Question
CSE 271 Program assignment 2 Create a class that represents a rational number according to these specifications: Here is a list of responsibilities for the
CSE 271 Program assignment 2
Create a class that represents a rational number according to these specifications:
Here is a list of responsibilities for the rational class:
- Know the value of the denominator.
- Know the value of the numerator.
- Be able to compute the negation of a rational number.
- Be able to compute the reciprocal of a rational number.
- Be able to compute the sum of two rational numbers.
- Be able to compute the difference of two rational numbers.
- Be able to compute the result of multiplying two rational numbers.
- Be able to compute the result of dividing two rational numbers.
- Be able to compute a printable representation of the rational number.
What instance data fields will the Rational class need to implement these responsibilities? What are appropriate names for the instance data?
- We need a numerator and a denominator for the rational number
- Good names would be numerator, denominator
Are there any constraints on the values of the data fields?
- We should not allow the denominator to be zero
- We should store the Rational number in normalized form. That means if the constructor in the client/tester program has arguments 2 and 4 for the numerator and denominator respectively, the actual numerator should be 1 and the denominator should be 2. (There is a text file with some starter code to help you with this more details later in this document)
Here is a list of constructors and methods that implement the responsibilities. Some include pre-conditions, post-conditions, and test cases/data for the Rational class tester. Notice that several methods return a Rational object.
Rational()
Pre-condition: none.
Post-condition: The rational number 1 has been constructed.
Test cases: none.
This constructor will set the numerator and denominator to 1
Rational(n, d)
Pre-condition: The denominator d is non-zero.
Post-condition: The rational number n/d has been constructed and is in normal form.
Test cases:
n = 2, d = 4; result is 1/2
n = 0, d = 7; result is 0/1
n = 12, d =30; result is 2/5
n = 4, d = 0; result should throw an exception
This constructor will set the numerator and denominator to parameters n and d respectively. If the parameter d is zero, throw an Exception. The ZeroDenominatorException class is provided. Refer to notes and examples from class (Module 2). You will also normalize the Rational number. Note the test cases; when numerator is 2 and denominator is 4, we store n as 1 and d as 2.
int getNumerator()
Pre-condition: The rational n/d is in a valid state.
Post-condition: The value n is returned.
Test cases:
n/d is 1/2; result is 1
n/d is 0/1; result is 0
n/d is 2/5; result is 2
This is the accessor method for numerator n
int getDenominator()
Pre-condition: The rational n/d is in a valid state.
Post-condition: The value d is returned
Test cases:
n/d is 1/2; result is 2
n/d is 0/1; result is 1
n/d is 2/5; result is 5
This is the accessor method for denominator d
Rational negate()
Pre-condition: The rational n/d is in a valid state.
Post-condition: The rational number n/d has been returned.
Test cases:
n/d is 1/2; result is Rational(-1,2)
n/d is 0/1; result is Rational(0,1)
n/d is 2/5; result is Rational(2,5)
Rational reciprocal()
Pre-condition: The rational n/d is in a valid state.
Post-condition: The rational number d/n has been returned.
Test cases:
n/d is 1/2; result is Rational(2,1)
n/d is 0/1; result is Rational(1,0) // this is returned, but can not be used in any calculations
n/d is 2/5; result is Rational(5,-2)
Rational add(Rational other)
Pre-condition: The rational n/d is in a valid state and other is the valid rational x/y.
Post-condition: The rational number (ny+xd)/dy has been returned.
Test cases:
n/d is 1/2, x/y is 1/2; result is 1/1
n/d is 1/2, x/y is 1/6; result is 2/3
n/d is 3/4, x/y is 5/6; result is 19/12
n/d is 1/3, x/y is 2/3; result is 1/3
Rational subtract(Rational other)
Pre-condition: The rational n/d is in a valid state and other is the valid rational x/y.
Post-condition: The rational number (ny-xd)/dy has been returned.
Test cases: left for you to determine
Rational multiply(Rational other)
Pre-condition: The rational n/d is in a valid state and other is the valid rational x/y.
Post-condition: left for you to determine
Test cases: left for you to determine
Rational divide(Rational other)
Pre-condition: The rational n/d is in a valid state and other is the valid rational x/y.
Post-condition: left for you to determine
Test cases: left for you to determine
String toString()overrides toString()
Pre-condition: The rational n/d is in a valid state.
Post-condition: The string n/d has been returned.
Test cases:
n/d is 1/2; result is 1/2
n/d is 0/1; result is 0/1
n/d is 2/5; result is 2/5
You should implement all of the above methods and write a tester class that will test every method.
Requirements and suggestions
- Documentation of all methods is required as discussed in the programming guidelines. The ID block at the top of the program is required
- Since exceptions are new, you will find the class ZeroDenominatorException is provided. You can simply use this if statement in the constructor. Then none of the fractions will have a zero denominator. (You will need to implement try-catch exception in your tester)
if(denominator == 0)
throw new ZeroDenominatorException("Can not have a zero denominator");
- There are two private methods provided for you in a file named normalize.txt. You can use them into your Rational class. private int gcd(int a, int b) to calculate the greatest common denominator. This method is only used by normalize private void normalize() to normalize the fraction; call this in the constructor Rational(n,d)
- In the tester/client class, you use the try-catch block. See example code from class.
- Notice there are getter methods but no setter methods.
- Notice that some methods return a Rational object. See Money example from class.
- Remember to document each method completely, as well as include the ID block at the top of the program.
- Be sure to test every method in Rational. Start with constructors and the toString, the getters, then the operations.
Submit: A zipped file of three java files.
Your Rational class - Rational.java
Your tester class - RationalTester.java
And the provided ZeroDenomimatorException.java
Do not zip any additional files with your program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
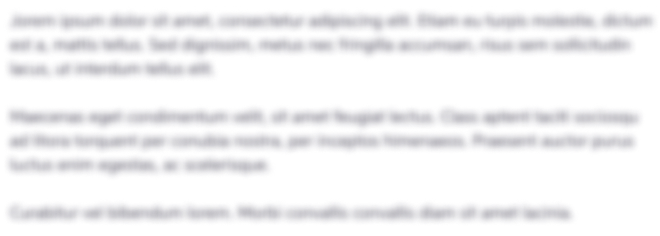
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started