Answered step by step
Verified Expert Solution
Question
1 Approved Answer
CST8132 Object-Oriented Programming Lab 4: Banking System I Due Date: Check Brightspace for the due date. Marks: 20 marks (worth 5% of term mark)
















CST8132 Object-Oriented Programming Lab 4: Banking System I Due Date: Check Brightspace for the due date. Marks: 20 marks (worth 5% of term mark) Demo: Demo your code and output to your lab professor during your own lab hours. Recommended Reading: Chapters 7-10 of Deitel and Deitel, Java How to Program book Exercise In this lab, we will create a few classes to represent a Bank system. A bank has various account holders in their system, and these account holders can have a Savings account or Chequing account. In this lab, we are developing a system that represents this scenario. We are creating a few classes namely Person, Account, Chequing, Savings, and Bank, along with the Driver class. This project also has an interface that stores some constants like a fee for the Chequing account ($13.50) and a yearly interest rate for the Savings account (3.99 %). All personal attributes like first name, last name, email, phone number should be in the Person class. Account class should have an account number attribute, accHolder which is a Person object, and a balance that is a double attribute that represents the balance amount (by having a Person object in Account class, we are implementing Composition). As we know that account can be Chequing or Savings account, we need to create two classes - Chequing and Savings, both extends Account class (As we are extending classes, we are using inheritance). The balance of both types of accounts will be updated based on the fee or the interest rate. The Bank class will manipulate the bank accounts. When you create classes, think about encapsulation, and make sure that you are using the correct access specifier. Also, you can have only one Scanner which is declared in the main method. You have to pass that Scanner object to all the methods that require a Scanner object (instead of creating multiple Scanner objects in various classes and methods). Make sure that your code will not crash at any point (except InputMismatch). Use conditions as required. As the first step, you need to create the following classes: Person class: should have personal attributes, required constructors, and a method to read personal information Account class: abstract class. Should have instance variables to represent account number, the account holder (Person object), and the balance. You should define the required constructors. There should be methods to read relevant information and to display the details. The balance amount that you read from the user cannot be negative. Also, you should have a method to update the balance. As you have 2 different types of accounts that do the balance update based on their own set rules, you cannot properly define the method to update the balance while you are in this class, but you need to define it as an abstract method here. Chequing class: This is a specific type of account that has a specific fee. This fee is stored in the Policies interface. Every month, when you run the monthly process, this fee amount will be deducted while updating the balance. Savings class: This is a specific type of account that has an interest rate (yearly interest rate is stored in the interface). While updating the balance, add the monthly interest to the balance. Bank class: This class should have a name (String) which is the name of the bank, and an ArrayList named accounts, which is an ArrayList of Account objects as instance variables. Constructors should be defined as required. There should be a parameterized constructor that gets the name of the bank and size, which is the number of account holders as parameters. This constructor sets the name of the Bank and creates the ArrayList of accounts with the given size (name and size will be read in main(), and will be sent here when creating the Bank object). You should define methods to read an account, run the monthly process (during which the balances will be updated) for all accounts, and print all existing accounts. You should also define some static methods to print the title, print stars, etc. Bank Test class This is the driver class (test class), which means this class has the main method. Main method This method read the name of the bank (example: "Spectrum") and the number of accounts (stored in num). Keep in mind that this number cannot be negative or 0. A Bank object will be created with the name and num. Display a menu. A few things that you need to care about: While printing the title, see that it is printed in uppercase even if the user entered it in lowercase or camelCase. In the output, we can see that even though we created the ArrayList with a capacity of 3, when we added the fourth account, it didn't crash, but the ArrayList increased its capacity. The methods that you need to override in subclasses should have the same signatures. Grading Scheme Item Person class Account class Chequing class Savings class Bank class Policies interface BankTest class (main method) Comments & Javadoc comments UML Test Plan Marks Required 1 1 1 2 2 2 3 3 5 I Submission Zip your code, JavaDoc, UML diagram, and test plan in a folder named. Lab4.zip and submit it to Brightspace before the due date. Demonstrate your work to your lab professor during your own lab hours. Be ready with the exported Javadoc too. Both submission and demo are required to get grades. Expected Output (blue - user