Answered step by step
Verified Expert Solution
Question
1 Approved Answer
CSC 2 5 6 Final Project An ancient robot game For this project, you are given a program that implements a game where a human
CSC Final Project An ancient robot game For this project, you are given a program that implements a game where a human user tries to escape from four robots. The user and four robots are on an xy grid. On every step, you enter a move for the human. The robots will attempt to get closer to the human. When a robot has the same xy coordinates as the human, the game is over. You will translate this program faithfully, following all function call guidelines and MIPS register use conventions. Two arrays x and y keep track of the x and ycoordinates of four robots. The positions of the human and the four robots are initialized in the program. On each step, the user enters a move; the positions of the human and the robots are updated. This continues until the human dies. This figure gives an idea of the game; well work with a textonly version, sorry. In the main loop, the user is prompted to enter a move. The position of the user is updated. Then the program calls a function moveRobots to update the position of the robots as they try to catch the human. The new positions of the human and the robots are then displayed. The function moveRobots has prototype int moveRobotsint arg int arg int arg int arg arg is the base address of array that contains the xcoordinates of the four robots, arg is the base address of array that contains the ycoordinates of the four robots, arg is the x coordinate of the human, arg is the ycoordinate of the human. moveRobotsupdates the positions of the four robots, and returns a if the human is alive, and a if the human is dead ie the human has the same coordinates as a robot Each coordinate of a robot is updated by calling the function getNew which returns the new coordinate based on the current coordinate of the robot and the current coordinate of the human. When you translate moveRobots to MIPS assembly language, arg through arg are in $a through $a; the return value is in $v The function getNew uses simple rules to move a robot closer to the human. If the difference in the coordinates is the robot's coordinate will move units closer to the human. If the difference in the coordinates is the robot's coordinate will move one unit closer to the human. See program listings. getNew has prototype int getNewint arg int arg arg is the coordinate x or y of a robot, arg is the coordinate x or y of the human. getNew returns the new coordinate of the robot, based on the position of the human. The function getNew is already translated to MIPS assembly language, arg and arg are in $a and $a respectively, and the return value is in $v A copy of the C program robots.cpp can be found on Canvas. The file robots.asm contains the main program and getnew already translated into MIPS assembly language. Your functions will follows the main program in the same file. Write the functions exactly as described in this handout. Do not implement the program using other algorithms or tricks. Do not even switch the order of the arguments in function calls; you must follow the order specified in the C code. The purpose of this program is to test whether you understand nested functions. If you wish to make changes to the algorithm, you must first check with the instructor. Your functions should be properly commented. Each function must have its own header block, including the prototype of the function, the locations of all arguments and return values, descriptions of the arguments and how they are passed, and a description of what the function does. Paste in the C code as inline comments for your MIPS assembly code. Refer to the Programming Style handout from Chapter slides from Canvas for guidelines on how to comment your code. You should try to make your code efficient. For example, your loops should follow the efficient form presented in class. Points will be deducted for obvious inefficiencies. Submission: submit your code via Canvas. All your code should be in a single plain text file. of your grade is for correctness. is for claritydocumentation Output Your coordinates: Enter move for x for x for y for y: Your coordinates: Robot at Robot at Robot at Robot at Enter move for x for x for y for y: Your coordinates: Robot at Robot at Robot at Robot at Enter move for x for x for y for y: Your coordinates: Robot at Robot at Robot at Robot at Enter move for x for x for y for y: Your coordinates: Robot at Robot at Robot at Robot at Enter move for x for x for y for y: Your coordinates: Robot at Robot at Robot at Robot at AAAARRRRGHHHHH... Game over libra
CSC Final Project
An ancient robot game
For this project, you are given a program that implements a game where a human user
tries to escape from four robots. The user and four robots are on an xy grid. On every
step, you enter a move for the human. The robots will attempt to get closer to the human.
When a robot has the same xy coordinates as the human, the game is over. You will
translate this program faithfully, following all function call guidelines and MIPS register
use conventions.
