Question
CSC 241 Lab 4 Given the following files (see Extra Files): IncreaseFactorial.java FactorialCounter.java TestFactorial.java Complete the parallelized version of the factorial method (n!). The multipleIncrement
CSC 241 Lab 4 Given the following files (see Extra Files): IncreaseFactorial.java FactorialCounter.java TestFactorial.java Complete the parallelized version of the factorial method (n!). The multipleIncrement method of the FactorialCounter class needs to be completed such that it uses the increment method. The result needs to be computed before it is returned from the factorialParallel method of TestFactorial class. Answer the following questions: 1) Remove the comments within the run method of the IncreaseFactorial class and observe the different threads executing with their various hold values. Do all four threads get to work all of the time? 2) Update the code to use only two threads. Does it run faster? Why, or why not? 3) Why does the parallelized method run slower than the serial version?
Need to code in Java.
TestFactorial:
//---------------------------------------------------------------------- // TestFactorial.java by Dale/Joyce/Weems Chapter 3 // // Repeatedly prompts user for a non-negative integer n. // Outputs n! calculated recursively and iteratively. // Uses a command line interface. //---------------------------------------------------------------------- package ch03.apps; import ch04.queues.*; import ch04.threads.*;
import java.util.Scanner;
public class TestFactorial { public static float factorialParallel(int n) throws InterruptedException{ float result; FactorialCounter c1 = new FactorialCounter(); FactorialCounter c2 = new FactorialCounter(); FactorialCounter c3 = new FactorialCounter(); FactorialCounter c4 = new FactorialCounter(); QueueInterface
t1.start(); t2.start(); t3.start(); t4.start(); t1.join(); t2.join(); t3.join(); t4.join(); //compute result here.
return result; } public static float factorialParallelIdea(int n){ float result = 1; //Thread1 for(int i = n; i > (n - (n/4)); i--) result *= i; System.out.println("result for 100 to 76 = " + result); //Thread2 for(int i = n - (n/4); i > (n - (n/2)); i--) result *= i; System.out.println("result for 75 to 51 = " + result);
//Thread3 for(int i = n - (n/2); i > (n - (3*n/4)); i--) result *= i; System.out.println("result for 50 to 26 = " + result);
//Thread4 for(int i = n - (3*n/4); i > 0; i--) result *= i; System.out.println("result for 25 to 1 = " + result);
return result; } public static float factorialParallelRec(int n){ float result = 1; result = factorial(n) / factorial(3*n/4); System.out.println("result for 100 to 76 = " + result);
result *= factorial(3*n/4) / factorial(n/2); System.out.println("result for 75 to 51 = " + result);
result *= factorial(n/2) / factorial(n/4); System.out.println("result for 50 to 26 = " + result);
result *= factorial(n/4); System.out.println("result for 25 to 1 = " + result);
return result; } private static float factorial(int n) // Precondition: n is nonnegative // // Returns the value of "n!" { if (n == 0) return (float) 1.0; else return (n * factorial (n - 1)); } public static float factorial2(int n) // Precondition: n is nonnegative // // Returns the value of retValue: n! { float retValue = 1; while (n != 0) { retValue = retValue * n; n = n - 1; } return(retValue); } public static void main(String[] args) throws InterruptedException { Scanner scan = new Scanner(System.in); System.out.println("Factorial calculation test: "); int n = 0; long timeStart, timeEnd; while (n >= 0) { System.out.print(" Enter n (-1 to stop) > "); n = scan.nextInt(); if (n >= 0) { System.out.println(n + "! is"); System.out.println("\t recursive " + factorial(n)); System.out.println("\t iterative " + factorial2(n)); timeStart = System.nanoTime(); String s = String.format("%.0f", factorialParallelIdea(n)); timeEnd = System.nanoTime() - timeStart; System.out.println("\t iterative parallel idea " + s + " total time = " + timeEnd); timeStart = System.nanoTime(); s = String.format("%.0f", factorialParallel(n)); timeEnd = System.nanoTime() - timeStart; System.out.println("\t iterative parallel " + s + " total time = " + timeEnd); System.out.println("\t iterative parallel recursive " + factorialParallelRec(n)); } } } }
Increase Factorial:
package ch04.threads;
import ch04.queues.QueueInterface;
public class IncreaseFactorial implements Runnable { private FactorialCounter c; private QueueInterface
Factorial Counter:
package ch04.threads;
public class FactorialCounter { private float count; public FactorialCounter() { count = 1; }
public synchronized void increment() { count++; }
public synchronized void multipleIncrement(int times){ //complete this code } public synchronized float getCount(){return count;} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
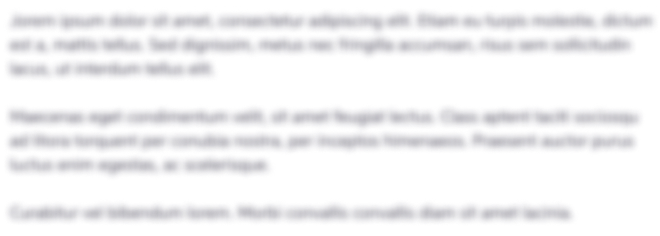
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started