input) Enter the name of the bank: Spectrum How many account holders do you have: 3 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 2 No accounts to process 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 3 No accounts to display 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 1 1. Chequing 2. Savings Enter the type of account you want to create: 5 Invalid account type... please re-enter 1. Chequing 2. Savings Enter the type of account you want to create: 8 Invalid account type... please re-enter 1. Chequing 2. Savings Enter the type of account you want to create: 1 Enter Account Number: 100123 Enter first name: John Enter last name: Doe Enter email: doe@test.com Enter phone number: 123456 Enter balance: 2300 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 3 ************* *********** Acc Number | ******* 100123 | SPECTRUM BANK Name | John Doe | ******* doe@test.com ************ Email | Phone Number | | 123456 **** Balance 2300.00 *** 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 1 1. Chequing 2. Savings Enter the type of account you want to create: 2 Enter Account Number: 100456 Enter first name: Paul Enter last name: Mathew Enter email: paul@test.com Enter phone number: 456123 Enter balance: 8500 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 1 1. Chequing 2. Savings Enter the type of account you want to create: 1 Enter Account Number: 100789 Enter first name: Peter Enter last name: George Enter email: pet@test.com Enter phone number: 321654 Enter balance: 5520 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 3 ********* Acc Number | 100123 | 100456 | 100789 Name | John Doe | Paul Mathew | Peter George | 1. Read Account 2. Run monthly process. 3. Print all Accounts 4. Exit SPECTRUM BANK Enter your option: 2 1. Read Account 2. Run monthly process. 3. Print all Accounts 4. Exit | Enter your option: 3 Email | Phone Number | 123456 456123 321654 doe@test.com paul@test.com pet@test.com Balance 2300.00 8500.00 5520.00 Acc Number | 100123 100456 100789 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 1 1. Chequing 2. Savings 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 3 Acc Number | ***** 100123 100456 100789 100258 John Doe Paul Mathew Peter George | Enter the type of account you want to create: 2 Enter Account Number: 100258 Enter first name: Sam Enter last name: Thomas Enter email: sam@test.com Enter phone number: 213546 Enter balance: 1000 1. Read Account 2. Run monthly process ********* 3. Print all Accounts 4. Exit Enter your option: 2 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Enter your option: 3 Acc Number I ******* 100123 100456 100789 100258 SPECTRUM BANK Name | John Doe Paul Mathew Peter George Sam Thomas 1. Read Account 2. Run monthly process 3. Print all Accounts 4. Exit Name | SPECTRUM BANK Enter your option: 4 Goodbye... Have a nice day Program written by Anu Thomas. Name | John Doe Paul Mathew | Peter George | Sam Thomas doe@test.com paul@test.com pet@test.com Email | Phone Number | SPECTRUM BANK doe@test.com paul@test.com pet@test.com sam@test.com Email | Phone Number | 123456 456123 | 321654 213546 Email | 123456 456123 | 321654 doe@test.com paul@test.com pet@test.com sam@test.com ******** Phone Number | Balance 2286.50 8528.26 5506.50 Balance 2286.50 8528.26 5506.50 1000.00 ************** Balance 123456 I 2273.00 456123 8556.62 321654 213546 5493.00 1003.33 CST8132 Object-Oriented Programming Lab 5: Banking System II Due Date: Check Brightspace for the due date. Marks: 20 marks (worth 5% of term mark) Demo: Demo your code and output to your lab professor during your own lab hours. Recommended Reading: Chapters 7-11 of Deitel and Deitel, Java How to Program book Exercise For this lab, we are evolving our Banking System of Lab4 so we can make it more robust and a bit richer in its services. The purpose is to apply further the concepts of inheritance, composition, polymorphism, the implementation of interfaces methods and mostly, the usage of Exceptions handling in terms of throwing, catching and defining them. You can freely use any of the classes of Lab4 but you would need to apply the modification to them as per the new requirements described here. Some features are replaced with others while some remain the same. The other goal of this lab is to create POJO classes (Plain Old Java Object) that can be transformed into beans for serialization and persistence. As the first step, you need to create the following classes: Person class: should have personal attributes and required constructor. As you can see, this class now would have only one constructor that takes all Person attributes. No more "no argument" constructor. This requires the readPerson method to be moved either into the driver class or another class. This is to depict real life experience where a Person cannot exist at any time without its attributes i.e., an empty Person object; hence the forbiddance of default/no argument constructor. Account class: abstract class. Should have instance variables to represent the account holder (Person object), the balance, account number and a credit card reference. There should be one constructor that takes a Person object. Therecould be methods to read relevant information and to display the details. With this new version, you need to account for methods as deposit, withdraw, return an account number and a credit card, as well as adding a credit card to an account object. You need to override as well the toString() method so you can display the complete details of an account, including the details of the