Two arrays x and y keep track of the x and ycoordinates of four robots. The
positions of the human and the four robots are initialized in the program. On each step,
the user enters a move; the positions of the human and the robots are updated. This
continues until the human dies. This figure gives an idea of the game; well work with a
textonly version, sorry.
In the main loop, the user is prompted to enter a move. The position of the user is
updated. Then the program calls a function moveRobots to update the position of
the robots as they try to catch the human. The new positions of the human and the robots
are then displayed.
The function moveRobots has prototype
int moveRobotsint arg int arg int arg int arg
arg is the base address of array that contains the xcoordinates of the four robots, arg is
the base address of array that contains the ycoordinates of the four robots, arg is the x
coordinate of the human, arg is the ycoordinate of the human. moveRobotsupdates the positions of the four robots, and returns a if the human is alive, and a if the human
is dead ie the human has the same coordinates as a robot Each coordinate of a robot
is updated by calling the function getNew which returns the new coordinate based
on the current coordinate of the robot and the current coordinate of the human.
When you translate moveRobots to MIPS assembly language, arg through arg
are in $a through $a; the return value is in $v
The function getNew uses simple rules to move a robot closer to the human. If the
difference in the coordinates is the robot's coordinate will move units closer to
the human. If the difference in the coordinates is the robot's coordinate will
move one unit closer to the human. See program listings. getNew has prototype
int getNewint arg int arg
arg is the coordinate x or y of a robot, arg is the coordinate x or y of the human.
getNew returns the new coordinate of the robot, based on the position of the human.
The function getNew is already translated to MIPS assembly language, arg and arg
are in $a and $a respectively, and the return value is in $v
A copy of the C program robots.cpp can be found on Canvas. The file robots.asm
contains the main program and getnew already translated into MIPS assembly
language. Your functions will follows the main program in the same file.
Write the functions exactly as described in this handout. Do not implement the
program using other algorithms or tricks. Do not even switch the order of the arguments
in function calls; you must follow the order specified in the C code. The purpose of
this program is to test whether you understand nested functions. If you wish to make
changes to the algorithm, you must first check with the instructor.
Your functions should be properly commented. Each function must have its own header
block, including the prototype of the function, the locations of all arguments and return
values, descriptions of the arguments and how they are passed, and a description of what
the function does. Paste in the C code as inline comments for your MIPS assembly
code. Refer to the Programming Style handout from Chapter slides from Canvas for
guidelines on how to comment your code.
You should try to make your code efficient. For example, your loops should follow the
efficient form presented in class. Points will be deducted for obvious inefficiencies.
Submission: submit your code via Canvas. All your code should be in a single plain text
file.
of your grade is for correctness. is for claritydocumentation
Output
Your coordinates:
Enter move for x for x for y for y:
Your coordinates:
Robot at
Robot at
Robot at
Robot at
Enter move for x for x for y for y:
Your coordinates:
Robot at
Robot at
Robot at
Robot at
Enter move for x for x for y for y:
Your coordinates:
Robot at
Robot at
Robot at
Robot at
Enter move for x for x for y for y:
Your coordinates:
Robot at
Robot at
Robot at
Robot at
Enter move for x for x for y for y:
Your coordinates:
Robot at
Robot at
Robot at
Robot at
AAAARRRRGHHHHH... Game over
libra
Step by Step Solution
There are 3 Steps involved in it
Step: 1
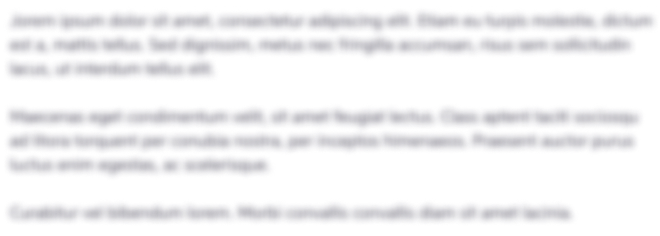
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started