Question
CS 145 Computer Science II Lab 02: More Java and Exceptions Goals After completing the lab, students should be able to: Create if statements to
CS 145 Computer Science II
Lab 02: More Java and Exceptions
Goals
After completing the lab, students should be able to:
Create if statements to select the code that is run.
Write loops to process data.
Create and use arrays to store data.
Use exceptions to indicate problems in the code.
Use try-catch blocks to correctly handle exceptions.
Process the data in files as input.
Resources
Use all of the resources available to you. These may include (in no particular order):
The book
Videos and slides on Eclipse (under How-To Eclipse)
The slides on commenting (under Unit 1 Module 1)
Videos on JavaDoc comments (under How-To JavaDoc Comments)
Videos and slides on zipping/unzipping files (under How-To Zipping Files)
Activities
This lab has you create a more complex set of classes using ifs, loops and arrays. It also introduces exceptions and file i/o. The classes themselves are small but they have a good amount of functionality to them. Think carefully about how the classes should work and how you can implement that functionality.
Lab Instructions
For this lab, you will be creating a list of accounts and the balances associated with those accounts. You will be able to find the total amount due, the amount due to customers as a refund and individual accounts by the account number. The account data is stored in a file, so the code should be able to read the data from a file and store it for processing.
You will be creating 3 classes:
AccountBalance stores an account number and the associated balance
AccountList stores a list of accounts and provides access to that data and summary information
AccountFileIO Loads a data file and produces an AccountList
Comments
You are required to comment all of your code. This includes a JavaDoc comment for the class and for each method in the code. The information in the comments should be complete, accurate, and useful. Ensure that all required tags are present. Refer to the notes on commenting and the guidelines posted under Useful Information -> Resources and under How-To -> JavaDoc Comments for detailed information about what is needed.
Testing
You are required to test all of your code using JUnit tests. This means you should have 100 % code coverage and cover all paths. Think carefully about where problems could be and write tests that specifically address those issues. Try multiple values but make sure that they are not testing the same thing. That is, do not just use positive numbers. Try negative numbers and zero as well.
You can see your code coverage in Eclipse too. Use the Run -> Coverage Last Launched option or the run button (arrow in a green circle) with a red/green bar next to it to launch the code coverage. It will highlight the code that was tested or not tested in your program.
AccountBalance
1. Create a package called uwstout.cs145.labs.lab02.
2. In the package create a class called AccountBalance.
a. The AccountBalance class associates an account number and the balance on the account. It also provides information about whether the account is due a refund or not.
3. Create class variables for the class. The account number is text and the balance is a double.
4. Create a constructor for the class. It should take (in order) the account number (String) and the current balance (double).
If the account number is null or if the account number is not exactly 8 characters in length, the constructor should throw an IllegalArgumentException.
5. Create getters called getAccountNumber and getAmount.
6. Create a method called needsRefund that returns a boolean and takes no parameters. The method should return true if the accounts balance is -25.00 or less. Otherwise, return false.
AccountList
1. Create a class called AccountList in the uwstout.cs145.labs.lab02 package.
a. This class will store multiple AccountBalance objects and be able to perform some calculations on them.
2. Create class variables for the class. It needs to have an array of AccountBalance objects and the current number of objects in the array (an int).
3. Create a constructor for the class. It should take no parameters. In the constructor, set the class variables. The array should have an initial size of 3 (use a constant). What should the current number of objects in the array be?
4. Create a method called getSize that returns an int and has no parameters. Return the number of objects in the array (that is not the same as the length of the array). This should be one line of code.
5. Create a method called getAccount. It should return an AccountBalance object and take an int (the position of the object in the array) as a parameter. If the parameter is valid (i.e. it is a valid position in the used portion of the array), return the AccountBalance at that position.
If the parameter is not valid, throw an IllegalArgumentException.
6. Create a method called add that returns nothing and takes an account number (String) and the account balance (double) as parameters.
Using the parameters, create an AccountBalance object.
Add the AccountBalance object to the first open position in the array and increment the number of objects in the array. (You dont need a loop to do this; based on the information in the class, how can you calculate the first empty position?)
If the array is full, grow the array by creating a new, larger array and copying the existing data into the new array. Then set the classs variable to use the newer array. The array should grow by 2 (use a constant) each time it needs to grow.
If the account number is invalid, this method should throw an IllegalArgumentException.
7. Create a method called getTotal that returns a double. Loop over all of the AccountBalances in the array and return the sum of the balances.
8. Create a method called getRefundTotal that returns a double. Loop over all of the AccountBalances in the array. If a refund needs to be given (since the balance is -25.00 or less), then add the balance to the sum. Return the sum.
9. Create a method called findBalance that returns an AccountBalance and takes an account id (String) as a parameter. Loop over all of the AccountBalances in the array. If one of the account numbers equals the parameter, return that AccountBalance. (Be careful how you compare the Strings.)