Person account holder. It is the method that displays the content of a row for each account listed. Chequing class: This is a specific type of account that has a specific fee of $1.5 for every transaction, except if the balance, at the time of the transaction, is greater than $3000; i.e., the fee is not applied if the balance is more than $3000. This fee is stored as a constant. Now the account number is automatically provided when an account is created; i.e., you need to keep track of the account number and increment it for every account created. Also, no two accounts (Chequing or Savings) would have the same account number. This number should be unique. This class takes care of the withdrawal and deposit process, along with the respective usage of the exception classes. Verify the rules pertaining to these types of transactions below. Savings class: This is a specific type of account that has an auto incremented account number for every Savings account created, just like the Chequing one. It holds a fee of $0.5 for every transaction regardless of the balance amount. We will be skipping the monthly update and the interest rate as per Lab4. This class takes care of the withdrawal and deposit process, along with the respective usage of the exception classes. Verify the rules pertaining to these types of transactions below. Bank class: This class should have a name (String) which is the name of the bank, and an ArrayList named accounts, which is an ArrayList of Account objects as instance variables. One constructor is required and it is the one that takes the name of the bank. A bank object should not exist without its name. This constructor sets the name of the Bank and creates the ArrayList of accounts. The main point of the new design is to build on your experience with Lab4 while keeping the MVC design pattern in mind. MVC stands for Model View Controller and the idea behind the abstract classes, the interfaces and their implementation are to hold the "model" where the data resides. The driver class would take care of the menu display and everything that has to do with the Scanner instance; hence, the "view" of the MVC. The "controller" is defined with the methods that interact with the Model and the View. These methods can exist inside Bank or the driver but we need to keep using only one Scanner object, as in Lab4 but to limit it to the driver class, BankTest. In real life, the model is persisted inside a database or some storage, which can be implemented using the file system as well. Credit Card interface: This is a simple interface that has one method, the one that returns the number (16 digits) of a credit card as a String with the card type listed at the end of it; i.e., 5522123456781001 (MC) or 4422123456781002 (V) for example, where MC stands for MasterCard and V for Visa. MasterCard class: This class is an implementation of the Credit Card interface along with carrying out of the credit card unique number. All MasterCard objects, for the purpose of this assignment, need to have this pattern of 5522123456781XXX where XXX are the ones that increment. Our limitation here is that we can only have 999 MasterCards. I guess this is enough for us Visa class: This class is an implementation of the Credit Card interface along with carrying out of the credit card unique number. All Visa objects, for the purpose of this assignment, need to have this pattern of 4422123456781XXX where XXX are the ones that increment. Our limitation here is that we can only have 999 Visa cards. I guess this is enough as well for us. Exceptions All custom exception classes should have Exception as their parent. They all need to only have their respective constructor that takes a String message. The list of these exception classes is: AccountNotFoundException InsufficientFundException TransationAmountCannotBeNegative Bank Test class This is the driver class (test class), which means this class has the main method. Main method . This method read the name of the bank (example: "Spectrum") A Bank object will be created with the name provided Displays all the menus and submenus listed in the sample output below Rules: Handles most of Controller part of the MVC design pattern but shares some as well with Bank class While printing the title, see that it is printed in uppercase even if the user entered it in lowercase or camelCase The methods that you need to override in subclasses should have the same signatures as defined in their parent classes, including any exception thrown As listed below, for every menu/submenu option, the user can enter a non-equivalent value and the program should be to handle it with no exception thrown (InputMismatchException or anything similar); i.e., if the menu options are 1. Or 2., the user can enter any other number and the program needs to recover gracefully by keep prompting and repeating the same menu/submenu until a valid selection is given Also, when a menu or submenu is presented, the user can enter a letter or a word (NOT more than one word) and although the program expects an int or a double for example, with the usage of exceptions, your program needs to handle the situation properly and keeps prompting with the same menu/submenu options until a valid selection is entered . Again, no two accounts can have the same numbers Every account can only hold one credit card. If you re-add a credit card to an account, the new credit card number will override the old one When displaying the details of an account, the last column, which is for the credit card, needs to only appear if there