Question
(CSCI 365) Project One: A Binary Number Calculator Objectives: Demonstrate basic programming logic Demonstrate how to convert between positive integers and their binary representation Demonstrate
(CSCI 365) Project One: A Binary Number Calculator
Objectives:
Demonstrate basic programming logic
Demonstrate how to convert between positive integers and their binary representation
Demonstrate how to add two binary numbers
Problem Description:
Write a C/C++ program that simulate a menu based binary number calculator. This calculate shall have the following three functionalities:
Covert a binary string to corresponding positive integers
Convert a positive integer to its binary representation
Add two binary numbers, both numbers are represented as a string of 0s and 1s
To reduce student work load, a start file CSCIProjOneHandout.cpp is given. In this file, the structure of the program has been established. The students only need to implement the following three functions:
int binary_to_decimal(string b);
// precondition: b is a string that consists of only 0s and 1s
// postcondition: the positive decimal integer that is represented by b
string decimal_to_binary(int n);
// precondition: n is a positive integer
// postcondition: ns binary representation is returned as a string of 0s and 1s
string add_binaries(string b1, string b2);
// precondition: b1 and b2 are strings that consists of 0s and 1s, i.e. b1 and b2 are binary
// representations of two positive integers
// postcondition: the sum of b1 and b2 is returned. For instance, if b1 = 11, b2 = 01, // then the return value is 100
The following functionality has been provided:
main function which presents the execution logic of the whole program
void menu(); which display the menu of this binary calculator
bool isBinary(string s); which returns true if the given string s consists of only 0s and 1s; false otherwise
int grade(); which returns an integer that represents the students grade of this projects.
bool test_binary_to_decimal() which returns true if the students implementation of binary_to_decimal function is correct; false otherwise
bool test_decimal_to_binary() which returns true if the students implementation of decimal_to_binary function is correct; false otherwise
bool test_add_binaries which returns true if the students implementation of add_binaries function is correct; false otherwise
Work Process:
Student shall download the CSCI365ProjOneHandout.cpp file, saved as YourNameCSCI365ProjOne.cpp, and add it to his/her C++ project. Please notice, at this point, your project shall be able to run. However, your project will not pass any test. i.e. if you choose option 4 from menu, it will shows that you get 0 out of 10.
Student shall NOT change any name of the declared functions or the given implemented functions. Student shall only implement the bodies of three functions that need to be implemented.
Please see sample run at the end of this file.
Due Date:
It is specified in syllabus and is announced on Blackboard
Sample Run: The highlighted ones are users input
******************************
* Menu *
* 1. Binary to Decimal *
* 2. Decinal to Binary *
* 3. Add two Binaries *
* 4. Grade *
* 5. Quit *
******************************
Enter you choice: 1
Enter a binary string: 101101
Its decimal value is: 45
******************************
* Menu *
* 1. Binary to Decimal *
* 2. Decinal to Binary *
* 3. Add two Binaries *
* 4. Grade *
* 5. Quit *
******************************
Enter you choice: 2
Enter a positive integer: 45
Its binary representation is: 101101
******************************
* Menu *
* 1. Binary to Decimal *
* 2. Decinal to Binary *
* 3. Add two Binaries *
* 4. Grade *
* 5. Quit *
******************************
Enter you choice: 3
Enter two binary numbers, separated by white space: 11 101
The sum is: 1000
******************************
* Menu *
* 1. Binary to Decimal *
* 2. Decinal to Binary *
* 3. Add two Binaries *
* 4. Grade *
* 5. Quit *
******************************
Enter you choice: 4
binary_to_decimal function passed the test
decimal_to_binary function passed the test
add_binaries function passed the test
If you turn in your project on blackboard now, you will get 8 out of 10
Your instructor will decide if one-two more points will be added or not based on your program style, such as good comments (1 points) and good efficiency (1 point)
******************************
* Menu *
* 1. Binary to Decimal *
* 2. Decinal to Binary *
* 3. Add two Binaries *
* 4. Grade *
* 5. Quit *
******************************
Enter you choice: 5
Thanks for using binary calculator program. Good-bye
Program ended with exit code: 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
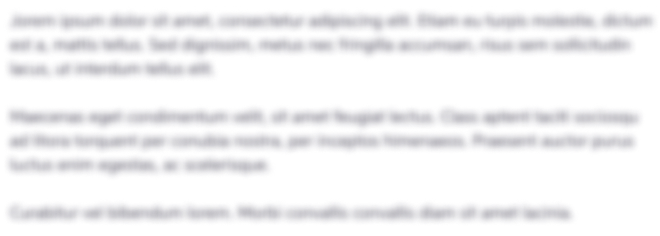
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started