Question
CSC 121 Lab 6 Functions Using Value Parameters Write a program in a file named Payroll2.cpp that reads and processes each line of input. Each
CSC 121
Lab 6
Functions Using Value Parameters
Write a program in a file named Payroll2.cpp that reads and processes each line of input. Each line of input starts with a string with an embedded blank that should be interpreted as an employee name. After the name, the input line contains a single pound (#) character followed by 3 double values. These should be interpreted as an employees hourly salary, the number of hours that the employee has worked and the tax rate as a percentage that is used for that employee. After inputting the remaining data on the input line, your program should display formatted values of the input values followed by the employees gross salary (hours hourly, with time and one-half for the number of hours beyond 40) and the net salary (the gross salary minus the gross salary the tax rate/100). After the last line is input, a pound character is input (#) without entering any name and your program should then display the total of all of the gross salaries and the total of all of the net salaries. For the best results use input redirection to read from a file instead of having the user input from the keyboard. A file is provided (payroll.txt) that you can download from Lab 6 Files in the Course Laboratories folder under Course Material on Blackboard. All monetary amounts should be right justified and formatted to display 2 digits to the right of the decimal point. All output for this program should be displayed on the monitor and should be embedded within functions. The file Payroll1.cpp contains functions instructions() and reportTitle() that display a descriptive message and the column headings, respectively. In addition, the program in Payroll2.cpp should use functions displayEmployeeInfo() to display the salary information for each employee and the function totalAmounts() to display the total_gross and total_net.
Variables (no global variables allowed):
name, hourly, hours, rate Employee name (contains embedded blanks), hourly rate,
hours worked and tax rate
net,gross net and gross income for employee
total_net, total_gross total net and total gross income (accumulation variables)
Input:
John Smith#10.45 40 15
Jane Doe#12.50 45 15
Harry Morgan#20.00 40 20
Carmen Martinez#25.00 35 25
Jacintha Washington#50.85 60 35
#
Read employee name (ending with #), hourly rate, hours worked and tax rate from standard input (cin) with a # character without any employee name on the last line to mark the end of input.
Since the name contains embedded blanks, we can use a form of the getline() function to read the input for us. This function comes in a couple of versions and the one that were interested in accepts 3 parameters.
getline(cin, string, char) Reads a string from cin terminated by a character with the value of the char parameter. All characters on the line up to but not including the first instance of char are stored in the string.
Output:
Display on the monitor the input values in addition to the gross pay and net pay using the function displayEmployeeInfo()
Display on the monitor the total_gross pay and the total_net pay using the function totalAmounts()
Step 0: Download Payroll1.cpp from Blackboard page Located in the Lab 6 Files folder in Course Materials->Course Laboratories.
Step 1: Initialize accumulators (total_gross, total_net) to 0 Set the double variables total_gross and total_net equal to 0.0.
Step 2: Display a descriptive message Invoke the function instructions()
Step 3: Display the column headings Invoke the function reportTitle()
Step 4: Read in the name on the first line This will be the invocation of the 3-parameter getline() function that is described above. The # character terminates the name string.
Step 5: Test if end of input is reached This is the while statement that loops on the condition that name.length() is greater than zero. The next 9 steps of the algorithm should be embedded within this loop.
Step 6: Input the remaining doubles from the current input line Use the extraction operator (>>) to read hourly, hours and rate (in this exact order)
Step 7: Set cin beyond the current item This needs to use the cin.ignore() function that expects 2 arguments. The first argument is the number of spaces to scan ahead. The second argument is the char value to stop the scanning process and acknowledge that as the end of the line. The following statement would work in this instance: cin.ignore(100, );
Step 8 - 10: Calculate gross pay This is a selection statement that deals with the possibility that the employee worked overtime (more than 40 hours). If their hours variable is 40, then their gross is set to 40 their hourly pay rate plus the number of hours that they worked beyond 40 their hourly pay rate 1.5 (time and one-half for overtime). When the hours < 40, the gross is the hourly pay scale the hours worked.
Step 11: Calculate net pay The net pay is the gross pay minus the tax rate divided by 100.0 the gross pay.
Step 12: Display info for this employee Invoke displayEmployeeInfo() making sure that the order of the arguments in the function invocation is in agreement with the parameters in the function implementation.