If parameter matches none of the account numbers, return null.
10. Test your class thoroughly.
Testing arrays can seem difficult, but it follows the same patterns youve used so far. Create an object, call methods and check the results. You can, in each test method, add the values directly to the list and then check the results. An approach as shown below may be more convenient in many cases. The example tests the add method, but a similar approach could be used to test getTotal, getRefundTotal, etc. Something like this would also work for testing the labs third class too.
private static final AccountBalance[] VALUES = {
new AccountBalance("ABC-1234", 56.70),
new AccountBalance("DEG-8534", 110.10),
new AccountBalance("BRS-3777", 10.10),
new AccountBalance("MLI-6484", 69.55),
new AccountBalance("UTC-7515", -25.00),
new AccountBalance("QEX-1987", 100.75),
new AccountBalance("TEF-3844", -6.75),
new AccountBalance("OUH-9765", 9.10),
new AccountBalance("TEF-3548", 451.65),
new AccountBalance("POG-7557", -50.15)
};
private static final double DELTA = 0.0001;
@Test
public void testAdd() {
AccountList list = new AccountList();
//fill list with data
for (int i = 0; i < VALUES.length; i++) {
list.add(VALUES[i].getAccountNumber(),
VALUES[i].getAmount());
}
//check if the list was built correctly
assertEquals(VALUES.length, list.getSize());
for (int i = 0; i < list.getSize(); i++) {
assertEquals(VALUES[i].getAccountNumber(),
list.getAccount(i).getAccountNumber());
assertEquals(VALUES[i].getAmount(),
list.getAccount(i).getAmount(), DELTA);
}
}
AccountFileIO
1. Create a class called AccountFileIO in the uwstout.cs145.labs.lab02 package. (This is a capital i then a capital o for input/output.)
a. This class will read data from a file and add it to an AccountList.
2. This class needs no class variables or constructors.
3. Create a method called parseFile that returns an AccountList and takes a file name (String) as a parameter.
CS 145 Computer Science II
This method will read in an input file a line at a time and add any valid account information to an AccountList. The file is formatted so that each line is a separate account. Each line has the format:
The account numbers do not have spaces in them but there is a space between the account number and the balance. See below for an example.
The input files may have errors in them. If a line is incomplete or otherwise corrupted, then it should not be added to the list, but any remaining lines should be processed.
If a line has too much information (i.e. it has values after the balance), any additional information should be ignored.
Create an AccountList that will store the data read in.
Use a Scanner to read from a file with the given file name (the parameter). For each line of input, read in the full line (check the Scanner class to see how to read in an entire line at one time).
With each line, create a new Scanner (use a different variable from the Scanner above) and use that new Scanner to read in the account number and balance. If they are successfully read in, add it to the list.
If the file cannot be opened, then the method should return null.
try-catch blocks will make this easy to do.
Dividing this method into a couple of separate methods may make this easier to do.
4. The AccountFileIO class can be tested by creating a set of files with data expected, calling parseFile and then checking to see if the AccountList returned has the data from the file.
When creating a test file, put it in the project directory and not the package directory. If it is in the project directory, you should be able to do open the file with just the file name.
myFileIO.parseFile("test1.txt");
If it is not in the project directory, you will need to supply a path to the file.
Sample input:
DHH-2180 110.25
TOB-6851 -258.45
JNO-1438 375.95 CS 145 Computer Science II
IPO-5410 834.15
PWV-5792 793.00
Make sure you code is formatter, documented and tested before you submit.
Notices, hints and suggestions
Commenting and Formatting
Make sure you format your code before you submit.
o Ctrl + Shift + F will do most of it for you automatically in Eclipse.
Ensure that your JavaDoc comments are there and that all the required tags are there too.
o You need a class comment for each class and a method comment for each method.
o You do not need a lot of depth when documenting the test class and methods (i.e. This tests the add method. is fine).
Everything will be uploaded through Canvas
Submission
1. Show running code to SI either in class lab or later in SI tutor lab
2. SI will determine how much you completed
Grading
1. The Instructor will provide a grade later based on how much was completed
Step by Step Solution
There are 3 Steps involved in it
Step: 1
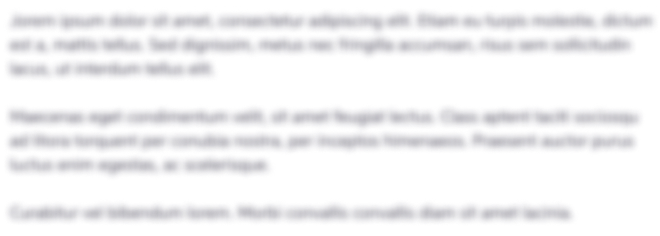
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started