Question
CSC S&E Purpose: To create a Javadoc and JUnit Tests for the methods in a Line class built from scratch. Lab 01 Exercise Topics Internal
CSC S&E
Purpose: To create a Javadoc and JUnit Tests for the methods in a Line class built from scratch.
Lab 01 Exercise
Topics
Internal view of object. HTML files JUnit Testing
Exercise
Creating a Line class. Creating a Javadoc. Test of Line Class.
Component Name
Line Line LineTest
package
plane tests
In the L1_ObjectLab folder store: o theLineclassintheplanepackage. o the JUnit test LineTest in the tests package.
The Javadoc should be stored in the following directory:
/Documents/CSC_143/W19/Labs/JavaDocs/ObjectLab A. ExerciseI:CreatingaLineClass
Write a class called Line that represents a line segment between two Point(s). Your Line objects should have the following :
1. datafields:Theseinternalvariableshaveprivateaccessibility. i. p1 : represents one location in coordinate space.
ii. p2 : represents another location in coordinate space. 2. constructors: The overloaded constructors will construct and initialize a
Line object in coordinate space with the values specified:
-
The first constructor is the default constructor of the Line class. It calls
the third constructor, setting the values to (0, 0, 1, 1).
public Line()
-
The second constructs a new Line with the two given end Point(s).
public Line(Point p1, Point p2)
-
The third constructs a new Line that contains the given two Point
objects specified with the values (x1,y1) and (x2,y2) respectively.
public Line(int x1, int y1, int x2, int y2)
3. mutators:Thesemethodsmodifythevaluesofthedatafieldsp1,p2.
-
setEndPoints : This method sets the end point location of a Line
object.
public void setEndPoints(Point p1, Point p2)
-
translate: This method translates each end points x-value by dx along the x-axis and each end points y-value by dy along the y-axis, giving it a resulting location at (x1 + dx, y1 + dy, x2 + dx, y2 + dy)
public void translate(int dx, int dy)
4. accessors:Thesearemethodsthatretrievestheinformationcontainedwithin the data. Similar to read-only operations.
-
getP1 : This method returns the Line first end point. public Point getP1()
-
getP2 : This method returns the Line second end point. public Point getP2()
-
isCollinear : This method returns true if the given Point is Collinear with the Point(s) of the Line. If the slope of each pair of points is the same for each pair, then the points are collinear. Note that this fails if the points have the same x coordinates, so you will have to handle this as a special case in your code. In addition, you may want to round all slope values to a reasonable accuracy, such as four decimal places past the decimal point, since the double type is imprecise.
public boolean isCollinear(Point p)
-
magnitude: This method returns the magnitude or length of the Line.
The magnitude of a line is equal to ( (x2 -x1)2 + (y2- y1)2 ). public double magnitude()
-
slope : This method returns the slope of the Line. The slope of a line between two points (x1, y1) and (x2, y2) is equal to (y2- y1)/(x2-x1). If x2 equals x1 the denominator is zero (0) and the slope is undefined, so this method must throw an exception in this case.
public double slope()
vi. toString : This method returns a string representation of the Line object, such as [(1, 2) , (6, 8)] .
public String toString()
B. ExerciseII:UMLDiagramfortheLineClass Create a UML diagram for the internal view of the Line class below.
C. ExerciseIII:CreateaJavadocfortheLineClass Create a Javadoc for the Line class. Show the instructor, when you have
successfully created the html file. Use the following directory:
/Documents/CSC_143/W19/Labs/JavaDocs/ObjectLab
Line |
Data Fields |
Constructors Methods |
D. ExerciseIV:CreateaJUnitTestfortheLineClass
Create a JUnit Test for the Line class called LineTest in your test package. Note: Lab I contains, an example of how the JUnit test for the Line class was created.
-
Rightclickonyourtestpackage,andselectNew>JUnitTestCase
Note: You can also get here from the File option in the top menu.
-
SelecttheNewJUnitJupitertest.
-
MakesurethecorrectsourcefolderislistedandnamethetestLineTest.
-
ChecktheGeneratecommentsboxandselectNext.
-
SelecttheconstructorsandmethodsoftheLineclass.ThenpressFinish.
-
AltertheJavadoccommentstomeetcoursestandards.
Things to Note: Exceptions
To test for exception, use annotation @Test with attribute expected = ExceptionClassName. e.g. @Test(expected = IllegalArgumentException.class) public void testMethod(){
vRef.divbyZerO(3, 0); //expect an IllegalArgumentException }
Boolean
To test for booleans, with assertTrue. public void testIsCollinear(){
assertTrue(line.isCollinear(p3)); }
package plane;
/****************************************************************** *
A point representing a location (x, y) in coordinate space, * specified in integer precision. ******************************************************************/
public class Point {
/*********************************************** * data fields * stores the (x, y) location of point ***********************************************/ private int x; private int y; /***************************************************************** * constructs and initializes a point at the origin (0,0) * of the coordinate plane. *****************************************************************/ public Point() { this(0,0); // calls constructor Point(int, int) } /****************************************************************** * constructs and initializes the point to the location specified * by point p. * @param p reference to the specified point object. ******************************************************************/ public Point(Point p) { setLocation(p); // reduce redundancy with setLocation } /***************************************************************** * constructs and initializes the point to the specified (x,y) * location. * @param x the x coordinate of point to construct. * @param y the y coordinate of point to construct. *****************************************************************/ public Point(int x, int y) { setLocation(x, y); // reduce redundancy with setLocation } /******************************************************************** * returns the x coordinate of the point in integer precision. * @return x the x coordinate of point object. ********************************************************************/ public int getX() { return x; } /******************************************************************** * returns the y coordinate of the point in integer precision. * @return y the y coordinate of point object. ********************************************************************/ public int getY() { return y; } /********************************************************************** * moves the point to the specified location in the (x, y) coordinate * plane. This method is identical to setLocation(int, int). * @param x the specified x coordinate value. * @param y the specified y coordinate value. **********************************************************************/ public void move(int x, int y) { this.x = x; this.y = y; } /********************************************************************* * changes the location of the point in the coordinate plane to the * location specified by point p. * @param p the specified point . *********************************************************************/ public void setLocation(Point p) { x = p.x; y = p.y; } /********************************************************************* * changes the location of the point in the coordinate plane to the * location specified by the values (x, y). * @param x the specified x coordinate value. * @param y the specified y coordinate value. *********************************************************************/ public void setLocation(int x, int y) { this.x = x; this.y = y; } /******************************************************************** * returns string representation of point. * @return point (x, y) coordinates. ********************************************************************/ public String toString() { return "(" + x + ", " + y + ")"; } /******************************************************************** * translates the point at the location (x,y) by dx along the * x-axis and dy along the y-axis, resulting with the point at * location (x + dx, y + dy). * @param dx the specified x coordinate value. * @param dy the specified y coordinate value. ********************************************************************/ public void translate(int dx, int dy) { x += dx; // x = x + dx y += dy; // y = y + dy } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
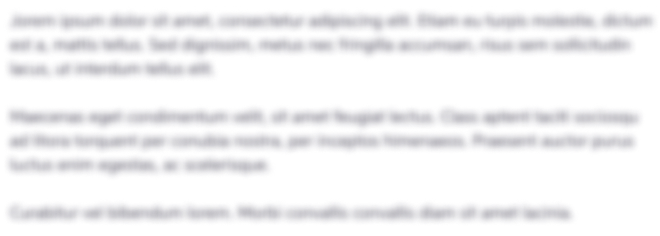
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started