Step 13: Update accumulation variables These assignment statements merely increase the value of total_gross by the gross and the value of the total_net by the net.
Step 14: Attempt to read another input line This line is no different than the line that was written for Step 4. This is the final statement that is embedded within the loop that was started at Step 5.
Step 15: Display the totals of the gross and net incomes Invoke function totalAmounts().
C++ Source File: Payroll1.cpp Download from Lab 6 Files in Course Materials->Course Laboratories on Blackboard.
Compiling the program (Using the GNU C++ compiler): g++ Payroll2.cpp o Payroll2 (on mars.harpercollege.edu: CPP Payroll2)
Redirection of input to read from a file In the Lab 6 Files folder in Course Materials->Course Laboratories on Blackboard there is a file called payroll.txt that contains example input. To use payroll.txt as input instead of using the keyboard for input, cin can be redirected to read from the file payroll.txt by running the program with the following command on mars.harpercollege.edu (or any other Unix/Linux environment): ./Payroll2.out < payroll.txt (assuming the compiled program file name is Payroll2.out) The command prompt in Windows uses the same syntax for input redirection: Payroll2.exe < payroll.txt
Turning in Lab 6:
Submit Payroll2.cpp (source file) to Lab 6 in Assignments on Blackboard. Binary/executable files will not be graded, the source file (.cpp) must be submitted.
Payroll1.cpp
/************************************************************************
* Name: Your name CSC 121
* Date: Today's date Lab 6
*************************************************************************
* Statement: Displays headings for a payroll report.
* Specifications:
* Input - none
* Output - Payroll report headings
************************************************************************/
//header files I/O, formatting, strings
#include
#include
#include
using namespace std;
// function prototypes
// instructions()- describes the program usage to the user
void instructions();
// reportTitle() displays the payroll report titles in columnar format
void reportTitle();
// displayEmployeeInfo() displays the information for each employee
// totalAmounts() displays the total gross and net amounts
int main()
{
// 1) initialize accumulaton variables
// 2) display a descriptive message
instructions();
// 3) display column headings
reportTitle();
// 4) attempt to input the name on the first input line
// 5) test that name contains at least one character
// 6) input the remaining doubles on the input line
// 7) set input beyond the current line
// 8) test hours >= 40
// 9) calculate gross & overtime
// 10) else calculate gross without overtime
// 11) calculate net pay
// 12) display info for this employee
// 13) update accumulation variables
// 14) attempt to input next name
// 15) display totals
}
// instructions() describes the program usage to the user
void instructions()
{
// display program instructions
cout << "This payroll program calculates an individual "
<< "employee pay and company totals "
<< "using data from a data file payroll.txt. "
<< " A payroll report showing payroll information "
<< " is displayed. ";
}
// reportTitle() displays the payroll report titles in columnar format
void reportTitle()
{
// set program formatting
cout << setprecision(2) << fixed << showpoint << left;
// display report titles
cout << setw(20) << "Employee" << setw(10) << "Hourly"
<< setw(10) << "Hours" << setw(10) << "Tax" << setw(10)
<< "Gross" << setw(10) << "Net" << endl;
cout << setw(20) << "Name" << setw(10) << "Rate"
<< setw(10) << "Worked" << setw(10) << "Rate" << setw(10)
<< "Amount" << setw(10) <<"Amount" << endl << endl;
}
// displayEmployeeInfor() displays payroll information for an employee
// totalAmounts() displays total gross and total net for the input file
Payroll.txt
John Smith#10.45 40 15 Jane Doe#12.50 45 15 Harry Morgan#20.00 40 20 Carmen Martinez#25.00 35 25 Jacintha Washington#50.85 60 35 #
Step by Step Solution
There are 3 Steps involved in it
Step: 1
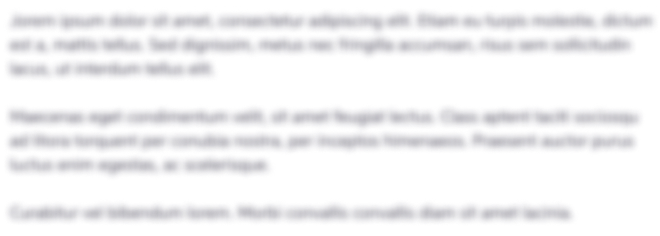
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started