is a credit card number on the account. If no credit card has been added to the account, that last column needs not to appear. Make use of the ternary operator in this case, inside the toString() implementation Please remember to submit code with no typos in it while copying and pasting between different versions Also remember that explaining your code takes precedence over sane code; i.e., if you cannot explain some part of your code, that will affect your marks, despite the fact that your code runs successfully Grading Scheme... in relation to explaining your code during code demo Item Marks Person class Required Account class Chequing class Savings class Bank class Credit Card interface MasterCard class 1 1 1 1 1 1 Visa class AccountNotFoundException Insufficient FundException TransactionAmountCannotBeNegative BankTest class (main method) Comments & Javadoc comments UML Test Plan 1 1 1 1 2 2 2 4 Submission Zip your code, JavaDoc, UML diagram, and test plan in a folder named Lab5.zip and submit it to Brightspace before the due date. Demonstrate your work to your lab professor during your own lab hours. Be ready with the exported Javadoctoo. Both submission and demo are required to get grades. Expected Output (blue - user input) Enter the name of the bank: bmo 1. Create Account 2. Transactions 3. Print all Accounts Apply for Credit Card 4. 5. Exit 2 You need to create an account first 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 3 You need to create an account first 1. Create Account 2. Transactions 3. 4. Apply for Credit Card 5. Exit 4 Print all Accounts You need to create an account first 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit abc Invalid menu option. Try again... 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 1 Let's get to know our new customer. Enter first name: Michel Enter last name: Choueiry Enter email: mic@mic.com Enter phone number: 6135551212 1. Chequing 2. Savings 3 Invalid menu option. Try again... 1. Chequing 2. Savings abc Invalid menu option. Try again... 1. Chequing 2. Savings 1 Acc number | Name | Email | Phone Number | 1 | Michel Choueiry| mic@mic.com | 6135551212 | 1. Create Account 2. Transactions Balance | 0.01 3. Print all Accounts 4. Apply for Credit Card 5. Exit 2 *** Acc number | Name | Email | Phone Number | mic@mic.com | 6135551212| *** 1 | Michel Choueiry| Enter account number: 9 Account number entered 9 is not found. Try again... Enter account number: abc Invalid menu option. Try again... Enter account number: 1 1. Deposit 2. Withdraw 3. Balance 9 Invalid menu option. Try again... 1. Deposit 2. Withdraw 3. Balance abc Invalid menu option. Try again.... 1. Deposit 2. Withdraw 3. Balance 1 Enter the amount to deposit: abc Invalid deposit amount... Enter the amount to deposit: -9 Balance | 0.01 ********* Transaction amount cannot be negative Enter the amount to deposit: 90 Acc number | Name | Email | Phone Number | Balance | ******* mic@mic.com | 6135551212| 88.5 | 1 | Michel Choueiry| 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 2 Acc number | Name | Email | Phone Number | 1 | Michel Choueiry | mic@mic.com | 6135551212| Enter account number: 1 1. Deposit 2. Withdraw 3. Balance 2 Enter the amount to withdraw: abc Enter a valid withdrawal amount... Enter the amount to withdraw: -9 Transaction amount cannot be negative Enter the amount to withdraw: 40 ***** Acc number | Name| Email | Phone Number | Balance | Balance | 88.5| 1 | Michel Choueiry| mic@mic.com | 6135551212 | 47.01 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 2 Acc number | Name| Email | Phone Number | 1 | Michel Choueiry | mic@mic.com | 6135551212 | Enter account number: 1 1. Deposit 2. Withdraw 3. Balance 3 Account balance: 47.0 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 1 Let's get to know our new customer. Enter first name: Shelly Enter last name: Doe Enter email: sdoe@me.com Enter phone number: 6136783456 1. Chequing 2. Savings 2 Acc number | Name| Email | 2 Shelly Doe | sdoe@me.com | Phone Number | 6136783456| Balance | 47.01 Balance | 0.01 ******** ******** ***** 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 2 Acc number | Name | Email | Phone Number | Balance | *** 1 | Michel Choueiry| mic@mic.com | 6135551212| 47.01 2 Shelly Doe | sdoe@me.com| Enter account number: 2 1. Deposit 2. Withdraw 3. Balance 1 Enter the amount to deposit: 100 Acc number | Name | Email | ****** 2 Shelly Doe | sdoe@me.com | 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 3 Acc number | Name| ******** 6136783456 | Phone Number | 6136783456| 0.0 | 1 | Michel Choueiry | mic@mic.com | 6135551212| 2 Shelly Doe | sdoe@me.com | 6136783456 | Balance | 99.5 | BMO BANK Email | Phone Number | Balance | 47.01 99.5 | *** 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 4 Acc number | Name | Email | Phone Number | 1 | Michel Choueiry | mic@mic.com | 6135551212| 2 Shelly Doe sdoe@me.com | 6136783456 | Enter account number: 1 1. MasterCard 2. Visa 1 Acc number | Name | Email | Phone Number | Balance | Credit Card ***** mic@mic.com | 6135551212| 1 | Michel Choueiry| 1. Create Account 2. Transactions 3. Print all Accounts 4. Apply for Credit Card 5. Exit 3 Acc number | BMO BANK Name | 1 | Michel Choueiry| 2 Shelly Doe | sdoe@me.com | 6136783456 | Email | Phone Number | mic@mic.com | 6135551212| Balance | 47.0 | 99.5 | 47.0 | 5522123456781001 (MC) Balance | Credit Card 47.0 | 5522123456781001 (MC) 99.5 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
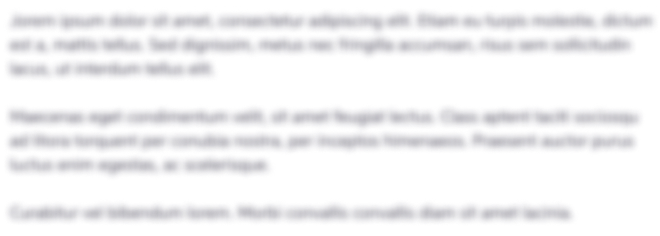
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started