Question
CS 1336.005 Assignment 4 1 Assignment 4 (Parts 1 and 2) Ifs, and switchs, and loops, oh my! (Actually, only one switch) Your fourth programming
CS 1336.005 Assignment 4 1 Assignment 4 (Parts 1 and 2) Ifs, and switchs, and loops, oh my! (Actually, only one switch) Your fourth programming assignment will focus on the use of the switch statement and loops that we covered in chapters 4 and 5. You will also be using a file. We will also be making use of the other C++ language constructs you have used in past assignments, including the if statement and functions. In part 2 you will be writing to an output file. The program will display a menu with various options as follows: Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of current total 7 Current total to the power x All data used by the functions must: ? Be internal to the function (declared and only used inside the function) or passed to the function via parameters ? Be returned back from the function via the return statement ? Not be global variables. No global variables can be used by your program unless the variables are defined as const values (that is, they cannot be changed). The function descriptions below tell you the details about what parameters are passed to the function and what values are returned back from the functions. You must adhere to this design or you will have points taken off from your grade. Required functions: void displayMenu() The menu must be displayed with a function called displayMenu. This function returns a void value (no return value) and takes no input parameters. It does not prompt the user for any values, it simply displays the contents of the menu. CS 1336.005 Assignment 4 2 unsigned int getCommand() You program must also have a function called getCommand. This function also takes no input parameters. The function returns an unsigned int value, the command entered by the application user. The getCommand function will call the displayMenu function and will then input a menu value from the application user via cin. The getCommand function must then check to make sure the input value is a valid menu command. If the value you read in is not a valid menu item, your getCommand function needs to redisplay the menu and read in a new value. Function getCommand needs to continue to display the menu and read in a new value until the user has entered in a valid menu item. To do this data validation of the input value entered, your getCommand function will have to use a loop. double processCommand(unsigned int command, double oldValue) The next required function is the processCommand function. It will use a switch statement to process the various commands. The commands are the non-zero commands entered in by the application user. The quit command will be processed in the main function. For the add menu item, the appropriate case in your switch statement will have to prompt for the value to be added. The value will be added to the oldValue to create the newValue. The oldValue is passed to the function via a parameter. The newValue is returned back to the main function via the return statement. For the subtract menu item, the appropriate case in your switch statement will have to prompt for the value to be subtracted. The value will be subtracted from the oldValue to create the newValue. The oldValue is passed to the function via a parameter. The newValue is returned back to the main function via the return statement. For the multiply menu item, the appropriate case in your switch statement will have to prompt for the value to be multiplied. The value will be multiplied with the oldValue and to create the newValue. The oldValue is passed to the function via a parameter. The newValue is returned back to the main function via the return statement. The processing for the divide menu item involves more work. In the appropriate case in your switch statement you will have to prompt for the value to be divided into oldValue. You must check this input value and make sure it is not zero. If it is zero you will display the following message: Division by zero is not allowed. When the input value is zero your processCommand function will return back the passed in oldValue via the return statement. If the input value is not zero (it can be either positive or negative) you program will divide oldValue by the input value to create the newValue. Your function will then return the newValue back via the return statement. CS 1336.005 Assignment 4 3 For the square root command you will take the oldValue passed to your processCommand function and take the square root of that value. The square root will be the value for newValue and will be returned back to main from your processCommand function via the return statement. You will need to use the sqrt function that is talked about in chapter 3, section 3.9, in your book. For the power command you will have to prompt the user for a new input value. You will then raise the oldValue to the input value to create the newValue returned by your function. You will need to use the pow function that is covered on pages 95 96 In chapter 3 of your book. Finally, your program must also process the clear menu item. In this case, your function should return back 0 to the calling function via the return statement. int main() You main function will have the main processing loop, but will not do much work beyond calling the getCommand and processCommand functions. Here is the pseudo-code for your main: Define the total variable. This is must contain a number with a fractional part. You need set this value to zero. Do Display the current value of total (see the sample output below) Call the getCommand function and save the returned command value If the command value is not quit Call the processCommand function. This will return a new value for total. Make sure you pass the command and current total value are parameters to the function End if While (command value is not quit) Note that your main is fairly