Question
CSC 143 Programming Project Iterators / Sets / Maps Created byProf. Hu Last The goals for this part of the assignment are tolearn about using
CSC 143 Programming Project Iterators / Sets / Maps Created byProf. Hu Last The goals for this part of the assignment are tolearn about using Iterators, Sets and Maps. Iterators – iteratethrough a map’s keySet, Sets – build a set, use map keySet, use setmethods. Maps – build a map, iterate and process, use keySetoperations to affect map. There are other Java Collections typesnot presented in the book such as Map.Entry, and you are notallowed to use them. You may only use material presented up toChapter 11 in the book. Remember to add Javadoc class headers, andmethod headers (JavaDoc if lots of parameters, otherwise a simplecomment may suffice). I’ve asked for a few specific comments, butyou’re responsible for proper commenting & documentation ofanything not obvious and labeling all important code blocks likepseudocode. Put all comments including answers to questionsimmediately above the relevant code. Don’t just put them all intothe method header. It’s usually better to comment before you writecode than to comment afterwards when you’ve likely forgotten whatwas important in figuring it out. This is a real-life example frommy experience as webmaster of a club. We use an Excel spreadsheetto keep our membership database. We also use Evite to send outmonthly meeting invites. Unfortunately, Evite gets “out-of-sync”with the Excel sheet. So occasionally I had to scan through andmanually mark up new addresses to add, and old addresses to delete.So, I wrote a program to do this comparison for me. The inputs arethe 2 CSV files (excel.csv and evite.csv), and the output is a listof actions I need to take. Your program should reconcile these twofiles to generate evites for the members if they are not already inthe evite file, and, remove evites for the members if those membersare not in the members file. 1. Create a function calledreadNamesMap(String filename) that reads the members from a CSVfiles and generates a sorted Map with . The email is used as a keybecause emails are unique. Make sure to declare any return types orvariable types as the abstract form (List, Map) but when you createit you must create a subclass (ArrayList, HashMap). 2. Add a callin the method PartB() to call the readNamesMap (“Excel.csv”) andstore what is being returned as “excelMap”. Print “Read %d namesfrom Excel Membership List” and verify 100 names read. 3. Add acall to PartB() to call readNamesMap (“Evite.csv”) and store thatas “eviteMap”. Print “Read %d names from Excel Membership List” andverify 101 names read. 4. Use Iterator & manually generateEvite adds 4.1. You need to generate a list of new members who needto be added to Evite. To do this, use a Java Iterator and iterateevery item in excelMap. Review the syntax to iterate over a map’skeySet in the book. Recall that Iterators are parameterized (need<>) and you need to iterate over the keys of the map – emailnames. Intelligently name all variables. 4.2. You should alwaysstore the result of your iterator as a local variable of thatparameterized type. In this case, you want to name your variablesomething like keyExcel. This makes the code equivalent to afor-each loop except the prohibition on modification is removed.CSC 143 Programming Project Iterators / Sets / Maps 4.3. In thepast, we’ve looped through each member of a collection to see ifit’s in there. But that’s very inefficient. What method on the Setclass can check if a key is in it? Even better, what method on theMap class can check if a key is in its keySet? Use either method tocheck if the Excel email is in the Evite email keyset. This iswhere the choice of a TreeSet or HashSet comes into play. A HashSetcan lookup a string without having to search at all, whereas aTreeSet requires searching proportional to the depth of therequired tree. In this case, we don’t need to iterate all the itemsin the Set in alphabetical order. A Hash table’s quick lookup isactually better. We’ll learn more about Binary Tree efficiencylater in the book. If it isn’t in it then you need to add this nameto Evite. Create a new ArrayList of Persons at the beginning ofpartB which will hold all the changes that need to be made. Thisarray will be used for both this & the next section – onlycreate one. Add this person to the changes list. Use the member’sfirst & last name. But for the email please add “+Evite” to theend. At the end of partB() print out each item in the changes liston its own line. Verify these two people need to be added to Evite.Person [firstName=Louisa, lastName=Cronauer,email=+E*louisa@cronauer.com+Evite] Person [firstName=Alease,lastName=Buemi, email=+E*alease@buemi.com+Evite] 5. Use Setoperations to generate Evite removes 5.1. Using an iterator was abit of extra work with the while loop and was no advantage since wedidn’t need to remove anything. There’s a much simpler way. We canuse set operations on the maps to do the work for us. If you wantto know what email names need to be removed from Evite, you canstart with Evite’s keyset, and removeAll the keyset of Excel.What’s left must be old members who dropped and need to be removed.One big caveat is that Set operations (union, intersection,difference) performed on a map’s keySet actually affect theunderlying Map. Java refers to this as “the Set is backed by theMap”. If you don’t operate on a copy of the keyset you’ll actuallydestroy the original map. Note that in this case the Excel keysetwon’t be modified, but Evite keyset will. To prevent fromdestroying Evite’s keyset, make a copy first. Here’s an example ofhow to do this using the set constructor which can take a set. SetnewSet = new HashSet(oldMap.keySet()); Add this person to thechanges list. Use the member’s first & last name. But for theemail please add “- Evite” to the end. Verify these people need tobe removed from Evite. Person [firstName=Oretha, lastName=Menter,email=-E*oretha_menter@yahoo.com-Evite] Person [firstName=Ty,lastName=Smith, email=-E*tsmith@aol.com-Evite] Person[firstName=Kris, lastName=Marrier, email=-E*kris@mail.com-Evite]CSC 143 Programming Project Iterators / Sets / Maps 6. At thebottom of partB() add a call to testPartB() passing in your newchange List. If you have any errors, double check things Did youread the right number of items from the source CSV files? Did youfind the removes/adds? If not, use the debugger to see why not. Didyou add the Person to the changes list? Did you make sure to addthe right suffix such as “+Evite”? 7. Submit your project
Step by Step Solution
There are 3 Steps involved in it
Step: 1
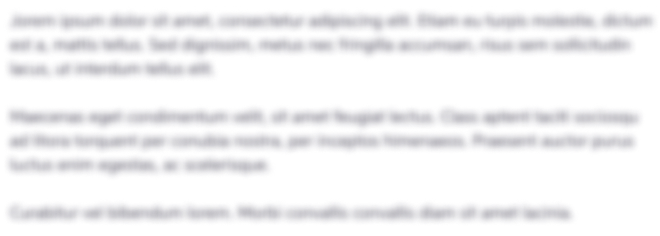
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started