00
Title: Jaws
Director: Steven Spielberg
Studio: Universal
Gross: $470,600,000.00
Title: The Return of the King
Director: Peter Jackson
Studio: New Line
Gross: $1,129,200,000.00
Title: Spider-Man
Director: Sam Raimi
Studio: Sony
Gross: $821,600,000.00
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
3
Movie name?
Jaws
Movie:
Title: Jaws
Director: Steven Spielberg
Studio: Universal
Gross: $470,600,000.00
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
3
Movie name?
Weasel-Man
Weasel-Man was not found
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
4
Good-bye
> java Lab7
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
1
Movie List:
Title: Star Wars
Director: George Lucas
Studio: 20th Century Fox
Gross: $798,000,000.00
Title: Jaws
Director: Steven Spielberg
Studio: Universal
Gross: $470,600,000.00
Title: The Return of the King
Director: Peter Jackson
Studio: New Line
Gross: $1,129,200,000.00
Title: Spider-Man
Director: Sam Raimi
Studio: Sony
Gross: $821,600,000.00
Enter your choice:
1. List movies
2. Add new movie
3. Find movie
4. Quit
4
Good-bye
> cat movieFile.txt
4
Star Wars
George Lucas
20th Century Fox
7.98E8
Jaws
Steven Spielberg
Universal
4.706E8
The Return of the King
Peter Jackson
New Line
1.1292E9
Spider-Man
Sam Raimi
Sony
8.216E8
Step by Step Solution
There are 3 Steps involved in it
Step: 1
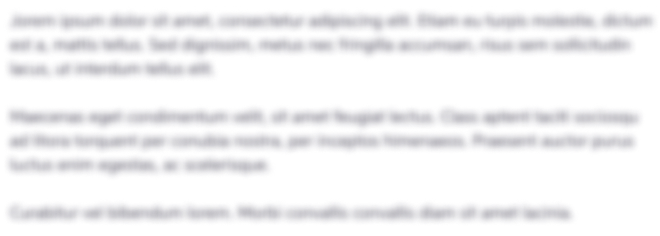
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started