simple. Most of the processing is done by the functions. A question for you. How many times will the menu be processed? Zero or more times or one or more times? That will help you decide what type of loop is most appropriate. Here is sample output from one run of the program. Note that input values are in bold. NOTE: Your output does not have to exactly match the output shown here, but your output MUST contain the equivalent information. CS 1336.005 Assignment 4 4 Total: 0 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 1 Enter the value to be added: 12.5 Total: 12.5 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 2 Enter the value to be subtracted: -2.3 Total: 14.8 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 3 Enter the value to be multiplied: .998 Total: 14.7704 CS 1336.005 Assignment 4 5 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 4 Enter the value of the divisor: 12.6 Total: 1.17225 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 4 Enter the value of the divisor: 0 Division by zero is not allowed. Total: 1.17225 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 5 Total: 0 Enter one of the following commands: 0 Quit the application 1 Add CS 1336.005 Assignment 4 6 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x -1 The menu item you have entered is invalid. Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 8 The menu item you have entered is invalid. Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 1 Enter the value to be added: 144 Total: 144 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 6 CS 1336.005 Assignment 4 7 Total: 12 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 7 Enter the value for x: 3 Total: 1728 Enter one of the following commands: 0 Quit the application 1 Add 2 Subtract 3 Multiply 4 Divide 5 Clear total 6 Square root of the current total 7 Current total to the power of x 0 Grading for Part 1 (80 points): Name your file Assignment4Part1.cpp. 10% of the grade for part 1 will for comments you have in your program, for having meaningful variable names, and for formatting your code as is shown in the C++ text book. 40% of the grade is for the program itself. It must perform the actions defined in the assignment. 40% of the grade is for having all of the required functions with the required signatures. This includes the stipulation that you do NOT use any global variables in your program. The only exception is that you can use const global variables. 10% of the grade is for having the correct formatting of the output as per the sample output shown throughout the assignment description. CS 1336.005 Assignment 4 8 Grading and directions for part 2 (20 points): For part 2 you are going to write out all commands to an output file in addition to the output you send to the console. When you perform an operation you are going to output two lines to the output file. The first line will be the operation and any operand value. Then second line will be the total. At the very start of the file you need to output the current total (0). See below for the output format of the file. The output file should be called calculator.txt. You should not output anything for an invalid command value. Name your file Assignment4Part2.cpp. You do not need to upload the calculator.txt file. Here is the output file for the program run starting on page 3. total 0 add 12.5 total 12.5 subtract -2.3 total 14.8 multiply 0.998 total 14.7704 divide 12.6 total 1.17225 divide 0 not allowed total 1.17225 clear total 0 add 144 total 144 sqrt total 12 pow 3 total 1728 quit You cannot just open, write, and close the file in the processCommand function. When you open up a file for output it will overwrite what was there and you will only get the output from the last transaction. Also, you are opening and closing the file multiple times and that is very inefficient. Finally, you are not allowed to open the output file with append and do an open and close of the file within the processCommand function. You MUST only open the output file once. To ensure you only open the output file once, the main function will need to create the ofstream, open the output file and write the original total to the output file. If the file cannot be opened the program needs to output an error message to cout and quit all processing. The main function will also need to pass the open ofstream to the processCommand function. CS 1336.005 Assignment 4 9 The signature of the processCommand function needs to change to: double processCommand(unsigned int command, double oldValue, ofstream &outputFile) Note that ofstream is being passed by reference. This is required. You cannot pass an ofstream by value, you will get errors at compile time if you try. The processCommand function will now have the output file stream and can write the correct output to the file. Once the user has specified that you are quitting, the function needs to output quit to the output file. Your main function should then close the output file. If your processCommand function calls other functions you may have to pass the ofstream to those functions as well. Summary of new processing: Your program needs to open a file for output and make sure it has been successfully opened. For the output file contents shown on page 8, the first line of output: total 0 is output by the main processing before any commands are executed. For the add processing your program will output: add 12.5 total 12.5 The subtract processing will output: subtract -2.3 total 14.8 This will continue for the multiply, divide, clear, sqrt and pow processing. The last pow process, for example, will output: pow 3 total 1728 Finally, after the quit command has been entered by the user the program will output: quit to the output file and close the output file. Let me know if you have any questions.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
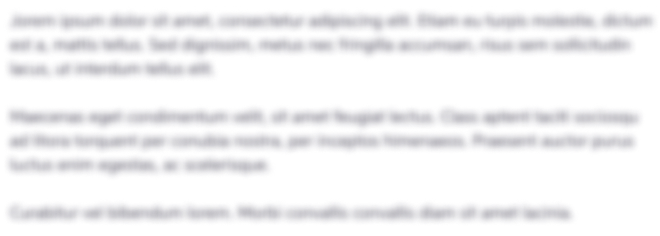
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started