Question
CSCI 240 This program will test the effect of rounding dollar amounts to the nearest $10 rather than keeping track of exact amounts. It has
CSCI 240
This program will test the effect of rounding dollar amounts to the nearest $10 rather than keeping track of exact amounts. It has been determined that if the difference between the results of the two methods (keeping track of exact values and keeping track of rounded values) is less than 2%, then it would be cost effective for a company to round rather than balance to the penny.
The program will accept dollar amounts from the user until 0 is entered. Each exact dollar amount is then rounded to the nearest $10 value. The exact and rounded amounts are then used to keep track of:
the sum of the exact dollar amounts
the sum of the rounded dollar amounts
the sum of the squares of the exact dollar amounts
the sum of the squares of the rounded dollar amounts
the number of dollar amounts entered
After the user has entered an exact amount of 0, the sum, average, and standard deviation for both the exact and rounded amounts are displayed. The percent difference between the sums of the exact and rounded amounts is calculated and a determination is made as to whether or not it's cost effective to round the dollar values.
The Functions
The following functions have been written:
double getExact()
This function will get an exact dollar amount from the user and verify that it is not negative.
It takes no arguments and returns a double: an exact dollar amount.
The function prompts the user to enter an exact dollar amount. The amount entered by the user is then tested to make sure that the user entered a value that is not negative. If the amount entered is invalid, an error message that includes the invalid amount is displayed and the user is prompted for a new amount. This happens repeatedly until a valid amount is entered. Once a valid amount has been entered, it is returned
double roundAmount( double amount )
This function will round a value to the nearest $10 value.
It takes one argument: a double that holds the amount to be rounded. It returns a double: the rounded amount.
It rounds the exact dollar amount by:
1. adding 5 to the passed in amount
2. dividing the sum by 10
3. multiplying the integer result of the division by 10.
double calcAverage( double sum, int count )
This function will calculate an average.
It takes two arguments: a double that holds a sum of values and an integer that holds the number of values used to calculate the sum. It returns a double: the calculated average or -1.
If the average can be calculated (there is no possibility of division by 0 or a negative number), the function uses the passed in sum and number of values to calculate and return the average. If the average cannot be calculated, the function returns -1.
double calcStdDev(double sum, double sumOfSquares, int count)
This function will calculate a standard deviation.
It takes three arguments: a double that represents a sum of dollar amounts, a double that represents the sum of the squared dollar amounts, and an integer that represents the number of dollar amounts. It returns a double: the calculated standard deviation or -1.
If the standard deviation can be calculated (there is no possibility of division by 0 or a negative number), the function uses the passed in sum, sum of squared dollar amounts, and number of dollar amounts to calculate and return the standard deviation. If the standard deviation cannot be calculated, the function returns -1.
The formula for standard deviation is:
where
( x2 ) is the sum of squared dollar amounts (sumOfSquares)
( x)2 is the square of the sum of dollar amounts (sum * sum)
n is the number of dollar amounts
double calcDiff( double exactSum, double roundedSum )
This function will calculate a percent difference.
It takes two arguments: a double that holds the sum of the exact values, and a double that holds the sum of the rounded values. It returns a double: the calculated percent difference or -1.
If the percent difference can be calculated (there is no possibility of division by 0 or a negative number), the function uses the passed in sum of the exact values and sum of the rounded values to calculate and return the percent difference. If the percent difference cannot be calculated, the function returns -1.
The formula to calculate the percent difference:
The fabs() function is used to find the absolute value.
The Blanks
There are 8 blanks in int main() that need to be filled in to successfully complete this part of Program 6. Each blank is indicated by a documentation box similar to:
//*********************************************************************
//*
//* Fill In The Blank 1:
//*
//* Get the first EXACT dollar amount from the user by calling the getExact
//* function. The value returned from the function should be saved in
//* the exactAmt variable.
//* //*********************************************************************
This box should be followed by a call to the getExact function that is described above.
Program Requirements
1. DO NOT WRITE ANY FUNCTIONS. Simply go through the program that has been provided and put in function calls where indicated.
2. The double results will be displayed with exactly 2 digits after the decimal point, including zeros.
3. Make sure to fill in the documentation box at the top of the C++ source code.
4. Hand in a copy of the source code (CPP file) using Blackboard.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
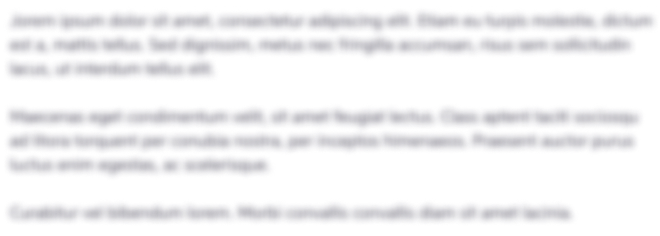
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started